Question
Which of the following patterns can be used for designing your class to add error checking such as not allowing a blank name for a
Which of the following patterns can be used for designing your class to add error checking such as not allowing a blank name for a bank account?
Question options:
Describing the Position of an Object | |||
Modeling Objects with Distinct States | |||
Managing Properties of an Object | |||
Collecting Values | |||
Question 4 | 0 / 1 point | ||
Consider the following class which is used to represent a polygon consisting of an arbitrary number of (x, y) points:
class Polygon :
def __init__(self) :
self._x_points = []
self._y_points = []
Which of the following code segments is the correct implementation for the addPoint method that adds another point to the polygon?
Question options:
def addPoint(self, x, y) : self._points.append(x, y) | |||
def addPoint(self, x, y) : self._x_points.append(x) self._y_points.append(y) | |||
def addPoint(self, x, y) : self._x_points = x self._y_points = y | |||
def addPoint(self, x, y) : self._x_points = [x] self._y_points = [y] | |||
Question 6 | 0 / 1 point | ||
Which of the following method headers represent a constructor for an Employee class?
Question options:
def Employee(self) : | |||
def __init()__ : | |||
def __Employee__(self) : | |||
def __init__(self) : | |||
Question 7 | 0 / 1 point | ||
What part of the virtual machine is responsible for removing objects that are no longer referenced?
Question options:
The allocator | |||
The garbage collector | |||
The recycler | |||
The regenerator | |||
Question 11 | 0 / 1 point | ||
Which of the following is NOT a true statement regarding object-oriented programming?
Question options:
Object oriented programming views the program as a list of actions to perform. | |||
Object oriented programs usually contain different types of objects, each corresponding to a particular kind of complex data, real-world object or concept. | |||
Object oriented programming is a style where tasks are solved by collaborating objects. | |||
Object oriented programs are organized around "objects" rather than "actions" and "data" rather than "logic". | |||
Question 13 | 0 / 1 point | ||
Given the following code snippet, what statement completes the code to add several items to one grocery list:
def addItems(self, price, quantity, itemName) :
for i in range(quantity) :
________________________
def addItem(self, price, itemName) :
self._itemCount = self._ItemCount + 1
self._totalPrice = self._totalPrice + price
. . .
Question options:
self.addItem(price, itemName) | |||
addItem(price, itemName) | |||
self._addItem(price, itemName) | |||
_addItem(price, itemName) | |||
Question 17 | 0 / 1 point | ||
What is the name of the method in the following code segment?
class Fruit :
def getColor(self) :
return self._color
Question options:
_color | |||
Fruit | |||
getColor | |||
self | |||
Question 18 | 0 / 1 point | ||
Consider the following class:
class Counter :
def getValue(self) :
return self._value
def click(self) :
self._value = self._value + 1
def unClick(self) :
self._value = self._value - 1
def reset(self) :
self._value = 0
Which method creates a new instance variable?
Question options:
click | |||
getValue | |||
reset | |||
unClick | |||
Question 19 | 0 / 1 point | ||
What change needs to be made in the following code segment so that lName will have a default value of "unknown"?
class PhoneNumber :
def __init__(self, lName, phone = "215-555-1212") :
self._name = lName
self._phone = phone
Question options:
Replace self._name = lName with self._name = "unknown" | |||
Replace the lName parameter with lName = "unknown" | |||
Add name = "unknown" to the end of the constructor | |||
Add self._lName = "unknown" to the end of the constructor | |||
Question 20 | 0 / 1 point | ||
Suppose you have a class ShoppingList, with instance variables _quantity, _cost, and _itemName. How should you access these variables when writing code that is not contained within the ShoppingList class?
Question options:
Directly access the instance variables by using the object and the instance variables' names. | |
Use methods provided by the ShoppingList class. | |
It is not possible to access the instance variables outside of the ShoppingList class. | |
Use the self parameter followed by the instance variables' names. |
Given the variable assignment sentence = "", what is the value of len(sentence)?
