Question
What is the complexity, Big- O Analysis for the code below and why? import java.util.ArrayList; import java.util.List; class Graph { private int numOfVertices;//number of vertices
What is the complexity, Big- O Analysis for the code below and why?
import java.util.ArrayList;
import java.util.List;
class Graph
{
private int numOfVertices;//number of vertices in graph
private String []vertices;//vertices array
private int [][]edges;//weights of edges matrix
public Graph(int num) //constructor to allocate space for matrix and vertices array
{
numOfVertices = num;
vertices = new String[numOfVertices];
edges = new int[numOfVertices][numOfVertices];
for(int i=0; i { for(int j=0; j { edges[i][j] = 0;//initially all are 0 meaning no edge exists //or we can allocate Integer.MAX_VALUE to each cell to represent no edge } } } public void setVertex(int index, String vertex) { vertices[index] = vertex;//sets vertices/cities names } public int getVertexNo(String vertex)//gets the position of city in matrix { for(int i=0;i { if(vertices[i].equals(vertex)) return i; } return -1;//returns -1 if city wasn't allocated } public void addEdge(String v1, String v2, int weightOfEdge)//adds and edge between two cities { int i = getVertexNo(v1);//retrieve the index of city/vertex int j = getVertexNo(v2);//retrieve the index of city/vertex if(i != -1 && j != -1)//if city exists { edges[i][j] = weightOfEdge;//sets edge for both cities indices edges[j][i] = weightOfEdge;//both are reachable through a single edge } } public int getDistance(String v1, String v2) { int i = getVertexNo(v1);//retrieve the index of city/vertex int j = getVertexNo(v2);//retrieve the index of city/vertex if(i != -1 && j != -1)//if cities exists if(edges[i][j] != 0)//if edge exists return edges[i][j];//returns the edge value return Integer.MAX_VALUE;//else maximum integer value is returned } public List { int i = getVertexNo(v1);//retrieve the index of city/vertex List if(i != -1)//if city exists for(int j=0; j { if(edges[i][j] != 0)//if edge exists neighbors.add(vertices[j]);//add the neighbor to list } return neighbors;//return the list } public void print() { for(int j=0; j { System.out.println(vertices[j]+"("+(char)(65+j)+") "); //we're representing city names with A to O (15 cities) rather than their complete names //for better display format of matrix //using implicit casting (char)(65+j) for A to O } System.out.println(""); System.out.print(" ");//adjusting spaces for(int j=0; j { System.out.print((char)(65+j)+"\t"); }//first row of matrix with column names as A to O System.out.println(""); for(int i=0; i { System.out.print((char)(65+i)+" ");//row numbers from A to O for(int j=0; j { System.out.print(edges[i][j]+"\t");//edge weight between cities/vertices } System.out.println(""); } } } public class Main { public static void main(String[] args) { Graph g = new Graph(15);//graph with 15 vertices //setting all city names explicitly g.setVertex(0,"Mohave"); g.setVertex(1,"Coconino"); g.setVertex(2,"Navajo"); g.setVertex(3,"Apache"); g.setVertex(4,"Greenlee"); g.setVertex(5,"Cochise"); g.setVertex(6,"Santa Cruz"); g.setVertex(7,"Pima"); g.setVertex(8,"Pinal"); g.setVertex(9,"Graham"); g.setVertex(10,"Gila"); g.setVertex(11,"Yavapai"); g.setVertex(12,"La Paz"); g.setVertex(13,"Yuma"); g.setVertex(14,"Maricopa"); //adding edges between cities g.addEdge("Mohave","Coconino",14); g.addEdge("Mohave","Yavapai",14); g.addEdge("Mohave","La Paz",9); g.addEdge("Coconino","Navajo",9); g.addEdge("Coconino","Gila",17); g.addEdge("Coconino","Yavapai",9); g.addEdge("Navajo","Gila",13); g.addEdge("Navajo","Graham",20); g.addEdge("Navajo","Apache",5); g.addEdge("Apache","Greenlee",17); g.addEdge("Apache","Graham",19); g.addEdge("Greenlee","Cochise",16); g.addEdge("Greenlee","Graham",4); g.addEdge("Cochise","Graham",12); g.addEdge("Cochise","Pima",9); g.addEdge("Cochise","Santa Cruz",8); g.addEdge("Santa Cruz","Pima",6); g.addEdge("Pima","Graham",12); g.addEdge("Pima","Pinal",7); g.addEdge("Pima","Maricopa",10); g.addEdge("Pima","Yuma",23); g.addEdge("Pinal","Graham",13); g.addEdge("Pinal","Gila",5); g.addEdge("Pinal","Maricopa",6); g.addEdge("Graham","Gila",7); g.addEdge("Gila","Yavapai",18); g.addEdge("Gila","Maricopa",8); g.addEdge("Yavapai","La Paz",9); g.addEdge("Yavapai","Maricopa",16); g.addEdge("La Paz","Maricopa",15); g.addEdge("La Paz","Yuma",11); g.addEdge("Maricopa","Yuma",16); //list of neighbors for Maricopa List System.out.print("Neighbor cities of Maricopa : "); System.out.println(myList);//display list System.out.print("Distance between Maricopa and Yuma : "+g.getDistance("Maricopa","Yuma")+" ");//distance between two cities g.print();//adjacency matrix } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
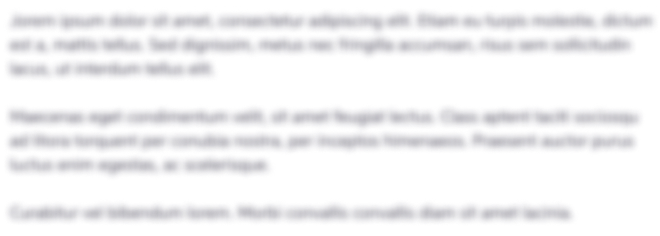
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started