Answered step by step
Verified Expert Solution
Question
1 Approved Answer
We will continue working on Python. Using a Google Colab notebook write the python statement to define a Pizza class. The test harness is provided
- We will continue working on Python. Using a Google Colab notebook write the python statement to define a Pizza class. The test harness is provided to you by the instructor as well as the resulting output given.
- Topics covered:
- List
- Dictionary
- Class
- Constructor
- Property
- Methods
- Exception
- How to do this assignment.
- From the test harness and the given output, try to deduce the definition of the Pizza class. Code this class in a colab notebook and copy the test harness to a cell below. Execute the notebook and ensure that the output matches EXACTLY with the output on the following page.
- You must use Python f-strings for your output.
- Instruction.
- Do not modify the test harness.
- Documentation.
- Please enter your name and the current date somewhere at the top of your code.
- How to submit this assignment.
- Make the notebook shareable and submit the link to the course assessment folder.
- See the folder documentation for due date.
- See the course documentation for policy on deadlines.
Specifications for the Pizza class.- Please implement the Pizza class using the following instructions.
- Class attributes
- Remember that class members do not have a "self" prefix and are accessed with the class name and the dot operator.
- Valid sizes for pizza are contained in a collection at the class level.
You get to decide on the type of collection to store the sizes below.
Valid sizes are small, medium, large and x-large. - Another class level collection having the prices for each valid size.
You get to decide on the type of collection to store the prices below.
Prices are small: $6.49, medium: $8.49, large: $10.49, x-large: $13.49. - Constructor
- Instance methods have an implicit first argument "self".
- A constructor that takes a default size of medium and topping of a list of cheese that does the following:
- Set the first argument size to the instance attribute size. Size must be verified. This can be done by setting the size property.
- Creates an instance attribute a list with the second argument. If the second argument is missing, a single cheese topping is inserted in the list.
- Instance methods
- Instance methods have an implicit first argument "self".
- A method that takes an argument of type list of strings. It adds topping to the list of pizza toppings.
- Implement the __str__() method to return a formatted string. Examine the output from the test harness for clues on how to implement this method.
- Instance Properties
- Instance properties have an implicit first argument "self".
- A property that returns the price of the pizza.
- Price is based on the size as well as the number of toppings.
See spec#2 for cost based on size. Each topping cost an additional $0.50 each.
- Price is based on the size as well as the number of toppings.
- A property that returns the size of the pizza.
- A property that sets the size of the pizza.
- Size must be verified. A ValueError exception is raised if the size is invalid.
- Instance Attributes
- All of the following Instance attributes are initialized in the constructor from the values of the argument. Notice the __ prefix to make is private.
- __size is a str that stores the size of this object. This is initialized in the constructor. It is mutated by the property in #8. It is returned in #7. It is used in #6 to calculate the cost of the pizza.
- __toppings a list of string that represents the toppings for this object. This is initialized in the constructor. It is mutated in the add() method and is used in #6 to calculate the cost of the pizza.
- The test harness.
- You may not change the test harness.
- print(f'Creating a default pizza')
- p = Pizza()
- print(p)
- toppings = 'cheese olive'.split()
- print(f'Adding topping: {toppings}')
- p.add(toppings=toppings)
- print(p)
- print(f'Creating a new pizza')
- p = Pizza('large', 'cheese pepper'.split())
- print(p)
- toppings = ['pineapple', 'mushroom']
- print(f'Adding topping: {toppings}')
- p.add(toppings)
- print(p)
- size = 'x-large'
- p.size = size
- print(f'Changing order size to {size}')
- print(p)
- size = 'gigantic'
- print(f'Changing order size to {size}')
- try:
- p.size = size
- except ValueError as err:
- print(err)
- The resulting output
- Creating a default pizza
- medium pizza with ['cheese'] for $8.99
- Adding topping: ['cheese', 'olive']
- medium pizza with ['cheese', 'cheese', 'olive'] for $9.99
- Creating a new pizza
- large pizza with ['cheese', 'pepper'] for $11.49
- Adding topping: ['pineapple']
- large pizza with ['cheese', 'pepper', 'pineapple', 'mushroom'] for $12.49
- Changing order size to x-large
- x-large pizza with ['cheese', 'pepper', 'pineapple', 'mushroom'] for $15.99
- Changing the order size to gigantic
- ERROR: Gigantic is not a valid size for a pizza
Step by Step Solution
★★★★★
3.52 Rating (162 Votes )
There are 3 Steps involved in it
Step: 1
python class Pizza Class attributes validsizes small medium large xlarge sizeprices small 649 medium ...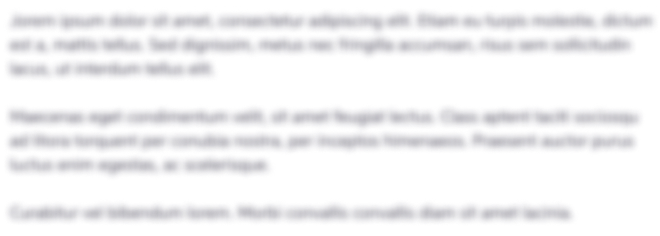
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started