Question options:
1 | |||
-1 | |||
an exception is raised | |||
0 | |||
Question 13 | 0 / 1 point | ||
Recall the Cash Register class developed in the textbook. What is output by the following code segment?
from cashregister2 import CashRegister
reg1 = CashRegister()
reg2 = reg1
reg1.addItem(3.00)
reg1.addItem(5.00)
reg2.clear()
print(reg1.getCount())
Question options:
0 | |||
1 | |||
2 | |||
The program terminates with a runtime error | |||
Question 16 | 0 / 1 point | ||
What happens when an object is no longer referenced?
Question options:
Nothing until the problem terminates | |||
The garbage collector removes the object | |||
The address is saved for future instances of the object | |||
Creates a None reference | |||
Question 18 | 0 / 1 point | ||
Which statement is NOT a description of encapsulation?
Question options:
The act of hiding the implementation details | |
A collection of methods through which the objects of the class can be manipulated | |
Enables changes in the implementation without affecting users of a class | |
Mechanism for restricting access to some of the object's components |
Which of the follow code segments could be used to help test the getColor method from the Fruit class?
Question options:
print(f.getColor()) print("Expected: Yellow") | |||
print("Yellow") print("Expected: Yellow") | |||
print(f.getColor()) print("Expected:", f.getColor()) | |||
print("Yellow") print("Expected:", f.getColor()) | |||
Question 2 | 0 / 1 point | ||
Which of the following patterns can be used for designing your class to update the balance of a bank account?
Question options:
Keeping a total | |||
Counting events | |||
Collecting values | |||
Managing properties of an object | |||
Question 3 | 0 / 1 point | ||
Consider a class that represents a hardware device. The device can be in one of two states: Plugged in, or unplugged. Which of the following class definitions is best for this situation?
Question options:
PLUGGED_IN = 0 UNPLUGGED = 1 class Device : def __init__(self) : . . . | |||
class Device : PLUGGED_IN = 0 UNPLUGGED = 1 def __init__(self) : . . . | |||
class Device : def __init__(self) : PLUGGED_IN = 0 UNPLUGGED = 1 . . . | |||
class Device : def __init__(self) : self.PLUGGED_IN = 0 self.UNPLUGGED = 1 . . . | |||
Question 6 | 0 / 1 point | ||
Which of the following is NOT a pattern used to help design the data representation of a class?
Question options:
An instance variable for thte total is updated in methods that increase or decrease the total amount | |||
An object reference specifies the location of an object | |||
An object can collect other objects in a list | |||
To model a moving object, you need to store and update its position | |||
Question 9 | 0 / 1 point | ||
Consider the following class:
class Contact :
def __init__(self, name, phone="")
self._name = name
self._phone = phone
def getName(self) :
return self._name
def setName(self, new_name) :
self._name = new_name
def getPhone(self) :
return self._phone
def setPhone(self, new_phone) :
self._phone = new_phone
What is output by the following code segment?
p1 = Contact("Bob", "555-123-4567")
p2 = Contact("Joe", "555-000-0000")
p1.setName(p2.getName())
print(p1.getPhone())
Question options:
Bob | |||
Joe | |||
555-000-0000 | |||
555-123-4567 | |||
Question 10 | 0 / 1 point | ||
How many constructors can be defined for each class?
Question options:
At least one must be defined | |||
Only one may be defined | |||
At least one, but as many as needed | |||
At least one, but no more than five | |||
Question 11 | 0 / 1 point | ||
Which of the following is NOT true about instance methods for a class?
