Question
Wont compile please help #include #include #include #include #include Movie.h #include Text.h using namespace std; Movie *Movie::createMovie(Text *title, int length) { //dynamically allocate a new
Wont compile please help
#include
Movie *Movie::createMovie(Text *title, int length) { //dynamically allocate a new Movie Movie *myMovie = new Movie; //assign parameter data to structure members myMovie->movieTitle = title; myMovie->movieLength = length; return myMovie; } Movie *Movie::createMovie(Text *title, int length, int year, Text *genre, Text *rating, int nom, float stars) //all info is know { //dynamically allocate a new Movie Movie *myMovie = new Movie; //assign parameter data to structure members myMovie->movieTitle = title; myMovie->movieLength = length; myMovie->movieYear = year; myMovie->movieGenre = genre; myMovie->movieRating = rating; myMovie->movieOscars = nom; myMovie->movieNumStars = stars; return myMovie; } void Movie::destroyMovie() { destroyText(movieTitle); destroyText(movieGenre); destroyText(movieRating); delete myMovie; } void Movie::printMovieDetails() { cout << endl; cout << right << setw(30) << "Movie Title: " << left; displayText(movieTitle); cout << endl; cout << right << setw(30) << "Length (minutes): " << left << movieLength << endl; cout << right << setw(30) << "Year Released: " << left << movieYear << endl; cout << right << setw(30) << "Genre: " << left; displayText(movieGenre); cout << endl; cout << right << setw(30) << "Rating: " << left; displayText(movieRating); cout << endl; cout << right << setw(30) << "Number of Oscars Won: " << left << movieOscars << endl; cout << right << setw(30) << "Number of Stars: " << left << movieNumStars << endl << endl; } void Movie::printMovieDetailsToFile(ofstream &outFile) { char temp[1000]; strncpy(temp, getText(movieTitle), 1000); outFile << temp << endl; outFile << movieLength << endl; outFile << movieYear << endl; strncpy(temp, getText(movieGenre), 1000); outFile << temp << endl; strncpy(temp, getText(movieRating), 1000); outFile << temp << endl; outFile << movieOscars << endl; outFile << movieNumStars << endl; } void Movie::editMovie() { int choice; Text *tempText; char temp[100]; do { cout << " Which detail do you wish to edit? "; cout << "1. Title "; cout << "2. Length "; cout << "3. Year "; cout << "4. Genre "; cout << "5. Rating "; cout << "6. Number of Oscars Won "; cout << "7. Number of Stars "; cout << "8. DONE EDITING "; cout << "CHOOSE 1-8: "; cin >> choice; while (choice < 1 || choice > 8) { cout << " OOPS! Enter choice 1 through 8: "; cin >> choice; } cin.ignore(); switch (choice) { case 1: cout << " Current Title: "; displayText(movieTitle); destroyText(movieTitle); cout << " NEW TITLE: "; cin.getline(temp, 100); tempText = createText(temp); movieTitle = tempText; break; case 2: cout << " Current Length: " << movieLength; cout << " NEW LENGTH: "; cin >> movieLength; break; case 3: cout << " Current Year: " << movieYear; cout << " NEW LENGTH: "; cin >> movieYear; break; case 4: cout << " Current Genre: "; displayText(movieGenre); destroyText(movieGenre); cout << " NEW GENRE: "; cin.getline(temp, 100); tempText = createText(temp); movieGenre = tempText; break; case 5: cout << " Current Rating: "; displayText(movieRating); destroyText(movieRating); cout << " NEW GENRE: "; cin.getline(temp, 100); tempText = createText(temp); movieRating = tempText; break; case 6: cout << " Current Number of Oscars Won: " << movieOscars; cout << " NEW NUMBER OF OSCARS: "; cin >> movieOscars; break; case 7: cout << " Current Star Rating from IMDB: " << movieNumStars; cout << " NEW STAR RATING: "; cin >> movieNumStars; break; } } while (choice != 8); } Text *Movie::getMovieTitle() { return movieTitle; } int Movie::getMovieLength() { return movieLength; } int Movie::getMovieYear() { return movieYear; } Text *Movie::getMovieGenre() { return movieGenre; } Text *Movie::getMovieRating() { return movieRating; } int Movie::getMovieOscars() { return movieOscars; } float Movie::getMovieNumStars() { return movieNumStars; } void Movie::setMovieTitle(Text *title) { movieTitle = title; } void Movie::setMovieLength(int length) { movieLength = length; } void Movie::setMovieYear(int year) { movieYear = year; } void Movie::setMovieGenre(Text *genre) { movieGenre = genre; } void Movie::setMovieRating(Text *rating) { movieRating = rating; } void Movie::setMovieOscars(int oscars) { movieOscars = oscars; } void Movie::setMovieNumStars(float stars) { movieNumStars = stars; }
Movie.h file
#ifndef MOVIE_H #define MOVIE_H #include "Text.h" #include
Text.h
#ifndef TEXT_H #define TEXT_H
#include
struct Text { const char* textArray; int textLength; };
/* Function Name: createText() Parameters: Send a pointer to a constant character array or a string literal to this function Returns: A pointer to a new Text variable, which contains the c-string & the length of the string Purpose: To create a new Text variable */ Text* createText(const char*);
/* Function Name: destroyText() Parameters: Send a pointer to a Text variable, which contains a c-string & length of the string Returns: nothing (void) Purpose: release dynamically allocated memory that the pointer is pointing to. */ void destroyText(Text*);
/* Function Name: displayText() Parameters: Send a pointer to a Text variable, which contains a c-string & length of the string Returns: nothing (void) Purpose: prints out the string (character array) */ void displayText(Text*);
/* Function Name: getText() Parameters: Send a pointer to a Text variable, which contains a c-string & length of the string Returns: pointer to a constant character array */ const char* getText(Text*);
/* Function Name: getLength() Parameters: Send a pointer to a Text variable, which contains a c-string & length of the string Returns: the length of the string */ int getLength(Text*);
#endif
Movie.h
Step by Step Solution
There are 3 Steps involved in it
Step: 1
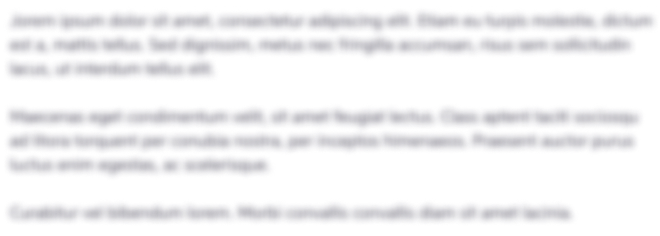
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started