Question
When I go to compile these by entering gcc Store.cpp, I am getting the following errors: Store.hpp:1:8: error: no macro name given in #ifndef directive
When I go to compile these by entering gcc Store.cpp, I am getting the following errors:
Store.hpp:1:8: error: no macro name given in #ifndef directive #ifndef ^ Store.cpp:8:6: error: Store has not been declared void Store::addProduct(Product *p) { ^ Store.cpp:8:24: error: variable or field addProduct declared void void Store::addProduct(Product *p) { ^ Store.cpp:8:24: error: Product was not declared in this scope Store.cpp:8:33: error: p was not declared in this scope void Store::addProduct(Product *p) { ^ Store.cpp:16:6: error: Store has not been declared void Store::addMember(Customer *c) { ^ Store.cpp:16:23: error: variable or field addMember declared void void Store::addMember(Customer *c) { ^ Store.cpp:16:23: error: Customer was not declared in this scope Store.cpp:16:33: error: c was not declared in this scope void Store::addMember(Customer *c) { ^ Store.cpp:25:1: error: Product does not name a type Product *Store::getProductFromID(std::string item) { ^ Store.cpp:43:1: error: Customer does not name a type Customer *Store::getMemberFromID(std::string cx) { ^ Store.cpp:67:6: error: Store has not been declared void Store::productSearch(std::string str) { ^ Store.cpp: In function void productSearch(std::string): Store.cpp:72:18: error: inventory was not declared in this scope double invSize = inventory.size(); ^ Store.cpp: At global scope: Store.cpp:118:6: error: Store has not been declared void Store::addProductToMemberCart(std::string pID, std::string mID) { ^ Store.cpp: In function void addProductToMemberCart(std::string, std::string): Store.cpp:125:18: error: inventory was not declared in this scope double invSize = inventory.size(); ^ Store.cpp:126:21: error: members was not declared in this scope double memberSize = members.size(); ^ Store.cpp: At global scope: Store.cpp:199:6: error: Store has not been declared void Store::checkOutMember(std::string mID) ^ Store.cpp: In function void checkOutMember(std::string): Store.cpp:203:18: error: members was not declared in this scope int memberSize = members.size(); ^ Store.cpp:232:21: error: inventory was not declared in this scope for (int k = 0; k < inventory.size(); k++)
Here are my codes:
Customer.cpp
#include "Customer.hpp"
/************************************************************** * Customer::Customer * Description: takes as parameters three values with which to * initialize the Customer's name, account ID, and whether the * customer is a premium member. **************************************************************/ Customer::Customer(std::string n, std::string a, bool pm) { name = n; accountID = a; premiumMember = pm; }
/************************************************************** * Customer::getAccountID * Description: returns the values stored in that Customer's * AccountID variable. **************************************************************/ std::string Customer::getAccountID() { return accountID; }
/************************************************************** * Customer::getCart * Description: return the value stored in taht Customer's * Cart variable. **************************************************************/ std::vector
/************************************************************** * Customer::addProductToCart * Description: adds the product ID code (as a string) to the * Customer's personal cart. **************************************************************/ void Customer::addProductToCart(std::string item) { cart.push_back(item); }
/************************************************************** * Customer::isPremiumMember * Description: retruns a boolean value that checks if the user * is registered as a premium member. **************************************************************/ bool Customer::isPremiumMember() { // Check to see if the user is a premium member, if so return true. if (premiumMember) { return true; } // Else return false else { return false; } }
/************************************************************** * Customer::emptyCart * Description: removes all of the items in that Customer's * cart. **************************************************************/ void Customer::emptyCart() { cart.clear(); }
Product.cpp
#include "Product.hpp"
/************************************************************** * Product::Product * Description: takes as parameters five values with which to * initialize the Product's idCode, title, description, price, * and quantity available **************************************************************/ Product::Product(std::string id, std::string t, std::string d, double p, int qa) { idCode = id; title = t; description = d; price = p; quantityAvailable = qa;
}
/************************************************************** * Product::getIDCode * Description: return the value stored within that Product's * ID Code Variable. **************************************************************/ std::string Product::getIdCode() { return idCode; }
/************************************************************** * Product::getTitle * Description: return the value within that Product's Title * Variable. **************************************************************/ std::string Product::getTitle() { return title; }
/************************************************************** * Product::getDescription * Description: return the value stored within that Product's * Description Variable. **************************************************************/ std::string Product::getDescription() { return description; }