Question options:
The object on which a method is applied is automatically passed to the self parameter variable of the method | |||
In a method, you access instance variables through the self parameter variable | |||
A class variable belongs to the class, not to any instance of the class | |||
The accessor and mutator methods are automatically called when an object is created | |||
Question 13 | 0 / 1 point | ||
What is the value of the variable bankAcct after the following code snippet is executed?
bankAcct = BankAccount("Fisher", 1000.00)
Question options:
"Fisher", 1000.00 | |||
A memory location | |||
"Fisher" | |||
1000.00 | |||
Question 18 | 0 / 1 point | ||
Consider the following class:
class Pet:
____________________
def __init__(self, name):
self._name = name
Pet._lastID = Pet._lastID + 1
self._registrationID = Pet._lastID
What line of code should be placed in the blank to create a class variable that keeps track of the most recently used registration identifier?
Question options:
_lastID = 0 | |
Pet.append(_lastID) | |
Pet._lastID = 0 | |
self._lastID = 0 |
Attempt Score: | 6 / 10 - D |
8 / 10 - B |
Question 1 | 0 / 1 point |
Before invoking a method on an object, what must be done first?
Question options:
Initialize all instance variables | ||
Invoke the accessor and mutator methods | ||
Create a public interface | ||
Construct the object | ||
Question 6 | 0 / 1 point | |
Which of the following questions should you ask yourself in order to determine if you have named your class properly?
Question options:
Does the class name contain 8 or fewer characters? | ||
Is the class name a verb? | ||
Can I visualize an object of the class? | ||
Does the class name describe the tasks that this class will accomplish? | ||
Question 8 | 0 / 1 point | |
Consider a class that represents a hardware device. The device can be in one of two states: Plugged in, or unplugged. Which of the following class definitions is best for this situation?
Question options:
PLUGGED_IN = 0 UNPLUGGED = 1 class Device : def __init__(self) : . . . | ||
class Device : PLUGGED_IN = 0 UNPLUGGED = 1 def __init__(self) : . . . | ||
class Device : def __init__(self) : PLUGGED_IN = 0 UNPLUGGED = 1 . . . | ||
class Device : def __init__(self) : self.PLUGGED_IN = 0 self.UNPLUGGED = 1 . . . | ||
Question 9 | 0 / 1 point | |
Which of the following is considered by the text to be the most important consideration when designing a class?
Question options:
Each class should represent an appropriate mathematical concept. | ||
Each class should represent a single concept or object from the problem domain. | ||
Each class should represent no more than three specific concepts. | ||
Each class should represent multiple concepts only if they are closely related. | ||
Question 16 | 0 / 1 point | |
Which name would be best for a private instance variable?
Question options:
_confidential | ||
HIDDEN | ||
private | ||
Secret | ||
Question 17 | 0 / 1 point | |
A method that queries an object for some information without changing it is known as a(n):
Question options:
Accessor | ||
Function | ||
Interface | ||
Mutator | ||
Question 19 | 0 / 1 point | |
Which of the following method headers represent a constructor for an Employee class?
Question options:
def Employee(self) : | |
def __init()__ : | |
def __Employee__(self) : | |
def __init__(self) : |
Question 2 | 0 / 1 point |
What items should be considered when creating the public interface?
Question options:
Method headers and method comments | ||
Instance variables | ||
Data and method bodies | ||
Method implementations | ||
Question 3 | 0 / 1 point | |
In the following example, which data is considered instance data?
You are assigned the task of writing a program that calculates payroll for a small company. To get started the program should do the following:
Add new employees including their first and last name and hourly wage
Ask for the number of hours worked
Calculate payroll (applying 1.5 for any hours greater than 40)
Print a report of all employees' salary for the week, total of all hours and total of all salaries
Question options:
firstName, lastName, hoursWorked, hourlyWage | ||
firstName, lastName, hoursWorked, hourlyWage, payrollAmount | ||
firstName, lastName, hoursWorked, hourlyWage, totalHours, totalSalary | ||
firstName, lastName, hoursWorked, hourlyWage, payrollAmount, totalHours, totalSalary | ||
Question 4 | 0 / 1 point |
Which of the following is NOT a step carried out by a tester program?
