Question
What I was required to do is based on the 30 instructions that were listed in the MIPS Green Card page, I'm was required to
What I was required to do is based on the 30 instructions that were listed in the MIPS Green Card page, I'm was required to implement the 30 instructions that were listed in the MIPS Green Card page by writing a code and run it on the MIPS Mars Simulator. This is the code I wrote.
Code:
# Add # add the values in $s4 and $s5 and store the result in $s3 li $s4, 16 # $s4 should contain the value 0x00000010 li $s5, -2 # $s5 should contain the value 0xFFFFFFFE add $s3, $s4, $s5 # $s3=$19 should contain the value 0xE.
# Add Immediate li $s4, 3 addi $s3, $s4, 10 # add the immediate value 10 to the value in $s3 and store the result in $s3 # $s3 should now contain the value 0xD.
# Add Unsigned li $s4, 5 li $s5, 7 addu $s3, $s4, $s5 # add the unsigned values in $s4 and $s5 and store the result in $s3 # $s3 should contain the value 0xC.
# Add Immediate Unsigned li $s4, 5 addiu $s3, $s4, 10 # add the immediate unsigned value 10 to the value in $s4 and store the result in $s3 # $s3 should now contain 15 = 0xF
# And li $s4, 0xC # $s4 should contain the bits 1100 li $s5, 0x5 # $s5 should contain the bits 0101 and $s3, $s4, $s5 # perform a bitwise AND operation between $s4 and $s5 and store the result in $s3 # The $s3 register should contain 0100 = 0x4
# And Immediate li $s4, 241 # $s4 should contain the value 0xF0 1111 0001 andi $s3, $s4, 19 # perform a bitwise AND operation between $s4 and the immediate value 0x13 and store the result in $s3 # The value in $s3 should contain 17 which is 0x11
# Branch On Equal li $t0, 5 li $t1, 5 beq $t0, $t1, L1 # branch to L1 if $t0 == $t1 add $t0, $t0, $t1 L1: # $t0 should still be 5 not 10.
# Branch On Not Equal li $t0, 5 li $t1, 5 bne $t0, $0, L2 # branch to L2 if $t0 = 1 add $t0, $t0, $t1 L2: # $t0 should be 10 not 5.
# Jump li $t0, 3 j target # jump to the instruction at label "target" li $t0, -99 target: # $t0 should be 3.
# Jump And Link
li $t0, 21 # jump to the instruction at label "method" and store the return address in $ra jal method # $t0= $8 should contain 0x42 j Ljr method: li $t0, 66 jr $ra
Ljr: # Jump Register li $t0, 64 la $s4, method # jump to the instruction at the address in $s4 jr $s4 # $t0=$8 should contain 0x42 j Llbu
Llbu: # Load Byte Unsigned la $s4, data lbu $s3, 0($s4) # $s3 should contain the ASCII code for A which is 0x41. # load the unsigned byte value at memory address $s4+0 and store it in $s3
# Load Halfword lh $s3, 4($s4) # $s3 should contain the ASCII code for "EF" which is 0x4645 # load the signed halfword value at memory address $s4+4 and store it in $s3
# Load Linked Word (for multiprocessors) ll $s3, 8($s4) # $s3 should contain the memory word at [data+8] which is "IJKL". # load the word value at memory address $s4+8, and mark it as a reserved address for the atomic operation
# Load Upper Immediate lui $s3, 4 # load the immediate value 4 into the upper 16 bits of $s3, and set the lower 16 bits to 0 # $s3 should contain the result 0x40000 = 262,144
# Load Word lw $s3, 12($s4) # $s3 should contain the ASCII code for "IJKL" which is 0x4C4B4A49 # load the signed word value at memory address $s4+12 and store it in $s3
# Nor li $s4, 0xC # $s4 should contain the bits 1100 li $s5, 0x5 # $s5 should contain the bits 0101 nor $s3, $s4, $s5 # perform a bitwise NOR operation between $s4 and $s5 and store the result in $s3 # $s3 should contain the complement of 1101, which is 0010, which equal to 2.
