Answered step by step
Verified Expert Solution
Question
1 Approved Answer
When a function accepts arguments, the valid data types and (if relevant) value ranges for these arguments are specified in the problem. The problem
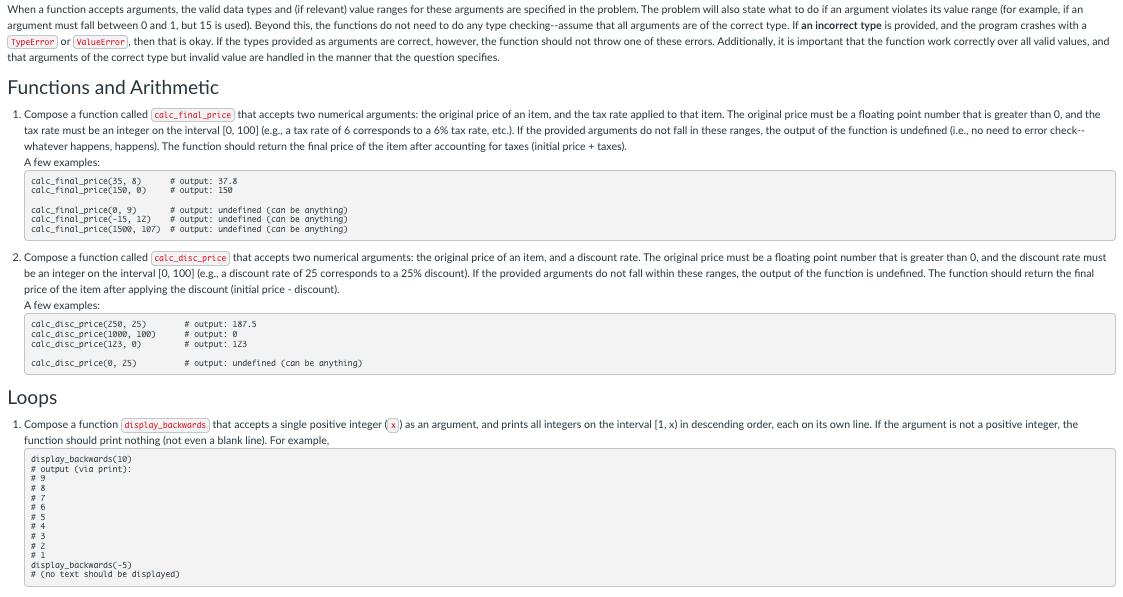
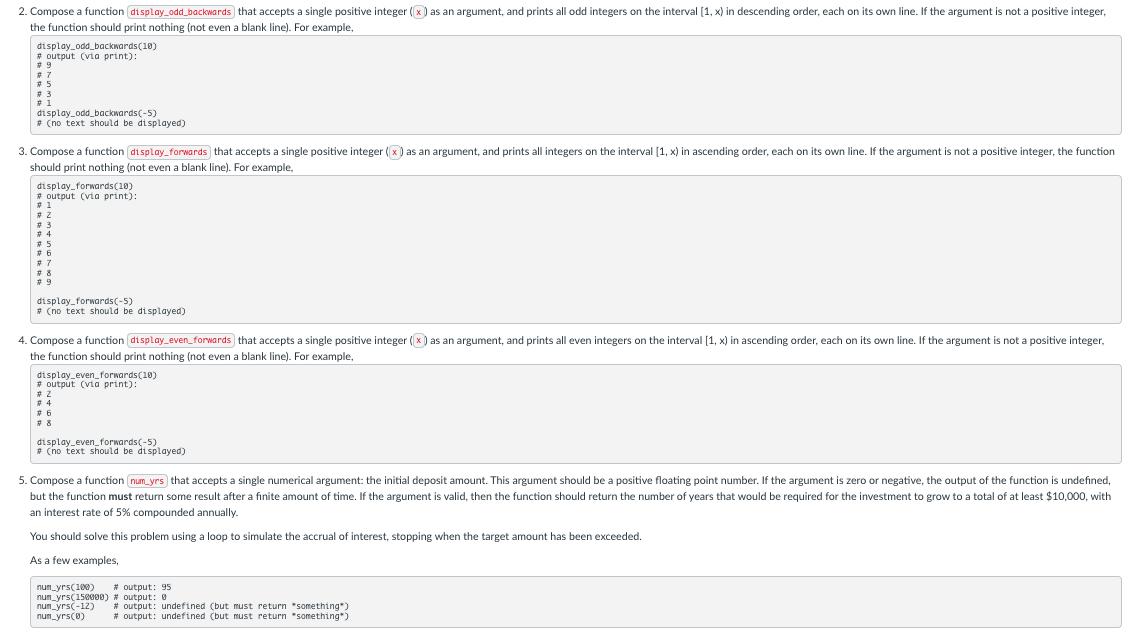
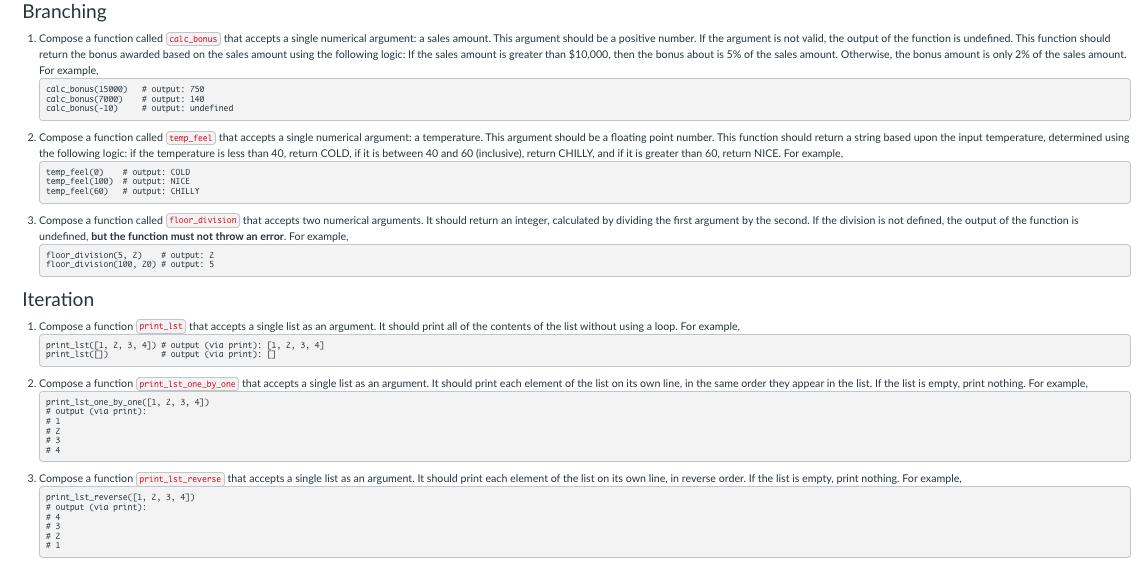
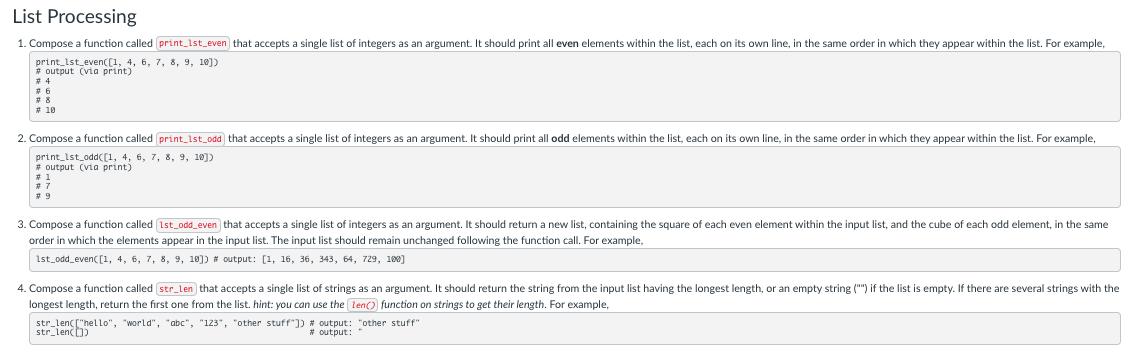
When a function accepts arguments, the valid data types and (if relevant) value ranges for these arguments are specified in the problem. The problem will also state what to do if an argument violates its value range (for example, if an argument must fall between 0 and 1, but 15 is used). Beyond this, the functions do not need to do any type checking--assume that all arguments are of the correct type. If an incorrect type is provided, and the program crashes with a TypeError or ValueError, then that is okay. If the types provided as arguments are correct, however, the function should not throw one of these errors. Additionally, it is important that the function work correctly over all valid values, and that arguments of the correct type but invalid value are handled in the manner that the question specifies. Functions and Arithmetic 1. Compose a function called calc_final_price] that accepts two numerical arguments: the original price of an item, and the tax rate applied to that item. The original price must be a floating point number that is greater than 0, and the tax rate must be an integer on the interval [0, 100] (e.g., a tax rate of 6 corresponds to a 6% tax rate, etc.). If the provided arguments do not fall in these ranges, the output of the function is undefined (i.e., no need error check-- whatever happens, happens). The function should return the final price of the item after accounting for taxes (initial price + taxes). A few examples: calc_final_price(35, 8) calc_final_price(150, 0) calc_final_price(0, 9) calc_final_price(-15, 12) calc_final_price(1500, 107) calc_disc_price(250, 25). calc_disc_price(1000, 100) calc_disc_price(123, 0) calc_disc_price(0, 25) 2. Compose a function called calc_disc_price) that accepts two numerical arguments: the original price of an item, and a discount rate. The original price must be a floating point number that is greater than 0, and the discount rate must be an integer on the interval [0, 100] (e.g., a discount rate of 25 corresponds to a 25% discount). If the provided arguments do not fall within these ranges, the output of the function is undefined. The function should return the final price of the item after applying the discount (initial price - discount). A few examples: display_backwards(10) #output (via print): #9 #8 #7 #6 output: 37.8 * output: 150 Loops 1. Compose a function display_backwards that accepts a single positive integer (x) as an argument, and prints all integers on the interval [1, x) in descending order, each on its own line. If the argument is not a positive integer, the function should print nothing (not even a blank line). For example, #5 #4 #output: undefined (can be anything) #output: