Answered step by step
Verified Expert Solution
Question
1 Approved Answer
We need to only work on minideque.h file. Main.cpp is given and only needs to be changed if needed for testing. Here is the prompt:
We need to only work on minideque.h file. Main.cpp is given and only needs to be changed if needed for testing.
Here is the prompt:
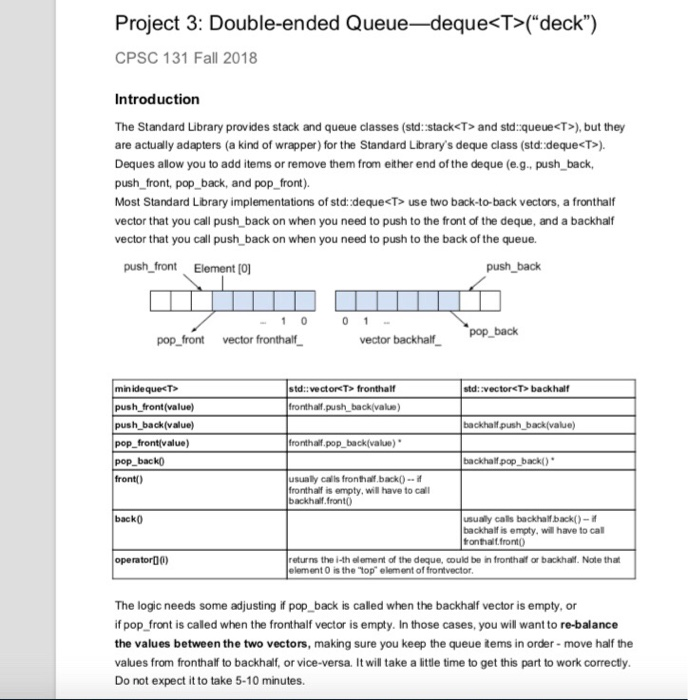
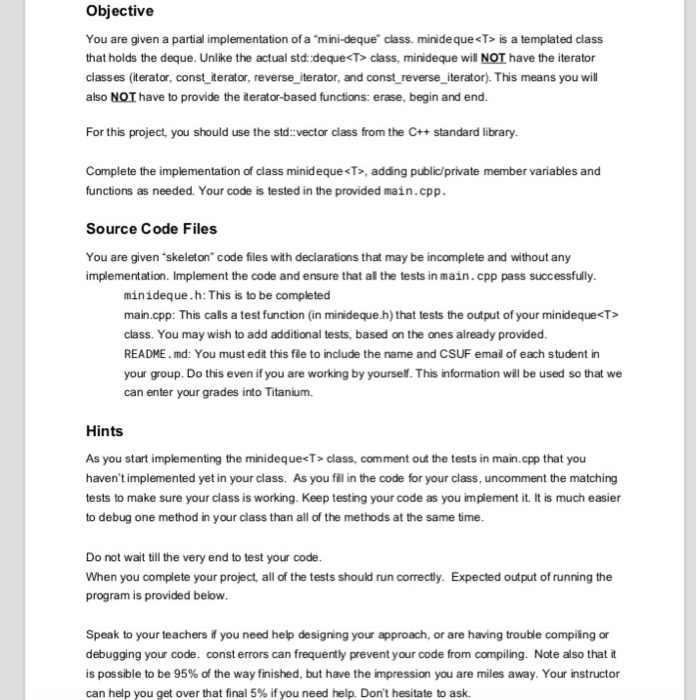
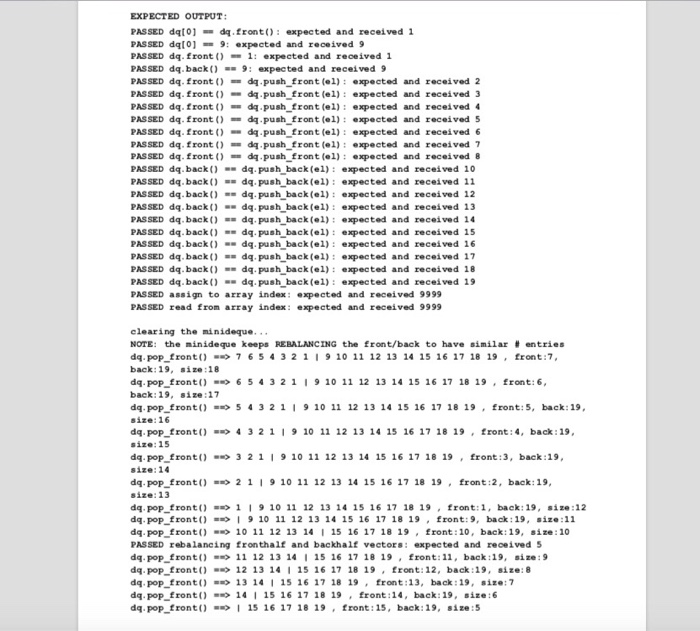
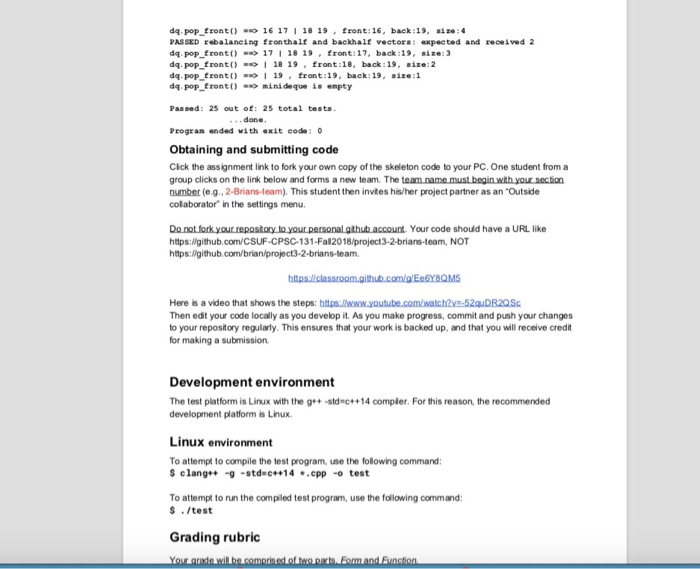
Minideque.h:
//
// minideque.h
// minidequeproject
//
#ifndef minideque_h
#define minideque_h
#include
#include
#include
#include
#include
template
class minideque {
private:
std::vector fronthalf_;
std::vector backhalf_;
public:
minideque() = default; // do not change, C++ defaults are ok
minideque(const minideque& other) = default; // rule of three
minideque& operator=(const minideque& other) = default;
~minideque() = default;
size_t size() const; // TODO
size_t fronthalfsize() const; // TODO
size_t backhalfsize() const; // TODO
bool empty() const; // TODO
{
if(top == -1)
return true;
else
return false;
}
// balance queue across both vectors if pop_front/back is requested on an empty vector
// e.g., minideque has this: | ABCDEFG
// after pop_front BCD | EFG (A discarded)
// symmetric logic for converse case: ABCDEFG | ===> ABC | DEF (G discarded) after pop_back
void pop_front(); // TODO
void pop_back(); // TODO
void push_front(const T& value); // TODO
void push_back(const T& value); // TODO
const T& front() const; // TODO
const T& back() const; // TODO
T& front(); // TODO
T& back(); // TODO
const T& operator[](size_t index) const; // TODO
T& operator[](size_t index); // TODO
void clear(); // TODO
friend std::ostream& operator& dq) { // do not change
if (dq.empty()) { return os
dq.printFronthalf(os);
os
dq.printBackhalf(os);
os
return os;
}
