Question
We want to design a class to encapsulate a FEN chess position and print out some information. We also want to make sure the FEN
We want to design a class to encapsulate a FEN chess position and print out some information. We also want to make sure the FEN is correct.
1. Create an InvalidFenException class, similar to the example from class.
2. Copy and paste the ChessGameClient.
3. Create a ChessGame class using the instructions below.
4. Have fun!
* ChessGame
* Fields
* String pieces
* Character activeColor
* String castlingOptions
* String enPassant
* Methods
* Constructor
* _ChessGame_
* Takes in a single argument, a String containing the entire FEN.
* This constructor may throw an InvalidFenException.
* Use the Scanner class to parse the FEN by spaces.
* Throw an InvalidFenException for missing data.
* If the activeColor is not a `b` or `w`, throw an InvalidFenException.
* Using a regular expression to check if the castling options are valid, if not, throw an InvalidFenException.
* Use a regular expression to check if the en passant is valid, if not, throw an InvalidFenException.
* _PrintBoard_
* Void method that prints out some information.
* Use the Scanner class to parse the pieces by slashes.
* Use the algorithm below.
* Print if it is white's move or black's move.
* Print the castling options, or not available if there are none.
* Print if en passant is available or not.
```
Parse the pieces by slashes.
while there is a next field
for i = 0; i < length; i++
get the character in the field at index i
if that character is a digit
convert it to an int
Use a for loop to print that many .'s
otherwise
print the character and a space
```
```java
public class ChessGameClient {
public static void main(String[] args) {
String[] boards = {
"2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w -",
"2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w",
"2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 k - -",
"2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w KQk- -",
"2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w - k3",
"2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w - a9",
"2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w - -",
"r2q1rk1/5pbp/2n1p1p1/p7/Pp1PpB2/1NP4P/1P3PP1/R2Q1RK1 b - -",
"rnbqkbnr/pppppppp/8/8/3P4/8/PPP1PPPP/RNBQKBNR b KQkq d3"};
System.out.println("-----------------------------");
for (String b : boards) {
System.out.println(b);
try {
ChessGame cg = new ChessGame(b);
cg.printBoard();
} catch (InvalidFenException ife) {
System.err.println(ife.getMessage());
}
System.out.println("-----------------------------");
}
}
}
```
Desired output:
```
-----------------------------
2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w -
Error, missing fields.
-----------------------------
2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w
Error, missing fields.
-----------------------------
2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 k - -
Error, active color is invalid.
-----------------------------
2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w KQk- -
Error, castling is invalid.
-----------------------------
2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w - k3
Error, en passant is invalid.
-----------------------------
2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w - a9
Error, en passant is invalid.
-----------------------------
2r1brk1/1p5p/p1nQ3P/2N2pq1/3P4/2P2p2/P4PP1/2R1R1K1 w - -
. . r . b r k .
. p . . . . . p
p . n Q . . . P
. . N . . p q .
. . . P . . . .
. . P . . p . .
P . . . . P P .
. . R . R . K .
It is white's move.
It is not possible to castle.
En passant is not available.
-----------------------------
r2q1rk1/5pbp/2n1p1p1/p7/Pp1PpB2/1NP4P/1P3PP1/R2Q1RK1 b - -
r . . q . r k .
. . . . . p b p
. . n . p . p .
p . . . . . . .
P p . P p B . .
. N P . . . . P
. P . . . P P .
R . . Q . R K .
It is black's move.
It is not possible to castle.
En passant is not available.
-----------------------------
rnbqkbnr/pppppppp/8/8/3P4/8/PPP1PPPP/RNBQKBNR b KQkq d3
r n b q k b n r
p p p p p p p p
. . . . . . . .
. . . . . . . .
. . . P . . . .
. . . . . . . .
P P P . P P P P
R N B Q K B N R
It is black's move.
The castling options are: KQkq
En passant is available.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
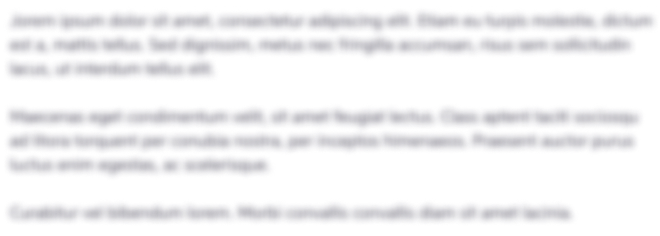
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started