Question
What is the finished code in Java? Some code to start is provided. Follow instructions. package listVsSet; public class ColoredSquare { } ------------------------------------------------------------ package listVsSet;
What is the finished code in Java? Some code to start is provided. Follow instructions.
package listVsSet;
public class ColoredSquare {
}
------------------------------------------------------------
package listVsSet; public class createDemoPanel { }
--------------------------------------------------------
package listVsSet;
import java.awt.Color;
/** * Test Client for class ListVsSetDemo * * @author Margret Posch */ public class ListVsSetConsoleApp { public static void main(String[] args) {
// // notice: I pass the individual elements, // because the constructor is implemented with varargs // ListVsSetDemo demo = new ListVsSetDemo( new ColoredSquare(14, Color.BLUE), new ColoredSquare(18, Color.RED), // new ColoredSquare(12, Color.YELLOW), new ColoredSquare(18, Color.RED), new ColoredSquare(16, Color.GREEN)); // // System.out.println("List: " + demo.getListElements()); System.out.println("Set: " + demo.getSetElements()); // // System.out.println(" Adding a new element:"); demo.addElement(new ColoredSquare(10, Color.ORANGE)); // System.out.println("List: " + demo.getListElements()); System.out.println("Set: " + demo.getSetElements()); // // System.out.println(" Adding a duplicate element:"); demo.addElement(new ColoredSquare(10, Color.ORANGE)); // System.out.println("List: " + demo.getListElements()); System.out.println("Set: " + demo.getSetElements()); } }
--------------------------------------------------------------------------------------------------
package listVsSet; public class ListVsSetDemo { }
---------------------------------------------------------------------------------
package listVsSet;
import java.awt.BorderLayout; import java.awt.CardLayout; import java.awt.Color; import java.awt.EventQueue; import java.awt.Font;
import javax.swing.JFrame; import javax.swing.JMenuBar; import javax.swing.JMenuItem; import javax.swing.JPanel; import javax.swing.JTextArea; import javax.swing.border.EmptyBorder; import java.awt.event.ActionListener; import java.awt.event.ActionEvent; import javax.swing.JRadioButton; import java.awt.GridLayout; import javax.swing.JLabel;
/** * Gui application that describes the two main difference between the * interfaces List and Set and that demonstrates the different * behaviors of a list and a set. * * @authors .............. and Margret Posch * */ public class ListVsSetGui extends JFrame { private static final long serialVersionUID = -942561673068858105L; private JPanel contentPane; private CardLayout cardLayout = new CardLayout(); private JTextArea textField;
/** * Launch the application. */ public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { try { ListVsSetGui frame = new ListVsSetGui(); frame.setVisible(true); } catch (Exception e) { e.printStackTrace(); } } }); }
/** * Create the frame. */ public ListVsSetGui() { setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setBounds(100, 100, 700, 450); setTitle("List vs Set Demo"); JMenuBar menuBar = createMenuBar(); setJMenuBar(menuBar); contentPane = new JPanel(); contentPane.setBorder(new EmptyBorder(5, 5, 5, 5)); setContentPane(contentPane); contentPane.setLayout(cardLayout); // main difference panel JPanel mainDifferencePanel = createMainDifferencePanel(); contentPane.add(mainDifferencePanel, "main difference"); // demo panel JPanel demoPanel = createDemoPanel(); contentPane.add(demoPanel, "demo"); }
/** * Creates a panel that demonstrates the two main differences * between lists and sets. * It does so by adding the same elements to the list and the set * and then displaying the contents of both the list and the set. * * @return the panel that demonstrates the main differences * between lists and sets. */ private JPanel createDemoPanel() { JPanel demoPanel = new JPanel(); demoPanel.setLayout(new BorderLayout()); // control panel JPanel controlPanel = new JPanel(); controlPanel.setBorder(new EmptyBorder(15, 12, 0, 12)); controlPanel.setLayout(new GridLayout(9, 0, 0, 0)); JLabel lblYourChoice = new JLabel("Your Choice:"); lblYourChoice.setFont(new Font("Tahoma", Font.PLAIN, 12)); controlPanel.add(lblYourChoice); JRadioButton rdbtnListElements = new JRadioButton("List Elements"); controlPanel.add(rdbtnListElements); JRadioButton rdbtnSetElements = new JRadioButton("Set Elements"); controlPanel.add(rdbtnSetElements); demoPanel.add(controlPanel, BorderLayout.WEST); // text field textField = new JTextArea(); textField.setFont(new Font("Monospaced", Font.PLAIN, 13)); textField.setBorder(new EmptyBorder(20, 20, 20, 15)); textField.setColumns(10); demoPanel.add(textField, BorderLayout.CENTER);