# Or li $s4, 0xC # $s4 should contain the bits 1100 li $s5, 0x5 # $s5 should contain the bits 0101 or $s3, $s4, $s5 # perform a bitwise OR operation between $s4 and $s5 and store the result in $s3 # $s3 should contain 1101 = 13 = 0xD
# Or Immediate li $s4, 0xC # $s4 should contain the bits 1100 ori $s3, $s4, 5 # perform a bitwise OR operation between $s4 and the immediate value 5 and store the result in $s3
# $s3 should contain 1101 = 13 = 0xD
# Set Less Than li $s4, 8 li $s5, 9 slt $s3, $s4, $s5 # $s3 should contain 1 because 8 is less than 9. # if $s4 is less than $s5, set $s3 to 1; otherwise, set $s3 to 0
# Set Less Than Immediate li $s4, -105 slti $s3, $s4, -100 # $s3 should contain 1 because $s4= -105 is less than -100. # if $s4 is less than the immediate value -100, set $s3 to 1; otherwise, set $s3 to 0
# Set Less Than Immediate Unsigned li $s4, 105 sltiu $s3,$s4, 100 # $s3 should contain 0 because $s4= 105 is not less than 100. # if $s4 is less than the immediate unsigned value 100, set $s3 to 1; otherwise, set $set to 0.
# Set Less Than Unsigned li $s4, 180 li $s3, 160 sltu $t0, $s3, $s4 # $t0 should contain 0 because $s4=180 is not less than 160. # if $s3 is less than $s4, set $t0 to 1; otherwise, set $t0 to 0
# Shift Left Logical li $s4, 0xA # 1010 sll $t0, $s4, 5 # $t0 should contain 101000000 = 1 0100 0000 = 0x140 # shift the value in $s4 left by 5 bits and store the result in $t0
# Shift Right Logical li $s4, 0x56789ABC srl $t0, $s4, 8 # $t0 should contain 0x0056789A # shift the value in $s4 right by 8 bits and store the result in $t0
# Store Byte li $t0, 0x4D la $s4, data sb $t0, 4($s4) # [data+4] should contain 0x4D # store the least significant byte of $t0 at memory address $s4+4
# Store Conditional Word (for multiprocessors) li $s3, 0x12345678 sc $s3, 4($s4) # store the value of $t0 at memory address $s4+4, if the address is still reserved
# Store Halfword li $s3, 0x12345678 la $s4, data sh $s3, 8($s4) # [data+8] should contain 0x4C4B5678
# Store Word sw $s3, 12($s4) # [data+12] should contain 0x12345678
# Subtract li $s4, 12 li $s5, 7 sub $s3, $s4, $s5 # $s3 contains the value 5. # subtract the values in $s4 and $s5 and store the result in $s3
# Subtract Unsigned subu $s3, $s4, $s5 # subtract the unsigned values in $s4 and $s5 and store the result in $s3
# exit li $v0, 10 # system call for exit syscall
.data data: d0: .ascii "ABCD" # 0x44434241 d4: .ascii "EFGH" # 0x48474645 d8: .ascii "IJKL" # 0x4C4B4A49 dC: .ascii "MNOP" # 0x504F4E4D e0: .ascii "QRST" # 0x54535251
After I wrote the code, it implemented the 30 instructions that were listed from the MIPS Green Card page, ran the program, and everything became a success without any errors. The only thing that needs to be done on your behalf is according to the 30 instructions that were listed from the MIPS Green Card page and the entire code that I wrote is to explain in writing execution of every instructions, the affected values in registers, explain all the 30 instructions listed (according to the MIPS Green Card Page and the entire code that I wrote) how they function and operate as well as explaining the affected values in registers. Try to copy and paste the code that I wrote in the MIPS Mars Simulator and explain how each and all of the 30 instructions function and operate as well as explaining the affected values in register.
IL 18 Reference DataStep by Step Solution
There are 3 Steps involved in it
Step: 1
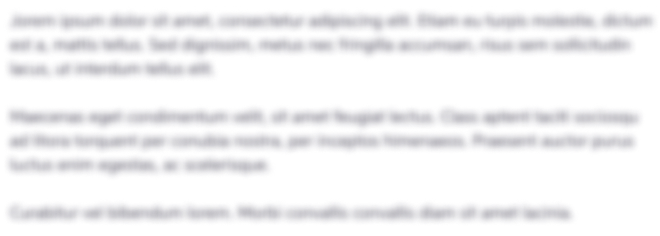
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started