Question
We will be working on the following files. WE will be working in the library sterling. The library consists of these two files: src/sterling.cpp src/sterling.h
We will be working on the following files.
- WE will be working in the library sterling. The library consists of these two files:
- src/sterling.cpp
- src/sterling.h
- Yur "driver/test" file is be src/main.cpp.
Please DO NOT MODIFY any other files. Modifying any other file may result in the tests not passing.
Part I
Create a class library with the following data items:
class Sterling { private: long pounds_; // pounds int shills_; // shillings: 20 per pound int pence_; // pence: 12 per shilling };
Your class should be able to support the following constructors:
Sterling(); // default constructor Sterling(long pounds, int shills, int pence); // 3-arg constructor
The following methods for your getters to interact with your class data members:
long get_pounds() const; int get_shills() const; int get_pence() const;
The following methods for your setters to interact with your class data members:
void set_pounds(long pounds); void set_shills(int shillings); void set_pence(int pence);
There are 12 pence in a shilling. There are 20 shillings in a pound. In your setters make sure that any pence over 12 are added to shillings and any shillings over 20 are added to pounds.
For example, if the set_shills is called with the value 28, shills_ would be set to 8, and 1 would be added to pounds_.
Another example, if set_pence is called with the value 28, pence_ would be set to 4, and 2 would be added to shills_ (if shills gets to 20 or more remember to move some to pounds).
Pounds can be any integer value.
Sample output Part I
Add code to main to test your code. Sample Output
Constructor with no arguments gives you: Pounds: 0 Shilling: 0 pence: 0 Constructor with three arguments (12, 18, 9) gives you: Pounds: 12 Shilling: 18 pence: 9 # Note: you have to convert extra pence to shillings and extra shillings to pounds Constructor with three arguments (12, 48, 29) gives you: Pounds: 14 Shilling: 10 pence: 5 This can be done by calling the setters.
Part II
Add the following data item:
private: // ... std::string old_system_; // 13.18.1
This data member stores the amount in a string in the format: pounds.shillings.pence, For example, 28.16.3
Add another constructor:
Sterling(const std::string &old_system); // 1-arg constructor old_system
This constructor is to set the old_system_ data member, as well as setting the other three data members: pound_, shills_, and pence_. To make this simpler, we will expect the value sent is in the proper format. There are two .s, the value of shillings is 0-19, the value of pence is 0-11, and there are no non-numeric characters.
Since this is a string it will need to be split to get the value of pounds, shillings, and pence.
Hint 1: Check the
Hint 2: You can convert strings to integers using the stoi() function.
You can create an auxiliary method (optional) to help you split the string into the three integers. For example, you can create a method that will help you split the input string.
(optional) void Split(std::string stringToBeSplit);
The Split function takes one argument, the string to be parsed.
Once you have split the string, update the private data members with the information extracted.
Add the following method for your getter to interact with your class data members:
const std::string& get_old_system() const; // gets "7.10.6"
Sample output Part II
Add code to main to test your code. Sample Output
Constructor with one arguments ("12.8.9") gives you: Pounds: 12 Shilling: 8 pence: 9 or using get_old_system() method: 12.8.9
Part III
Add another constructor that takes the input in decimal pound format. This is the modern format.
Sterling(double decimal_pounds); // 1-arg constructor dec pounds
For this, you will need to do the conversions from the decimal representation to extract the pound_, shills_, and pence_ data members.
The whole part of the number is pounds. You can get this using the floor function or typecasting to an int. The floor function is available in the
pounds = floor(decimal_pounds)
The fractional part of the number will be the shillings and pence. To get the fractional part, you can use subtraction.
fractional_part = decimal_pounds - pounds;
Be careful here with types. The decimal_pounds, fractional_part, and decimal_shillings are doubles. The pounds, shillings, and pence are integers.
You can get the decimal_shillings by multiplying the fractional part times 20. Then the shillings are the whole part of this number. Use floor to get this.
decimal_shillings = fractional_part * 20; shillings = floor(decimal_shillings);
Now what's left is the pence. You go through the same process here. You get the part that is left by subtracting, multiply by 12, and take the floor* of that to get pence.
// subtraction pence = floor((decimal_shillings - shillings) * 12);
Once you have computed the pounds, shillings, and pence, use the set methods to set the data members.
*For more accuracy you would use rounding here but that varies between operating systems so it doesn't work well with the unit tests and students using different operating systems.