undefined (can be anything) * output: undefined (can be anything). #3 #2 #1 # output: 187.5 # output: 0 # output: 123 # output: undefined (can be anything) display_backwards(-5) # (no text should be displayed) 2. Compose a function display_add_backwards that accepts a single positive integer (x) as an argument, and prints all odd integers on the interval [1, x) in descending order, each on its own line. If the argument is not a positive integer, the function should print nothing (not even a blank line). For example, display_odd_backwards (10) #output (via print): #9 #7 # 5 #3 # 1 display_odd_backwards(-5) # (no text should be displayed) 3. Compose a function display_forwards that accepts a single positive integer (x) as an argument, and prints all integers on the interval [1, x) in ascending order, each on its own line. If the argument is not a positive integer, the function should print nothing (not even a blank line). For example, display_forwards(10) #output (via print): #1 #2 # 3 #4 #5 #6 #7 #8 #9 display_forwards(-5) # (no text should be displayed) 4. Compose a function display_even_forwards that accepts a single positive integer (x) as an argument, and prints all even integers on the interval [1, x) in ascending order, each on its own line. If the argument is not a positive integer, the function should print nothing (not even a blank line). For example, display_even_forwards(10) #output (via print): #2 #4 #6 #8 display_even_forwards(-5) # (no text should be displayed) 5. Compose a function [num_yrs that accepts a single numerical argument: the initial deposit amount. This argument should be a positive floating point number. If the argument is zero or negative, the output of the function is undefined, but the function must return some result after a finite amount of time. If the argument is valid, then the function should return the number of years that would be required for the investment to grow to a total of at least $10,000, with an interest rate of 5% compounded annually. You should solve this problem using a loop to simulate the accrual of interest, stopping when the target amount has been exceeded. As a few examples, num yrs(100) #output: 95 num yrs(150000) # output: 0 num_yrs(-12) # output: undefined (but must return num_yrs(0) something") # output: undefined (but must return something"). Branching 1. Compose a function called catc_bonus that accepts a single numerical argument: a sales amount. This argument should be a positive number. If the argument is not valid, the output of the function is undefined. This function should return the bonus awarded based on the sales amount using the following logic: If the sales amount is greater than $10,000, then the bonus about is 5% of the sales amount. Otherwise, the bonus amount is only 2% of the sales amount. For example, calc_bonus(15000) calc bonus(7000) calc_bonus(-10) 2. Compose a function called temp_feel that accepts a single numerical argument: a temperature. This argument should be a floating point number. This function should return a string based upon the