Question
When I print out my project its showing null in the first and last name and not updating my hours when I changed them, why?
When I print out my project its showing null in the first and last name and not updating my hours when I changed them, why? public class ManageVolunteers {
public static void main(String[] arg) {// main method Scanner keyboard = new Scanner(System.in); System.out.print("Enter volunteer First Name: "); String firstName = keyboard.nextLine(); System.out.print("Enter volunteer Last Name: "); String lastName = keyboard.nextLine(); System.out.print("Enter the start date: (mm/dd/yyy)"); String startDate = keyboard.nextLine(); System.out.println(startDate); System.out.println("volunteer 1"); System.out.print("Enter Volunteer Hours: "); double volunteerHours = keyboard.nextDouble(); Volunteer vol1 = new Volunteer(firstName, lastName, startDate, volunteerHours); System.out.println(vol1); System.out.println("volunteer 2"); System.out.println("Enter Volunteer Hours: "); double volunteerHours2 = keyboard.nextDouble(); Volunteer vol2 = new Volunteer(firstName, lastName, startDate, volunteerHours); System.out.println(vol2); } }
public class Volunteer {
private String firstName; private String lastName; private LocalDate startDate; private double volunteerHours;
public static final String DEFAULT_FIRST_NAME = "first name not assigned"; public static final String DEFAULT_LAST_NAME = "last name not assigned"; public static final LocalDate DEFAULT_START_DATE = LocalDate.now(); public static final double DEFAULT_HOURS = 0;
public Volunteer(String firstName, String lastName, LocalDate startDate, double volunteerHours) { this.firstName = firstName; this.lastName = lastName; this.startDate = startDate; this.volunteerHours = volunteerHours; }
public Volunteer(String firstName, String lastName, String startDate, double volunteerHours) { }
public String getFirstName() { return firstName; }
public void setFirstName(String firstName) { if (firstName != null && firstName.length() > 0) this.firstName = firstName; }
public String getLastName() { return lastName; }
public void setLastname(String lastName) { if (lastName != null && lastName.length() > 0) this.lastName = lastName; }
public LocalDate getStartDate() { return startDate; }
public void setStartDate(LocalDate startDate) { if (startDate != null) this.startDate = startDate; }
public void setStartDate(int newYear, int newMonth, int newDay) { this.setStartDate(newYear, newMonth, newDay); }
public double getVolunteerHours(double volunteerHours) { return volunteerHours; }
public void setVolunteerHours(double volunteerHours) { this.volunteerHours = volunteerHours; }
public void updateVolunteerHours(double updateVolunteerHours) { double updateVolunteerHours = 0; this.volunteerHours = (this.volunteerHours + updateVolunteerHours); }
public static String getDefaultFirstName() { return DEFAULT_FIRST_NAME; }
public static String getDefaultLastName() { return DEFAULT_LAST_NAME; }
public static LocalDate getDefaultStartDate() { return DEFAULT_START_DATE; }
public static double getDefaultHours() { return DEFAULT_HOURS; }
@Override public String toString() { return "Volunteers [firstName = " + firstName + ", lastName = " + lastName + ", startDate = " + startDate + ", volunteerHours = " + volunteerHours + "]"; }
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
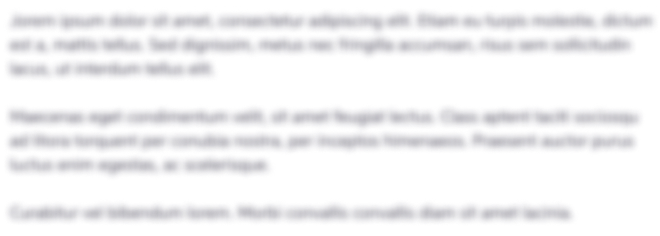
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started