/************************************************************** * Product::getPrice * Description: return the value stored within tha Product's * Price Variable. **************************************************************/ double Product::getPrice() { return price; }
/************************************************************** * Product::getQuantityAvailable * Description: return the value stored within that Product's * Quantity Available Variable. **************************************************************/ int Product::getQuantityAvailable() { return quantityAvailable; }
/************************************************************** * Product::decreaseQuantity * Description: decreases the quantity available by one within * that Product. **************************************************************/ void Product::decreaseQuantity() { quantityAvailable --; }
Store.cpp
#include "Store.hpp" #include
/************************************************************** * Store::addProduct * Description: adds a product to the inventory of the store **************************************************************/ void Store::addProduct(Product *p) { inventory.push_back(p); }
/************************************************************** * Store::addMember * Description: adds a customer to the members of the store **************************************************************/ void Store::addMember(Customer *c) { members.push_back(c); }
/************************************************************** * Store::getProductFromID * Description: returns pointer to product with matching ID. * Returns NULL if no matching ID is found. **************************************************************/ Product *Store::getProductFromID(std::string item) { // Search through the exact amount of inventory items at that moment for(int i = 0; i < inventory.size(); i++) { // If the item code matches on in our inventory, return a pointer to it if (item == inventory[i]->getIdCode()) { return inventory[i]; } } return NULL; }
/************************************************************** * Store::getMemberFromID * Description: returns pointer to customer with matching ID. * Returns NULL if no matching ID is found. **************************************************************/ Customer *Store::getMemberFromID(std::string cx) { // Search through the exact amount of currently registered members for(int i = 0; i < members.size(); i++) { // If the current member search ID is equivalent to the customer query if (cx == members[i]->getAccountID()) { // return the pointer to that member return members[i]; } } return NULL; }
/************************************************************** * Store::productSearch * Description: for every product whose title or description * contains the search string, prints out that product's title, * ID code, price and description. The first letter of the * search string should be case-insensitive, i.e. a search for * "wood" should match Products that have "Wood" in their title * or description, and a search for "Wood" should match * Products that have "wood" in their title or description. **************************************************************/ void Store::productSearch(std::string str) { // Set an exists boolean variable to check the items is in inventory bool exists = false;
// Save the current size of the inventory double invSize = inventory.size();
for (int i = 0; i < invSize; i++) { // Set the first letter to a lower case in the title std::string itemTitle = inventory.at(i)->getTitle(); itemTitle[0] = tolower(itemTitle[0]);
// Set the first letter to a lower case in the description std::string itemDesc = inventory.at(i)->getDescription(); itemDesc[0] = tolower(itemDesc[0]);
// Set the first letter to a lower case in the search term str[0] = tolower(str[0]);
// Utilized the find function learned of npos from the .find() man pages if (itemTitle.find(str)!=std::string::npos || itemDesc.find(str)!=std::string::npos) { exists = true; std::cout << inventory[i]->getTitle() << std::endl; std::cout << "ID Code: " << inventory[i]->getIdCode() << std::endl; std::cout << "Price: $" << inventory[i]->getPrice() << std::endl; std::cout << inventory[i]->getDescription() << std::endl; std::cout << " "; } } // If the product is not found in inventory, alert the user. if (!exists) { std::cout << "There are no items that match this search. Try again! " << std::endl; } }
/************************************************************** * Store::addProductToMemberCart * Description: If the product isn't found in the inventory, * print "Product #[idCode goes here] not found." If the * member isn't found in the members, print "Member #[accountID * goes here] not found." If both are found and the product is * still available, calls the member's addProductToCart method. * Otherwise it prints "Sorry, product #[idCode goes here] is * currently out of stock." The same product can be added * multiple times if the customer wants more than one of * something. **************************************************************/ void Store::addProductToMemberCart(std::string pID, std::string mID) { // Variables needed to keep track on items and assist with readability bool inStore = false; bool inMember = false; bool inStock = false; int itemLocation = -1; int memberLocation = -1; double invSize = inventory.size(); double memberSize = members.size();
// Check if item is in the store for (int i = 0; i < invSize; i++) { // If we are able to find the item, mark inStore as true and save it's location if (inventory[i]->getIdCode() == pID) { inStore = true; itemLocation = i;