input temperature, determined using the following logic: if the temperature is less than 40, return COLD, if it is between 40 and 60 (inclusive), return CHILLY, and if it is greater than 60, return NICE. For example. # output: 750 #output: 140 #output: undefined temp_feel(0) #output: COLD temp_feel(100) # output: NICE temp_feel(60) # output: CHILLY 3. Compose a function called floor_division that accepts two numerical arguments. It should return an integer, calculated by dividing the first argument by the second. If the division is not defined, the output of the function is undefined, but the function must not throw an error. For example, floor_division (5, 2) # output: 2 floor_division (100, 20) # output: 5 Iteration 1. Compose a function print_1st that accepts a single list as an argument. It should print all of the contents of the list without using a loop. For example, print 1st([1, 2, 3, 4]) # output (via print): 1, 2, 3, 4] print 1st([]) #output (via print): 2. Compose a function print_1st_one_by_one that accepts a single list as an argument. It should print each element of the list on its own line, in the same order they appear in the list. If the list is empty, print nothing. For example, print_1st_one_by_one([1, 2, 3, 4]) #output (via print): #1 # 2 #3 74 3. Compose a function print_1st_reverse that accepts a single list as an argument. It should print each element of the list on its own line, in reverse order. If the list is empty, print nothing. For example, print_1st_reverse([1, 2, 3, 4]) #output (via print): # 4 #3 #2 #1 List Processing 1. Compose a function called print_1st_even that accepts a single list of integers as an argument. It should print all even elements within the list, each on its own line, in the same order in which they appear within the list. For example, print_1st_even([1, 4, 6, 7, 8, 9, 10]) #output (via print) #4 #6 #8 #10 2. Compose a function called print_1st_odd] that accepts a single list of integers as an argument. It should print all odd elements within the list, each on its own line, in the same order in which they appear within the list. For example, print_1st_odd([1, 4, 6, 7, 8, 9, 10]) #output (via print) # 1 #7 #9 3. Compose a function called (1st_odd_even that accepts a single list of integers as an argument. It should return a new list, containing the square of each even element within the input list, and the cube of each odd element, in the same order in which the elements appear in the input list. The input list should remain unchanged following the function call. For example, 1st odd even([1, 4, 6, 7, 8, 9, 10]) # output: [1, 16, 36, 343, 64, 729, 100] 4. Compose a function called str_len that accepts a single list of strings as an argument. It should return the string from the input list having the longest length, or an empty string ("") if the list is empty. If there are several strings with the longest length, return the first one from the list. hint: you can use the len() function on strings to get their length. For example, str_len(["hello", "world", "abc", "123", "other