Question
When the next move X wants to make is invalid. Its turn is skipped. Please fix code! I have two source files import java.util.Arrays; import
When the next move X wants to make is invalid. Its turn is skipped. Please fix code! I have two source files
import java.util.Arrays;
import java.util.HashMap;
import java.util.Random;
import java.util.Scanner;
/**
* This class creates an AI TicTacToe game
*
* @author Morgan
*
*/
public class TicTacToe {
private char[][] gameBoard;
private boolean gameOngoing = true;
private HashMap
/**
* This is the constructor for the GameBoard class
*/
public TicTacToe() {
gameBoard = new char[3][3];
dictionary = new HashMap
for (int row = 0; row < gameBoard.length; row++) {
Arrays.fill(gameBoard[row], ' ');
}
} // end of constructor
public void startGame() {
generateBoard("---------", 9, 'X');
int counter = 0;
int emptySpaces = 9;
while (gameActive() && counter < 10) {
if (counter % 2 == 0) {
askPlayer('O');
} else {
System.out.println(" Computers turn... ");
String boardStr = convertBoardToString();
int bestMove = getBestMove(boardStr);
int[] rowcol = new int[2];
rowcol = convertCoordinateToDouble(bestMove);
int row = rowcol[0];
int col = rowcol[1];
boolean move = makeMove('X', row, col);
while (move == false) {
generateBoard(boardStr, emptySpaces, 'X');
int[] rowcol1 = new int[2];
rowcol = convertCoordinateToDouble(bestMove);
int row1 = rowcol[0];
int col1 = rowcol[1];
move = makeMove('X', row1, col1);
}
emptySpaces--;
}
++counter;
System.out.println();
displayBoard();
checkForWinner();
if (counter == 10) {
System.out.println("Stale mate! ");
}
}
}
/**
* This method will display the gameBoard to the screen
*/
public void displayBoard() {
for (int row = 0; row < gameBoard.length; row++) {
for (int col = 0; col < gameBoard[0].length; col++) {
System.out.print("\t" + gameBoard[row][col] + "\t");
if (col == 0 || col == 1) {
System.out.print("|");
}
if ((row == 0 && col == 2) || (row == 1 && col == 2)) {
System.out.print(" ------------------------------------------------- ");
}
}
}
System.out.println();
System.out.println();
System.out.println();
} // end displayBoard method
/**
* This method will validate if a players move is allowed and return true if the
* move was completed
*/
public boolean makeMove(char player, int row, int col) {
if (row >= 0 && row <= 2 && col >= 0 && col <= 2) {
if (gameBoard[row][col] != ' ') {
return false;
} else {
gameBoard[row][col] = player;
return true;
}
} else {
return false;
}
} // end of makeMove method
/**
* Will return true if the game is still active
*/
public boolean gameActive() {
return gameOngoing;
} // end of gameActive method
/**
* This method will ask the user to pick a row and column, validate the inputs
* and call the method makeMove()
*/
public void askPlayer(char player) {
Scanner sc = new Scanner(System.in);
int row, col;
do {
System.out.printf("Player %s please enter a row (1-3): ", player);
row = sc.nextInt();
System.out.printf("Player %s Please enter a column (1-3): ", player);
col = sc.nextInt();
} while (notValid(row, col));
makeMove(player, row - 1, col - 1);
} // end of askPlayer method
// Getters for gameBoard and gameOngoing
public char[][] getGameBoard() {
return gameBoard;
}
/**
* This method will validate if the row and column are between 1-3 and if the
* position is currently empty
*/
public boolean notValid(int row, int col) {
if (row > 3 || row < 1 || col > 3 || col < 1 || !isEmpty(row, col)) {
return true;