Question options:
Construct one or more objects of the class | ||
Invoke one or more methods | ||
Print out one or more results | ||
Identify syntax errors | ||
Question 16 | 0 / 1 point | |
What change needs to be made in the following code segment so that lName will have a default value of "unknown"?
class PhoneNumber :
def __init__(self, lName, phone = "215-555-1212") :
self._name = lName
self._phone = phone
Question options:
Replace self._name = lName with self._name = "unknown" | ||
Replace the lName parameter with lName = "unknown" | ||
Add name = "unknown" to the end of the constructor | ||
Add self._lName = "unknown" to the end of the constructor | ||
Question 20 | 0 / 1 point | |
Which of the following is NOT a difference between methods and functions?
Question options:
A method is defined within a class. A function is defined outside of a class. |
|
A function is defined within a class. A method is defined outside of a class. |
|
The first parameter variable for a method is self. Functions do not have a self parameter variable. |
|
A method can access the instance variables of an object. A function cannot. |
Question 1 | 0 / 1 point |
Assume a class exists named Fruit. Which of the follow statements constructs an object of the Fruit class?
Question options:
def Fruit() : | ||
class Fruit() : | ||
x = Fruit() | ||
x = Fruit.create() | ||
Question 5 | 0 / 1 point | |
Assume that x is a variable containing an object reference. Which special method is invoked when the following statement executes?
print(x)
Question options:
__init__ | ||
__print__ | ||
__repr__ | ||
__str__ | ||
Question 6 | 0 / 1 point | |
How do you access instance variables in a method?
Question options:
Using the constructor | ||
Using the public interface | ||
Using a default value | ||
Using the self reference | ||
Question 7 | 0 / 1 point | |
Consider the following code segment:
class PhoneNumber :
def __init__(self, lName, phone = "215-555-1212") :
self._name = lName
self._phone = phone
def getName(self):
return self._name
def getPhone(self):
return self._phone
Jones = PhoneNumber("Jones")
print(Jones.getName(), Jones.getPhone())
What will be printed when it executes?
Question options:
| Jones 215-555-1212 | |
| AttributeError: 'PhoneNumber' object has no attribute | |
| Jones | |
| Jones 000-000-0000 | |
Question 8 | 0 / 1 point |
What is the purpose of an object's instance variables?
Question options:
| Store the data required for executing its methods | |
| Store the data for all objects created by the same class | |
| To provide access to the data of an object | |
| To create variables with public visibility | |
Question 11 | 0 / 1 point |
Consider the following code segment:
def mutate(self, newType) :
self._type = newType
self._mutations = self._mutations + 1
What is the name of the local variable in it:
Question options:
mutate | ||
_mutations | ||
newType | ||
_type | ||
Question 13 | 0 / 1 point | |
When is a constructor invoked?
Question options:
When an object is created | ||
When instance data is modified | ||
When a method is invoked | ||
When multiple arguments are required to create an object | ||
Question 15 | 0 / 1 point |
Which of the following statements determines if x currently refers to an object containing an integer?
Question options:
if int(x) : | ||
if x == int : | ||
if x is int : | ||
if isinstance(x, int) : | ||
Question 20 | 0 / 1 point | |
Assume a class exists named Person. Which of the follow statements constructs an object of the Person class?
Question options:
bob = __init__(Person) | |
bob = Person.__init__() | |
bob = Person | |
bob = Person() |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
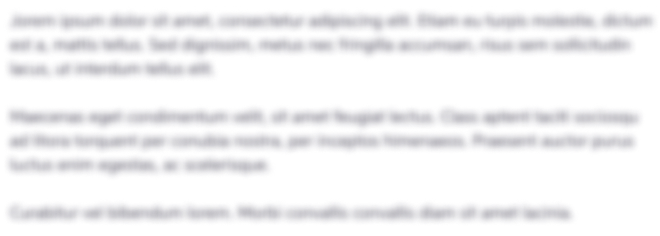
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started