stuff"]) # output: "other stuff" str_len([]) # output: When a function accepts arguments, the valid data types and (if relevant) value ranges for these arguments are specified in the problem. The problem will also state what to do if an argument violates its value range (for example, if an argument must fall between 0 and 1, but 15 is used). Beyond this, the functions do not need to do any type checking--assume that all arguments are of the correct type. If an incorrect type is provided, and the program crashes with a TypeError or ValueError), then that is okay. If the types provided as arguments are correct, however, the function should not throw one of these errors. Additionally, it is important that the function work correctly over all valid values, and that arguments of the correct type but invalid value are handled in the manner that the question specifies. Functions and Arithmetic 1. Compose a function called calc_final_price) that accepts two numerical arguments: the original price of an item, and the tax rate applied to that item. The original price must be a floating point number that is greater than 0, and the tax rate must be an integer on the interval [0, 100] (e.g., a tax rate of 6 corresponds to a 6% tax rate, etc.). If the provided arguments do not fall in these ranges, the output of the function is undefined (i.e., no need error check-- whatever happens, happens). The function should return the final price of the item after accounting for taxes (initial price + taxes). A few examples: calc_final_price(35, 8) calc_final_price(150, 0) calc_final_price(0, 9) calc_final_price(-15, 12) calc_final_price(1500, 107) calc disc price(250, 25). calc_disc_price(1000, 100) calc disc price(123, 0) calc disc_price(0, 25) 2. Compose a function called calc_disc_price) that accepts two numerical arguments: the original price of an item, and a discount rate. The original price must be a floating point number that is greater than 0, and the discount rate must be an integer on the interval [0, 100] (e.g., a discount rate of 25 corresponds to a 25% discount). If the provided arguments do not fall within these ranges, the output of the function is undefined. The function should return the final price of the item after applying the discount (initial price - discount). A few examples: display_backwards(10) #output (via print): #9 #8 #7 #6 output: 37.8 * output: 150 Loops 1. Compose a function display_backwards that accepts a single positive integer (x) as an argument, and prints all integers on the interval [1, x) in descending order, each on its own line. If the argument is not a positive integer, the function should print nothing (not even a blank line). For example, #5 #4 #output: undefined (can be anything) #output: undefined (can be anything) * output: undefined (can be anything). #3 #2 #1 # output: 187.5 # output: 0 # output: 123 # output: undefined (can be anything) display_backwards(-5) # (no text should be displayed) 2. Compose a function display_add_backwards that accepts a single positive integer (x) as an argument, and prints all