} else {
return false;
}
} // end notValid method
/**
* This method will check if a position is empty
*
* @return true if the position is empty, false otherwise
*/
public boolean isEmpty(int row, int col) {
if (gameBoard[row - 1][col - 1] == ' ') {
return true;
} else {
System.out.println("That postion is taken.");
return false;
}
}// end isEmpty method
/**
* This method will check to see if there are 3 X's or O's in a row
*
* @return true if there is a winner, false otherwise
*/
public boolean checkForWinner() {
for (int row = 0; row < gameBoard.length; row++) {
// checking horizontal
if (gameBoard[row][0] == gameBoard[row][1] && gameBoard[row][1] == gameBoard[row][2]
&& gameBoard[row][0] != ' ') {
System.out.println("The winner is " + gameBoard[row][0]);
gameOngoing = false;
}
}
for (int col = 0; col < gameBoard[0].length; col++) {
// checking vertical
if (gameBoard[0][col] == gameBoard[1][col] && gameBoard[1][col] == gameBoard[2][col]
&& gameBoard[0][col] != ' ') {
System.out.println("The winner is " + gameBoard[0][col]);
gameOngoing = false;
}
}
if (gameBoard[0][0] == gameBoard[1][1] && gameBoard[1][1] == gameBoard[2][2] && gameBoard[0][0] != ' ') {
System.out.println("The winner is " + gameBoard[0][0]);
gameOngoing = false;
}
if (gameBoard[2][0] == gameBoard[1][1] && gameBoard[1][1] == gameBoard[0][2] && gameBoard[0][2] != ' ') {
System.out.println("The winner is " + gameBoard[2][0]);
gameOngoing = false;
}
return false;
} // end checkForWinner method
/**
* Checks status of game
*
* @return if the game continues
*/
public boolean isGameOngoing() {
return gameOngoing;
}
/**
* Turns the gameBoard into a string
*/
@Override
public String toString() {
String str = "";
for (int row = 0; row < gameBoard.length; row++) {
for (int col = 0; col < gameBoard[0].length; col++) {
str = str + gameBoard[row][col];
}
}
return str;
} // end toString method
/**
* Converts board of char[][] to a string
*/
public String convertBoardToString() {
String strBoard = "";
for (int row = 0; row < gameBoard.length; row++) {
for (int col = 0; col < gameBoard[0].length; col++) {
strBoard = strBoard + gameBoard[row][col];
}
}
strBoard = strBoard.replace(' ', '-');
System.out.println(strBoard);
return strBoard;
}
/**
* This method converts coordinates to a single digit
*/
public int convertCoordinateToSingle(int row, int col) {
if (row == 1 && col == 1) {
return 0;
}
if (row == 1 && col == 2) {
return 1;
}
if (row == 1 && col == 3) {
return 2;
}
if (row == 2 && col == 1) {
return 3;
}
if (row == 2 && col == 2) {
return 4;
}
if (row == 2 && col == 3) {
return 5;
}
if (row == 3 && col == 1) {
return 6;
}
if (row == 3 && col == 2) {
return 7;
}
if (row == 3 && col == 3) {
return 8;
}
return 0;
} // end convertCoordinatesToSingle method
/**
* This method converts coordinates to a row and col
*/
public int[] convertCoordinateToDouble(int dig) {
int[] rowcol = new int[2];
if (dig == 0) {
rowcol[0] = 1;
rowcol[1] = 1;
return rowcol;
}
if (dig == 1) {
rowcol[0] = 1;
rowcol[1] = 2;
return rowcol;
}
if (dig == 2) {
rowcol[0] = 1;
rowcol[1] = 3;
return rowcol;
}
if (dig == 3) {
rowcol[0] = 2;
rowcol[1] = 1;
return rowcol;
}
if (dig == 4) {
rowcol[0] = 2;
rowcol[1] = 2;
return rowcol;
}
if (dig == 5) {
rowcol[0] = 2;
rowcol[1] = 3;
return rowcol;
}
if (dig == 6) {
rowcol[0] = 3;
rowcol[1] = 1;
return rowcol;
}
if (dig == 7) {
rowcol[0] = 3;
rowcol[1] = 2;
return rowcol;
}
if (dig == 8) {
rowcol[0] = 3;
rowcol[1] = 3;
return rowcol;
