Question
We want to write a Java program to implement classes and methods for geometrical shapes using inheritance and polymorphism. Following is the skeleton and description
We want to write a Java program to implement classes and methods for geometrical shapes using inheritance and polymorphism.
Following is the skeleton and description of the classes that you need to complete.
class Point { public Point(double x, double y);
Creates an instance of point using its x and y coordinates. public double getX();
Returns the x coordinate of the point. public double getY();
Returns the y coordinate of the point. public double getDistance(Point point);
Returns the Euclidean distance of the point from a given point.
}
abstract class Shape { public abstract double getArea();
It calculates and returns the area of a shape. Note that the method is abstract and all concrete subclasses of shape should implement it.
public abstract double getPerimeter(); It calculates and returns the perimeter of a shape. Again, this method is abstract and all concrete subclasses of shape should implement it. }
interface Symmetric { Point getPointOfSymmetry();
Returns an object of type Point which is the point of symmetry (or center of symmetry) of a shape. This method is abstract and each concrete class that implements the interface should implement it.
}
class Triangle extends Shape { public Triangle(Point firstPoint, Point secondPoint, Point
thirdPoint);
Creates a triangle object using 3 points.
public Point getFirstPoint(); Returns the first point of the triangle which is the first point given in the constructor.
public Point getSecondPoint(); Returns the second point of the triangle which is the second point given in the constructor.
public Point getThirdPoint(); Returns the third point of the triangle which is the third point given in the constructor.
} class Rectangle extends Shape {
public Rectangle(Point topLeftPoint, double length, double width); Creates a rectangle using the top-left corner of the rectangle and its length and width.
public Point getTopLeftPoint(); Returns the top-left corner of the rectangle.
public double getLength(); Returns the length of the rectangle.
public double getWidth(); Returns the width of the rectangle.
}
class Trapezoid extends Shape { public Trapezoid(Point topLeftPoint, Point bottomLeftPoint, double
topSide, double bottomSide); Creates a trapezoid using top-left and bottom-left corners as well as the length of the top and bottom sides.
public Point getTopLeftPoint(); Returns the top-left corner of the trapezoid.
public Point getBottomLeftPoint(); Returns the bottom-left corner of the trapezoid.
public double getTopSide(); Returns the length of the top side of the trapezoid.
public double getBottomSide(); Returns the length of the bottom side of the trapezoid.
}
class Circle extends Shape implements Symmetric { public Circle(Point center, double radius);
Creates a circle using its center and radius.
public Point getCenter(); Returns center of the circle.
public double getRadius(); Returns radius of the circle.
} class EquilateralTriangle extends Triangle implements Symmetric {
public EquilateralTriangle(Point topPoint, double side); Creates an equilateral triangle using the top point and its side length. Note that the other two corners (points) have the same y value. Also, getFirstPoint(), getSecondPoint(), and getThirdPoint() methods should return the top, bottom-left, and bottom-right corners, respectively.
public Point getTopPoint(); Returns the top corner of the equilateral triangle.
public double getSide(); Returns the side length of the equilateral triangle.
} class Square extends Rectangle implements Symmetric {
public Square(Point topLeft, double side); Creates a square using the top-left corner and its side length.
public double getSide(); Returns the side length of the square.
} public class Plane {
public Plane(); Creates an empty plane.
public Shape[] getShape(); Returns an array of shape objects on the plane. The order of objects is not important.
Public void addShape(Shape shape); Takes a shape object and adds it to the array of shapes on the plane. (Remember addPoint() method in Assignment 3?)
public double getSumOfAreas(); Calculates the sum of the areas of all the shapes on the plane. If there is any overlapped area, it will be counted twice.
public double getSumOfPerimeters(); Calculates the sum of the perimeters of all the shapes on the plane.
public Point getCenterOfPointOfSymmetries(); Returns the center of all points of symmetry of symmetric shapes. Center of symmetry of a set of points is a point with x coordinate being the average of x coordinate of the points, and y coordinate
being the average of y coordinates of the points. If there is no symmetric shapes on the plane, returns null. You might need to read about instanceof operator to complete this method (Suggested link).
You can implement other methods in the above-mentioned classes. Except from the methods listed above, all of the extra methods and all class fields should be defined as private. You have to use concepts from inheritance and polymorphism whenever applicable. Your mark for this question is not solely based on the correct output, but it is also based on the correct usage of inheritance and polymorphism.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
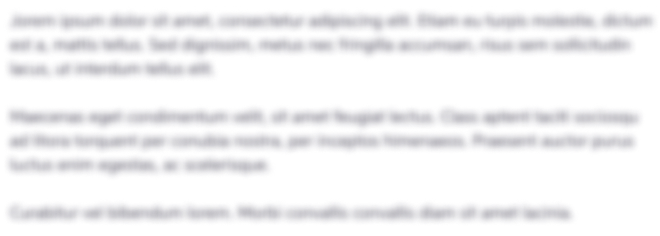
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started