void printFronthalf(std::ostream& os=std::cout) const { // do not change
if (empty()) { std::cout
for (typename std::vector::const_reverse_iterator crit = fronthalf_.crbegin();
crit != fronthalf_.crend(); ++crit) {
os
}
}
void printBackhalf(std::ostream& os=std::cout) const { // do not change
if (empty()) { os
for (typename std::vector::const_iterator cit = backhalf_.cbegin();
cit != backhalf_.cend(); ++cit) {
os
}
}
};
#endif /* minideque_h */
MAIN.CPP:
//
// minideque_project_main.cpp
// minideque_project
//
//
#include
#include "minideque.h"
template
bool testAnswer(const std::string& nameOfTest, const T& received, const T& expected);
size_t testsPassed = 0;
size_t testsFailed = 0;
void test_minideque() {
minideque dq;
dq.push_back(9);
testAnswer("dq[0] == dq.front()", dq[0] == dq.front(), true);
testAnswer("dq[0] == 9", dq[0], 9);
dq.push_front(1);
testAnswer("dq.front() == 1", dq.front(), 1);
testAnswer("dq.back() == 9", dq.back(), 9);
std::vector valuesfront = { 2, 3, 4, 5, 6, 7, 8 };
std::vector valuesback = { 10, 11, 12, 13, 14, 15, 16, 17, 18, 19 };
for (auto el : valuesfront) {
dq.push_front(el);
testAnswer("dq.front() == dq.push_front(el)", dq.front(), el);
}
for (auto el : valuesback) {
dq.push_back(el);
testAnswer("dq.back() == dq.push_back(el)", dq.back(), el);
}
int value = 9999;
dq[0] = value;
testAnswer("assign to array index", dq[0], value);
testAnswer("read from array index", dq[0], value);
std::cout
std::cout
while (!dq.empty()) {
dq.pop_front();
std::cout "
if (!dq.empty()) {
if (dq.front() == 10 || dq.front() == 16) {
testAnswer("rebalancing fronthalf and backhalf vectors",
dq.fronthalfsize(), dq.backhalfsize());
}
}
}
std::cout
}
template
bool testAnswer(const std::string& nameOfTest, const T& received, const T& expected) {
if (received == expected) {
std::cout
++testsPassed;
return true;
}