}
return null;
} // end convertCoordinatesToDouble method
public HashMap
return dictionary;
}
/**
* Generates all possible TicTacToe boards that are valid
*/
public void generateBoard(String board, int emptySpaces, char currentPlayer) {
if (emptySpaces == 0) {
return;
}
for (int i = 0; i < 9; i++) {
if (board.charAt(i) == '-') {
String newBoard = board.substring(0, i) + currentPlayer + board.substring(i + 1);
// System.out.println(newBoard);
// System.out.println(getBestMove(newBoard));
dictionary.put(newBoard, getBestMove(newBoard));
generateBoard(newBoard, emptySpaces - 1, determineTurn(currentPlayer));
newBoard = board.substring(0, i) + '-' + board.substring(i + 1);
}
}
} // end generateBoard method
/**
* Takes in a string and returns an array representation
*/
public Character[] convertStringToArrayRep(String board) {
Character[] arrayBoard = new Character[9];
for (int i = 0; i < board.length(); i++) {
arrayBoard[i] = board.charAt(i);
}
return arrayBoard;
} // end convertStringToArrayRep method
public int getBestMove(String board) {
for (int i = 0; i < board.length(); i++) {
if (board.charAt(i) == '-') {
String nextMoveBoardX = board.substring(0, i) + 'X' + board.substring(i + 1);
if (winExists(nextMoveBoardX) == 'X') {
return i;
}
}
}
for (int i = 0; i < board.length(); i++) {
if (board.charAt(i) == '-') {
String nextMoveBoardO = board.substring(0, i) + 'O' + board.substring(i + 1);
if (winExists(nextMoveBoardO) == 'O') {
return i;
}
}
}
for (int i = 0; i < board.length(); i++) {
if (board.charAt(i) == '-') {
return i;
}
}
Random rand = new Random();
int n = rand.nextInt(8) + 0;
return n;
} // end getBestMove method
/**
* Determines if there is a win within the string
*
* @param board
* @return
*/
private char winExists(String board) {
char winner = ' ';
String firstRow = board.substring(0, 3);
String secondRow = board.substring(3, 6);
String thirdRow = board.substring(6, 9);
// Check first row
if (firstRow.charAt(0) == firstRow.charAt(1) && firstRow.charAt(2) == firstRow.charAt(0)
&& firstRow.charAt(0) != '-') {
winner = firstRow.charAt(0);
}
// Check second row
if (secondRow.charAt(0) == secondRow.charAt(1) && secondRow.charAt(2) == secondRow.charAt(0)
&& secondRow.charAt(0) != '-') {
winner = secondRow.charAt(0);
}
// Check third row
if (thirdRow.charAt(0) == thirdRow.charAt(1) && thirdRow.charAt(2) == thirdRow.charAt(0)
&& thirdRow.charAt(0) != '-') {
winner = thirdRow.charAt(0);
}
// Check diagonal
if (firstRow.charAt(0) == secondRow.charAt(1) && firstRow.charAt(0) == thirdRow.charAt(2)
&& firstRow.charAt(0) != '-') {
winner = firstRow.charAt(0);
}
// Check opposite diagonal
if (firstRow.charAt(2) == secondRow.charAt(1) && firstRow.charAt(2) == thirdRow.charAt(0)
&& firstRow.charAt(2) != '-') {
winner = firstRow.charAt(2);
}
return winner;
} // end winExists method
/**
* Determines whos turn it is
*
* @param currentPlayer
* @return
*/
private char determineTurn(char currentPlayer) {
if (currentPlayer == 'X') {
return 'O';
} else {
return 'X';
}
} // end determineTurn method
} // end class
XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
/**
* This class starts a TicTacToe game
*
* @author Morgan
*
*/
public class TicTacToeInteraction {
public static void main(String[] args) {
TicTacToe myGame = new TicTacToe();
myGame.startGame();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
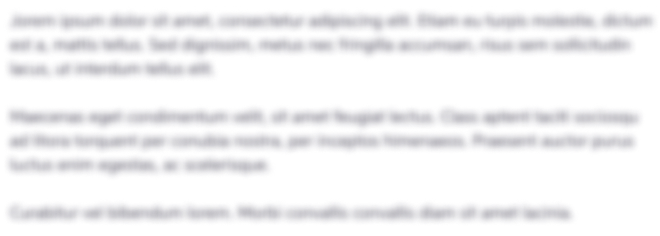
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started