odd integers on the interval [1, x) in descending order, each on its own line. If the argument is not a positive integer, the function should print nothing (not even a blank line). For example, display_odd_backwards (10) #output (via print): #9 #7 # 5 #3 # 1 display_odd_backwards(-5) # (no text should be displayed) 3. Compose a function display forwards that accepts a single positive integer (x) as an argument, and prints all integers on the interval [1, x) in ascending order, each on its own line. If the argument is not a positive integer, the function should print nothing (not even a blank line). For example, display_forwards(10) #output (via print): #1 #2 # 3 #4 #5 #6 #7 #8 #9 display_forwards(-5) # (no text should be displayed) 4. Compose a function display_even_forwards that accepts a single positive integer (x) as an argument, and prints all even integers on the interval [1, x) in ascending order, each on its own line. If the argument is not a positive integer, the function should print nothing (not even a blank line). For example, display_even_forwards(10) #output (via print): #2 #4 #6 #8 display_even_forwards(-5) # (no text should be displayed) 5. Compose a function (num_yrs that accepts a single numerical argument: the initial deposit amount. This argument should be a positive floating point number. If the argument is zero or negative, the output of the function is undefined, but the function must return some result after a finite amount of time. If the argument is valid, then the function should return the number of years that would be required for the investment to grow to a total of at least $10,000, with an interest rate of 5% compounded annually. You should solve this problem using a loop to simulate the accrual of interest, stopping when the target amount has been exceeded. As a few examples, num yrs(100) #output: 95 num yrs(150000) # output: 0 num yrs(-12) num yrs(0) # output: undefined (but must return something") # output: undefined (but must return something"). Branching 1. Compose a function called catc_bonus that accepts a single numerical argument: a sales amount. This argument should be a positive number. If the argument is not valid, the output of the function is undefined. This function should return the bonus awarded based on the sales amount using the following logic: If the sales amount is greater than $10,000, then the bonus about is 5% of the sales amount. Otherwise, the bonus amount is only 2% of the sales amount. For example, calc bonus(15000) calc bonus (7000) calc bonus(-10) 2. Compose a function called temp_feel that accepts a single numerical argument: a temperature. This argument should be a floating point number. This function should return a string based upon the input temperature, determined using the following logic: if the temperature is less than 40, return COLD, if it is between 40 and 60 (inclusive), return CHILLY, and if it is greater than 60, return NICE. For example. temp_feel(0) temp_feel (100) # output: 750 #output: 140 #output: undefined #output: COLD # output: NICE