Sample output Part III
Add code to main to test your code. Sample Output
Constructor with one arguments (45.67) gives you: Pounds: 45 Shilling: 13 pence: 4
Part IV
Next, we will add a friend function to overload the stream output << operator.
friend std::ostream& operator<<(std::ostream &os, const Sterling &sterling);
Your method is to print a friendly output for the Sterling object. Match the following with spaces as shown.
pounds: 31 shills: 11 pence: 4
Sample output Part IV
Add code to test your operator in main
cout << "Constructor with one arguments (45.67) and using << operator " << endl; Sterling s6(45.67); cout << s6;
Output:
Constructor with one arguments (45.67) and using << operator pounds: 45 shills: 13 pence: 4
Part V
We have been testing our "getters" a lot, but in this part, you will test your "setters" to make sure you can update information in your class.
void set_pound(long pounds); void set_shills(int shillings); void set_pence(int pence); void set_old_system(const std::string &sterling); // user types "7.10.6"
Sample output Part V
Add code to main to test your code. Sample Output
Constructor with one arguments (42.27) pounds: 42 shills: 5 pence: 5 Update pounds to 23 pounds: 23 shills: 5 pence: 5 Constructor with one arguments (42.27) pounds: 42 shills: 5 pence: 5 Update shills to 67, and adjust numbers pounds: 45 shills: 7 pence: 5 Constructor with one arguments (42.27) pounds: 42 shills: 5 pence: 5 Update pence to 31, and adjust numbers pounds: 42 shills: 7 pence: 7
Part VI
Next, we will develop the following comparison operators:
bool operator == (const Sterling &rhs) const; bool operator != (const Sterling &rhs) const;
Your operators are to compare the pounds_, shills_, and pence_ data members. If they are the same, return true for the == and false for the != operator.
Sample output Part VI
Add code to main to test your code. Sample Output
# Testing == operator Create two objects: pounds: 9 shills: 4 pence: 7 pounds: 9 shills: 4 pence: 7 object1 and object2 are equal Create two objects: pounds: 9 shills: 4 pence: 7 pounds: 12 shills: 4 pence: 2 object1 and object2 are not equal # Testing != operator Create two objects: pounds: 67 shills: 17 pence: 7 pounds: 33 shills: 2 pence: 2 object1 and object2 are not equal Create two objects: pounds: 33 shills: 2 pence: 2 pounds: 33 shills: 2 pence: 2 object1 and object2 are equal
Part VII
Next, add a method to convert back to decimal pound system. Your method will use the pounds_, shills_, and pence_ data members to compute this.
float decimal_pound();
This method will return the decimal pound form of the Sterling. For this you need to use the following formula:
pounds + shills/20.0 + pence/240.0
Sample output Part VII
Add code to main to test your code. Sample Output
Constructor with one arguments (12.27) pounds_: 12 shills_: 5 pence_: 5 Using decimal_pound() operator 12.2708
Part VIII
Finally, add some arithmetic operator overloads to add and subtract to Sterling objects
Sterling operator + (Sterling s2) const; // sterling + sterling Sterling operator - (Sterling s2) const; // sterling - sterling // // or with friend function // friend Sterling operator + (Sterling const &s1, Sterling const &s2); // sterling + sterling friend Sterling operator - (Sterling const &s1, Sterling const &s2); // sterling + sterling
Hint: Remember to use static_cast
Sample output Part VIII
// You may use this code to test your operators // Testing + operator cout << " Create two objects:" << endl; Sterling s13(33.15); Sterling s14(6.21); cout << s13 << endl; cout << s14 << endl; Sterling s15 = s13 + s14; cout << "The sum of obj1 + obj2 = " << s15.decimal_pound() << endl; // Testing - operator cout << endl << endl; cout << s13 << endl; cout << s14 << endl; Sterling s16 = s13 - s14; cout << "The difference of obj1 - obj2 = " << s16.decimal_pound() << endl;
Sample Output
# Testing + operator Create two objects: pounds_: 33 shills_: 2 pence_: 12 pounds_: 6 shills_: 4 pence_: 2 The sum of obj1 + obj2 = 39.3083 # Testing - operator Create two objects: pounds_: 33 shills_: 2 pence_: 12 pounds_: 6 shills_: 4 pence_: 2 The difference of obj1 - obj2 = 26.9417
Optional: If you want one more puzzle to solve, in the operator - method handle the case where the subtraction leaves a negative number in either shillings or pence. When this happens you need to move value from pounds or shillings.
You can use the following to test it.
cout << " The difference of obj1 - obj2 = " << s16 << endl; // or cout << " Test Optional Subtraction:" << endl; Sterling s17(27.26); Sterling s18(12.78); cout << s17 << endl; cout << s18 << endl; Sterling s19 = s17 - s18; cout << "The difference of obj3 - obj4 = " << s19 << endl;
Sample Output
The difference of obj1 - obj2 = pounds_: 26 shills_: 18 pence_: 9 Test Optional Subtraction: pounds_: 27 shills_: 5 pence_: 2 pounds_: 12 shills_: 15 pence_: 7 The difference of obj3 - obj4 = pounds_: 14 shills_: 9 pence_: 7
Step by Step Solution
There are 3 Steps involved in it
Step: 1
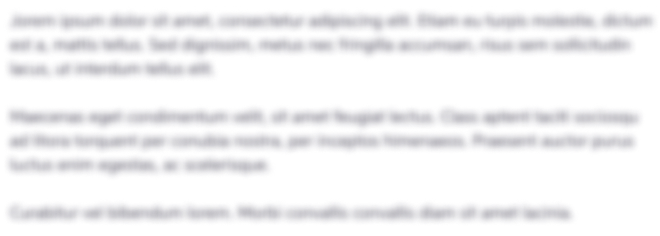
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started