Question
Web Programming with Notepad++ Programming Assignment JavaScript Static Multiplication Table Overview The purpose of this assignment is to demonstrate competency in translating a static HTML
Web Programming with Notepad++ Programming Assignment JavaScript Static Multiplication Table
Overview
The purpose of this assignment is to demonstrate competency in translating a static HTML mark-up layout containing a table and render the table in a static manner with JavaScript. The student will re-enforce skills learned in relation to mark-up, CSS, and JavaScript while completing this assignment.
Assignment
Recall the W3 Schools website JavaScript example on the following topics from last assignment for this problem (http://www.w3schools.com/js/default.asp):
JS Home
JS Introduction
JS Where To
JS Output
JS Syntax
JS Statements
JS Comments
JS Variables
JS Operators
JS Arithmetic
JS Assignment
Additionally, review the W3 Schools website JavaScript examples on the following topics:
JS Data Types
JS Functions
JS Where To
JS Objects
JS Scope
JS Strings
JS String Methods
JS Numbers
JS Number Methods
JS Math
Once you have a working understanding of the concepts reviewed in these examples, take your multiplication table from Assignment #03 with the well-separated style-sheets. Then, write a JavaScript that draws the table instead of including the mark-up within the HTML document itself (the HTML file simple contains a call to the JS that is responsible for writing the mark-up that produces the multiplication table. The HTML, CSS, and JS should all be in different files and together in a zipped folder for submission. When the HTML file is opened, connectivity to the script and associated styles should occur naturally as long as the files are together in the folder.
The table does not have to change shape, although considering the way to make this solution scale for a custom-dimension table request would be wise since the next assignment will call for this. For this assignment though, the emphasis should be on the connectivity between the different files that go into drawing the webpage and blindly producing the mark-up to draw the table with JS. Some of the topics you have been asked to review are not necessarily needed to complete this assignment, but since they will be topics of great emphasis in upcoming assignments, exposure to these ideas now and an attempt to incorporate some of them into your assignment submission is an excellent idea and strongly encouraged.
Please include a separate Javascript file. Here's what I have so far:
My Multiplication Table.html
My Multiplication Table. Enjoy!!!
1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 |
2 | 4 | 6 | 8 | 10 | 12 | 14 | 16 | 18 | 20 | 22 | 24 |
3 | 6 | 9 | 12 | 15 | 18 | 21 | 24 | 27 | 30 | 33 | 36 |
4 | 8 | 12 | 16 | 20 | 24 | 28 | 32 | 36 | 40 | 44 | 48 |
5 | 10 | 15 | 20 | 25 | 30 | 35 | 40 | 45 | 50 | 55 | 60 |
6 | 12 | 18 | 24 | 30 | 36 | 42 | 48 | 54 | 60 | 66 | 72 |
7 | 14 | 21 | 28 | 35 | 42 | 49 | 56 | 63 | 70 | 77 | 84 |
8 | 16 | 24 | 32 | 40 | 48 | 56 | 64 | 72 | 80 | 88 | 96 |
9 | 18 | 27 | 36 | 45 | 54 | 63 | 72 | 81 | 90 | 99 | 108 |
10 | 20 | 30 | 40 | 50 | 60 | 70 | 80 | 90 | 100 | 110 | 120 |
11 | 22 | 33 | 44 | 55 | 66 | 77 | 88 | 99 | 110 | 121 | 132 |
12 | 24 | 36 | 48 | 60 | 72 | 84 | 96 | 108 | 120 | 132 | 144 |
My Multiplication Table styles.css
body { line-height: 1; margin: 50px; background-color:DeepSkyBlue; font-family:Agency FB; } div { background: #9abab5; border-radius: 15px; box-sizing: border-box; padding: 15px; width: 310px; } header { overflow: clear; position: relative; } h2 { font-family: sans-serif; font-size: 20px; font-weight: 700; margin: 0 0 10px; text-align: center; } table { border-color: black; background: #fffe; border-collapse: collapse; font-size: 20px; width: 50%; } td { border: 1px solid #ccc; border-color: black; color: #444; line-height: 22px; text-align: center; } tr { color: #222; font-weight: 700; } .selected { background: #f0951d; border: 0; box-shadow: 0 2px 6px rgba(0, 0, 0, .5) inset; }
1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 |
2 | 4 | 6 | 8 | 10 | 12 | 14 | 16 | 18 | 20 | 22 | 24 |
3 | 6 | 9 | 12 | 15 | 18 | 21 | 24 | 27 | 30 | 33 | 36 |
4 | 8 | 12 | 16 | 20 | 24 | 28 | 32 | 36 | 40 | 44 | 48 |
5 | 10 | 15 | 20 | 25 | 30 | 35 | 40 | 45 | 50 | 55 | 60 |
6 | 12 | 18 | 24 | 30 | 36 | 42 | 48 | 54 | 60 | 66 | 72 |
7 | 14 | 21 | 28 | 35 | 42 | 49 | 56 | 63 | 70 | 77 | 84 |
8 | 16 | 24 | 32 | 40 | 48 | 56 | 64 | 72 | 80 | 88 | 96 |
9 | 18 | 27 | 36 | 45 | 54 | 63 | 72 | 81 | 90 | 99 | 108 |
10 | 20 | 30 | 40 | 50 | 60 | 70 | 80 | 90 | 100 | 110 | 120 |
11 | 22 | 33 | 44 | 55 | 66 | 77 | 88 | 99 | 110 | 121 | 132 |
12 | 24 | 36 | 48 | 60 | 72 | 84 | 96 | 108 | 120 | 132 | 144 |
My Multiplication Table styles.css
body { line-height: 1; margin: 50px; background-color:DeepSkyBlue; font-family:Agency FB; } div { background: #9abab5; border-radius: 15px; box-sizing: border-box; padding: 15px; width: 310px; } header { overflow: clear; position: relative; } h2 { font-family: sans-serif; font-size: 20px; font-weight: 700; margin: 0 0 10px; text-align: center; } table { border-color: black; background: #fffe; border-collapse: collapse; font-size: 20px; width: 50%; } td { border: 1px solid #ccc; border-color: black; color: #444; line-height: 22px; text-align: center; } tr { color: #222; font-weight: 700; } .selected { background: #f0951d; border: 0; box-shadow: 0 2px 6px rgba(0, 0, 0, .5) inset; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
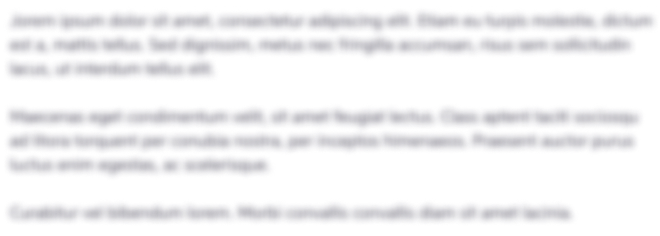
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started