temp_feel(60) # output: CHILLY 3. Compose a function called floor_division that accepts two numerical arguments. It should return an integer, calculated by dividing the first argument by the second. If the division is not defined, the output of the function is undefined, but the function must not throw an error. For example, floor_division (5, 2) # output: 2 floor_division (100, 20) # output: 5 Iteration 1. Compose a function print_1st that accepts a single list as an argument. It should print all of the contents of the list without using a loop. For example, print 1st([1, 2, 3, 4]) # output (via print): 1, 2, 3, 4] print 1st([]) #output (via print): 2. Compose a function print_1st_one_by_one that accepts a single list as an argument. It should print each element of the list on its own line, in the same order they appear in the list. If the list is empty, print nothing. For example, print_1st_one_by_one([1, 2, 3, 4]) #output (via print): #1 # 2 #3 74 3. Compose a function print_1st_reverse that accepts a single list as an argument. It should print each element of the list on its own line, in reverse order. If the list is empty, print nothing. For example, print_1st_reverse([1, 2, 3, 4]) #output (via print): # 4 #3 #2 #1 List Processing 1. Compose a function called print_1st_even that accepts a single list of integers as an argument. It should print all even elements within the list, each on its own line, in the same order in which they appear within the list. For example, print_1st_even([1, 4, 6, 7, 8, 9, 10]) #output (via print) #4 #6 #8 #10 2. Compose a function called print_1st_odd] that accepts a single list of integers as an argument. It should print all odd elements within the list, each on its own line, in the same order in which they appear within the list. For example, print_1st_odd([1, 4, 6, 7, 8, 9, 10]) #output (via print) # 1 #7 #9 3. Compose a function called 1st_odd_even that accepts a single list of integers as an argument. It should return a new list, containing the square of each even element within the input list, and the cube of each odd element, in the same order in which the elements appear in the input list. The input list should remain unchanged following the function call. For example, 1st odd even([1, 4, 6, 7, 8, 9, 10]) # output: [1, 16, 36, 343, 64, 729, 100] 4. Compose a function called str_len that accepts a single list of strings as an argument. It should return the string from the input list having the longest length, or an empty string ("") if the list is empty. If there are several strings with the longest length, return the first one from the list. hint: you can use the len() function on strings to get their length. For example, str_len(["hello", "world", "abc", "123", "other stuff"]) # output: "other stuff" str_len([]) # output:
Step by Step Solution
★★★★★
3.41 Rating (148 Votes )
There are 3 Steps involved in it
Step: 1
Here are the functions you requested 1 calcfinalprice function python def calcfinal...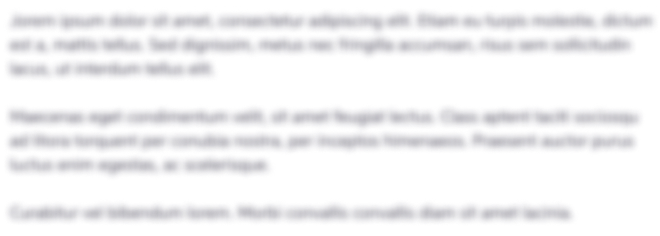
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started