Question
We would like to modify the ADT Stack interface to have the following new methods: 1) peek2(): T peeks at the entry beneath the top
We would like to modify the ADT Stack interface to have the following new methods:
1)peek2(): T
peeks at the entry beneath the top entry, ie 2nd entry in stack. If the stack has more than 1 entry then peek2 returns the 2nd element in the stack (without removing it) otherwise it returns null.
2)wasta1PopLast(): T
pops the last element in the stack if stack is not empty otherwise it returns null.
3)revenge():
removes the first element in the stack to the last element in the stack.
a) Update the StackInterface accordingly,
b) Update the implementation of the LinkedStackInterface
c) Create a new class Student with the following
* Data: studentName and studentId
* Methods:
i.Constructor with 2 parameters, one for student name and one for student id;
ii.Getter methods getStudentName & getstudentId
iii.toString method
d) Write a test case to test peek2, wasta1PopLast, and revenge(); Create several new students, add them to the stack, then
* Call method testPeek2() and see if it returns the correct result
* Call method wasta1PopLast and see if returns the correct result
* Call method revenge and see if it works correctly
-------------------------------------------------------
/** An interface for the ADT stack. @author Frank M. Carrano @author Timothy M. Henry @version 4.0 */ public interface StackInterface
-------------------------------------------------------
import java.util.EmptyStackException; /** A class of stacks whose entries are stored in a chain of nodes. @author Frank M. Carrano @author Timothy M. Henry @version 4.0 */ public final class LinkedStack
public T peek() { if (isEmpty()) throw new EmptyStackException(); else return topNode.getData(); } // end peek
public T pop() { T top = peek(); // Might throw EmptyStackException assert (topNode != null); topNode = topNode.getNextNode();
return top; } // end pop
/* // Question 1, Chapter 6: Does not call peek public T pop() { if (isEmpty()) throw new EmptyStackException(); else { assert (topNode != null); top = topNode.getData(); topNode = topNode.getNextNode(); } // end if return top; } // end pop */
public boolean isEmpty() { return topNode == null; } // end isEmpty public void clear() { topNode = null; // Causes deallocation of nodes in the chain } // end clear
private class Node { private T data; // Entry in stack private Node next; // Link to next node
private Node(T dataPortion) { this(dataPortion, null); } // end constructor
private Node(T dataPortion, Node linkPortion) { data = dataPortion; next = linkPortion; } // end constructor
private T getData() { return data; } // end getData
private void setData(T newData) { data = newData; } // end setData
private Node getNextNode() { return next; } // end getNextNode
private void setNextNode(Node nextNode) { next = nextNode; } // end setNextNode } // end Node } // end LinkedStack
Step by Step Solution
There are 3 Steps involved in it
Step: 1
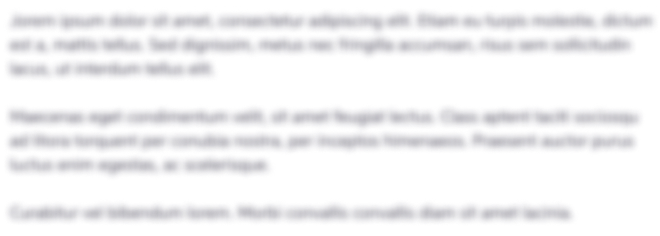
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started