return demoPanel; }
/** * Creates a panel that describes the two main differences * between lists and sets. */ private JPanel createMainDifferencePanel() { JPanel mainDifferencePanel = new JPanel(); mainDifferencePanel.setLayout(new BorderLayout(0, 0)); JTextArea txtrTheMain = new JTextArea(); txtrTheMain.setText("The 2 main differences between interface List and Set are:"); txtrTheMain.setBorder(new EmptyBorder(15, 15, 15, 15)); txtrTheMain.setFont(new Font("Arial", Font.PLAIN, 20)); txtrTheMain.setBackground(new Color(141, 141, 131)); txtrTheMain.setForeground(Color.WHITE); mainDifferencePanel.add(txtrTheMain); return mainDifferencePanel; }
/** * Create a menu bar with the following choices: * Demo the behavior of lists and sets * Describe the two main differences between lists and sets * Exit the application * * @return the menu bar */ private JMenuBar createMenuBar() { JMenuBar menuBar = new JMenuBar(); // menu item "Demo" JMenuItem mntmDemo = new JMenuItem("Demo"); mntmDemo.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { cardLayout.show(contentPane, "demo"); } }); mntmDemo.setFont(new Font("Segoe UI", Font.PLAIN, 14)); menuBar.add(mntmDemo); // menu item "Compare List and Set" JMenuItem mntmCompareListAndSet = new JMenuItem("Compare List and Set"); mntmCompareListAndSet.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { cardLayout.show(contentPane, "main difference"); } }); mntmCompareListAndSet.setFont(new Font("Segoe UI", Font.PLAIN, 14)); menuBar.add(mntmCompareListAndSet); // menu item "Exit" JMenuItem mntmExit = new JMenuItem("Exit"); mntmExit.setFont(new Font("Segoe UI", Font.PLAIN, 14)); menuBar.add(mntmExit); return menuBar; }
}
Console Output: List: side: 14 #0000FF side:18 #FF0000 side : 12 #FFFF00 side:18 #FF0000 side: 16 #00FF00 Learning Objectives: Implement equals and hashCode to compare objects of a custom class User the internet to learn about the interfaces List and Set, and class HashSet Describe the differences between the interfaces List and Set Create, initialize, and display a HashSet "Modify an existing GUI application based on specific instructions Set: side :14 #0000FF side : 12 #FFFF00 side: 16 #00FF00 side :18 #FF0000 Demonstrate the benefits of separating functionality and display by using class ColoredSquare both in a console application and in a GUI application Description: Download the starter project and import it into Eclipse Import > Existing Projects Into Workspace Implement the classes ColoredSquare and ListVsSetDemo as described below. Uncomment the code in class ListVsSetConsoleApp to test your classes. This test client should not be changed Once you verified that the classes ColoredSquare and ListVsSetDemo work as expected modify the Gui Application according to the description below Adding a new element: List: side :14 #0000FF side: 18 #FF0000 side:12 #FFFF00 side: 18 #FF0000 side:16 #00FF00 side: 10 #FFC800 Set: side: 14 #0000FF side:12 #FFFF00 side: 10 #FFC800 side: 16 #00FF00 side:18 #FF0000 Ad Coloredsquare: Implement ColoredSquare according to the UML diagram. No class members should be added or removed Important: ColoredSquare should NOT include any print methods Adding a duplicate element: List: side :14 #0000FF side :18 #FF0000 side:12 #FFFF00 side: 18 #FF0000 side:16 #00FF00 side: 10 #FFC800 side: 10 #FFC800 ColoredSquare - side int Hint import java.awt.Color color Color + ColoredSquare (s int, c: Color) + area (): int + equals Object obj): boolean + hashCode () int + toString StringgStep by Step Solution
There are 3 Steps involved in it
Step: 1
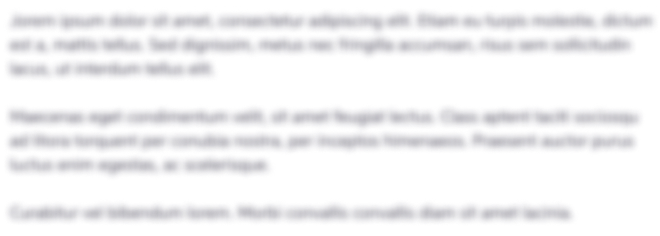
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started