Question
Windows Form APP (I need help with putting it in Windows App Form) This should be the Output: C# Create an application that will allow
Windows Form APP (I need help with putting it in Windows App Form) This should be the Output:
C# Create an application that will allow a loan amount, interest rate, and number of finance years to be entered for a given loan. Determine the monthly payment amount. Calculate how much interest will be paid over the life of the loan. Display an amortization schedule showing the new balance after each payment is made. Design an object - oriented solution. Use two classes. Loan class: characteristics such as the amount to be financed, rate of interest, period of time for the loan, an d total interest paid will identify the current state of a loan object. Include methods to determine the monthly payment amount, return the total interest paid over the life of the loan, and Loan table function to display an amortization schedule that include: number of the cycle, payment amount, principal paid, interest paid, total interest paid, balance LoanTest class: In the m ain class: instantiate an objec t of the loan class. Allow the user to input data about the loan . T hen use Loan t able function to display the Loan information Monthly Payment Formula: get monthly payment A from Loan Amount P , Interest Rate i , and Loan Period n
My Code:
LoanTest.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LoanApplication
{
//Defien the class.
class LoanTest
{
//Define the main method.
static void Main(string[] args)
{
//Declare the variables
double amount;
double rate;
int years;
//prompt the user to enter the loan details.
Console.WriteLine("Enter loan amount");
amount = Convert.ToDouble(Console.ReadLine());
Console.WriteLine("Enter annual interest rate");
rate = Convert.ToDouble(Console.ReadLine());
Console.WriteLine("Enter number of years");
years = Convert.ToInt32(Console.ReadLine());
Loan loanObj = new Loan(amount, rate, years);
//Call the method to display the details.
loanObj.loanTable();
Console.ReadKey();
}
}
}
Loan.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LoanApplication
{
//Create the loan class.
class Loan
{
//Declare the variables
private double p;
private double i;
private int n;
//Define the constructor.
public Loan(double amount, double rate, int years)
{
this.p = amount;
this.i = (rate/100.0)/12.0;
this.n = years;
}
//Define the method to calculate the
//Monthly payment.
public double getMonthlyPayment()
{
int months = n * 12;
return (p * i * Math.Pow(1 + i, months)) /
(Math.Pow(1 + i, months) - 1);
}
//Define the method to calculate the
//total interest paid
public double totalInterestPaid()
{
//int months = n * 12;
double m_p = getMonthlyPayment();
double p_paid = 0;
double totalInt=0;
for (int months = 1; months
{
double interest = p * i;
p_paid = m_p-interest;
totalInt += interest;
p = p-p_paid;
}
return totalInt;
}
//Define the method to prints the
//amortization schedule of Loan
public void loanTable()
{
//Define the variables.
double princiPaid = 0;
double newBal = 0;
double intPaid = 0;
double princi = p;
double ti=0;
//Get the monthly payment in the variable.
double monthlyPayment = getMonthlyPayment();
//Display the amortization schedule headings.
Console.WriteLine("{0,-10}{1,-10}{2,-10}{3,-10}{4,-10}",
"Month\t", "payment amount\t", "interest paid\t",
"principal paid\t", "Balance");
//Calculate the amount paid and new balance
// and display the details in form
// of amortization schedule table.
for (int months = 1; months
{
intPaid = princi * i;
princiPaid = monthlyPayment - intPaid;
newBal = princi - princiPaid;
ti = ti+ intPaid;
Console.WriteLine(
"{0,-7}\t\t{1,10:N2}\t{2,10:N2}\t{3,10:N2}\t{4,10:N2}",
months,monthlyPayment, intPaid,princiPaid, newBal);
//Update balance as new balance.
princi = newBal;
}
//Dispaly the total interest paid.
Console.WriteLine(" Total interest paid:{0}",
totalInterestPaid().ToString ("#.##"));
}
}
}
Loan Data Input Month rincipal Int/Emt Prn/Ent $99.55 2 99, 800. 40 499. 50 100. 05 3 99, 699 , 85 499. 00 100. 55 4 99, 598 . 80 498. 60 101 . 05 5 99,497.24 $497.99 101.56 E $99, 395 , 18 497. 49 $102. 06 7 +99, 292. 49E.9 102.57 8 99,189.52 496.4 $103.09 9 99,085.92 495.95 103.60 10 98,981.79 435.43 104.12 11 $98,877.15 494.91 $104.64 12 98,771. 99 494 . 39 106. 16 13 98, 666 . 30 493. 86 106. 69 14 98,560.08 493.33 106.22 15 $98,453.33 492.80 $106.75 IE 98, 346.04 $492.27 $107.28 17 98,238.22 491.73 107.82 18 $98, 129.8 491.19 $108.36 1 $99,900.45 500.00 100000 Loan Amount Tem in Years (1 to 50) APR percent Monthly Payment Total Money Paid $599.55 S215,838.19 Clear Amortize 900000000000000000 050694949 93838271 099999999999999999 + co co co co co Loan Data Input Month rincipal Int/Emt Prn/Ent $99.55 2 99, 800. 40 499. 50 100. 05 3 99, 699 , 85 499. 00 100. 55 4 99, 598 . 80 498. 60 101 . 05 5 99,497.24 $497.99 101.56 E $99, 395 , 18 497. 49 $102. 06 7 +99, 292. 49E.9 102.57 8 99,189.52 496.4 $103.09 9 99,085.92 495.95 103.60 10 98,981.79 435.43 104.12 11 $98,877.15 494.91 $104.64 12 98,771. 99 494 . 39 106. 16 13 98, 666 . 30 493. 86 106. 69 14 98,560.08 493.33 106.22 15 $98,453.33 492.80 $106.75 IE 98, 346.04 $492.27 $107.28 17 98,238.22 491.73 107.82 18 $98, 129.8 491.19 $108.36 1 $99,900.45 500.00 100000 Loan Amount Tem in Years (1 to 50) APR percent Monthly Payment Total Money Paid $599.55 S215,838.19 Clear Amortize 900000000000000000 050694949 93838271 099999999999999999 + co co co co coStep by Step Solution
There are 3 Steps involved in it
Step: 1
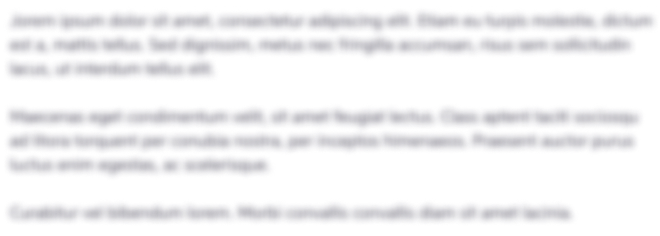
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started