// Check if the item is in stock if (inventory[i]->getQuantityAvailable() > 0) { inStock = true; } } } // Check if individual is a member for (int j = 0; j < memberSize; j++) { if (members[j]->getAccountID() == mID) { inMember = true; memberLocation = j; } } // Check to make sure they are a member, the item is in stock, and we have the item if (inMember && inStock && inStore) { members[memberLocation]->addProductToCart(pID); } // Check to see if the customer is a member else if (!inMember) { std::cout << "Sorry you are not a member." << std::endl; } // Check to see if the item is actually in inventory else if (!inStore) { std::cout << "Sorry, the item you are looking for is not in our store" << std::endl; } // Check to see if they are a member, the item is in store, and that the item is out of stock else if (!inStock) { std::cout << "Sorry, product #" << inventory[itemLocation]->getIdCode() << " is currently out of stock." << std::endl; } // Just a fallback if it misses everything...somehow else { std::cout << "Sorry, an error has occurred, try again." << std::endl; }
}
/************************************************************** * Store::checkOutMember * Description: If the member isn't found in the members, print * "Member #[accountID goes here] not found." Otherwise prints * out the title and price for each product in the cart and * decreases the available quantity of that product by 1. If * any product has already sold out, then on that line it * should print 'Sorry, product #[idCode goes here], "[product * name goes here]", is no longer available.' At the bottom it * should print out the subtotal for the cart, the shipping * cost ($0 for premium members, 7% of the cart cost for * normal members), and the final total cost for the cart * (subtotal plus shipping). If the cart is empty, it should * just print "There are no items in the cart." When the * calculations are complete, the member's cart should be * emptied. **************************************************************/ void Store::checkOutMember(std::string mID) { // Variables needed for function functionality // Amount of members int memberSize = members.size(); // Found the Member bool exists = false; // Variable to hold the total cart value double totalCost = 0; // Variable to hold the end cost of shipping double shipping = 0;
// Loop through members to see if the inputted member is in it for (int i = 0; i < memberSize; i++) { // If the member in the store matches the current member search mark as true. if (members[i]->getAccountID() == mID) { exists = true;
// Pull the size of that current users cart double cartSize = members[i]->getCart().size();
// If there are no items in the cart, let the user know if (cartSize == 0) { std::cout << "There are no items in the cart." << std::endl; } else { // Select each cart item for (int j = 0; j < cartSize; j++) { // Search through entire inventory for matching item for (int k = 0; k < inventory.size(); k++) { // When we find a matching item... if (inventory.at(k)->getIdCode() == members[i]->getCart().at(j)) { // Check to see if it is in stock, if not let customer know if (inventory.at(k)->getQuantityAvailable() < 1) { std::cout << "Sorry, product #" << inventory.at(k)->getIdCode() << ", " << inventory.at(k)->getTitle() << " is no longer available" << std::endl; } // Otherwise print out name and price and add it to total else { std::cout << inventory.at(k)->getTitle() << " - $" << inventory.at(k)->getPrice() << std::endl;
// Add price to total and decrease quantity totalCost += inventory.at(k)->getPrice(); inventory.at(k)->decreaseQuantity(); } } } } // Check to see if the user is a premium member and link respective shipping costs if (members[i]->isPremiumMember()) { shipping = 0; } else { shipping = totalCost * 0.07; }
// Print out the subtotal and shipping for the user std::cout << "Subtotal: $" << totalCost << std::endl; std::cout << "Shipping: $" << shipping << std::endl; std::cout << "Total: $" << totalCost + shipping << std::endl;
// Empty the cart once done members[i]->emptyCart(); } } } // If the member does not exist, then let the user know they couldn't be found if (!exists) { std::cout << "Member #" << mID << " not found." << std::endl; } }
Here are the .hpp files:
Product.hpp
#ifndef
PRODUCT_HPP
#define
PRODUCT_HPP
#include
class
Product
{
private:
std::string
idCode;
std::string
title;
std::string
description;
double
price;
int
quantityAvailable;
public:
Product(std::string
id,
std::string
t, std::string d,
double
p, int
qa);
std::string
getIdCode();
std::string
getTitle();
std::string
getDescription();
double
getPrice();
int
getQuantityAvailable();
void
decreaseQuantity();
};
#endif
Customer.hpp
#ifndef
CUSTOMER_HPP
#define
CUSTOMER_HPP
#include
#include
"Product.hpp"
class
Customer
{
private:
std::vector
cart;
std::string
name;
std::string
accountID;
bool
premiumMember;
public:
Customer(std::string
n, std::string
a, bool
pm);
std::string
getAccountID();
std::vector
getCart();
void
addProductToCart(std::string);
bool
isPremiumMember();
void
emptyCart();
};
#endif
Store.hpp
#ifndef
STORE_HPP
#define
STORE_HPP
#include
#include
"Customer.hpp"
class
Store
{
private:
std::vector
inventory;
std::vector
members;
public:
void
addProduct(Product*
p);
void
addMember(Customer*
c);
Product*
getProductFromID(std::string);
Customer*
getMemberFromID(std::string);
void
productSearch(std::string
str);
void
addProductToMemberCart(std::string
pID,
std::string
mID);
void
checkOutMember(std::string
mID);
};
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
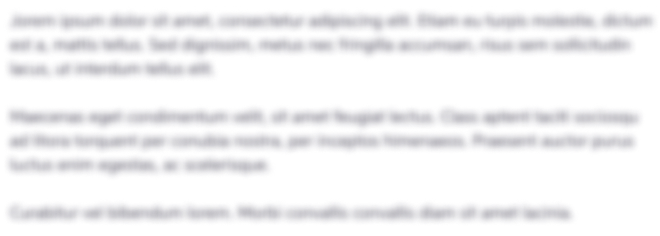
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started