std::cout Project 3: Double-ended Queue-deque T ("deck") CPSC 131 Fall 2018 Introduction The Standard Library provides stack and queue classes (std:stack and std:queuecT>), but they are actually adapters (a kind of wrapper) for the Standard Library's deque class (std::deque). Deques allow you to add items or remove them from either end of the deque (e.g, push back, push front, pop back, and pop front). Most Standard Library implementations of std::dequecT> use two back-to-back vectors, a fronthalf vector that you call push back on when you need to push to the front of the deque, and a backhalf vector that you call push back on when you need to push to the back of the queue. push front Element 0 push back pop front vector fronthalf vector backhalf pop back minidequecT push front(value) push back(value) pop_front(value) pop backo front) std:: vectoT> fronthalf std: vectorcT> backhalf push back(value) backhalf push back(value) pop back(value) backhalf pop back) y cals fronthalf back0- s empty, wil have to call backhalf.front0 back0 usualy cals backhalf back)- backhalf is empty, will have to cal fonthalt.front operator00) returns the i-th element of the deque, ould be in fronthalf or backhaf. Note that tO is the top element of frontvector The logic needs some adjusting if pop back is called when the backhalf vector is empty, or if pop front is called when the fronthalf vector is empty. In those cases, you will want to re-balance the values between the two vectors, making sure you keep the queue tems in order- move half the values from fronthalf to backhalf, or vice-versa. It will take a little time to get this part to work correctly Do not expect it to take 5-10 minutes
++testsFailed;
return false;
}
int main(int argc, const char * argv[]) {
test_minideque();
std::cout
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
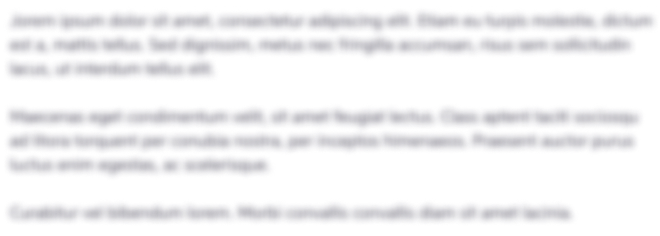
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started