Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Working with the ArrayList Class, study the Programming Activity Guidance document provided below. Make sure you compare your output to the Example Programming Activity 9-2
Working with the ArrayList Class, study the Programming Activity Guidance document provided below.
Make sure you compare your output to the Example Programming Activity 9-2 Output document (provided below the guidance)
Programming Activity 9-2 Guidance
=================================
9-2 is 1-dimensional not 2-dimensional
--------------------------------------
There are 2 topics in chapter 9: 2D arrays and the 1D ArrayList.
Activity 9-1 (the topic of Discussion Forum 1) was about 2D arrays.
This Activity 9-2 does not have any multi-dimensional arrays.
Instead, it has an ArrayList called "carList", which is a single dimensional entity.
The simplest way to loop through an ArrayList is to use an "enhanced for loop".
9-2 enhanced for loop
---------------------
For 9-2, you have to write the code for parts 2 through 5. The framework
already provides the code for part 1.
Each part that you write (2 through 5) should use an "enhanced for loop"
to loop through the ArrayList called carList, which is an ArrayList of
Auto objects.
You can use the exact same "enhanced for loop" for each part, with the
differences being the code that comes before the loop (if any), the code
that makes up the body of the loop, and the code that comes after the
loop (if any).
For each part, don't forget to properly call the framework's animate
method as the last statement in the body of the "enhanced for loop".
Knowing how to code an enhanced "for" loop is a key part of 9-2.
See section "9.7.3 Looping Through an ArrayList Using an Enhanced for Loop".
Enhanced "for" loop example
---------------------------
Here is an example of an enhanced for loop that loops through an
ArrayList of Book classes:
for (Book book : bookList)
{
// Do something with the current book for this iteration
// of the loop. Let's suppose it has a function called
// getTitle() that returns its title as a String:
String title = book.getTitle();
System.out.println("Title: " + title);
}
Don't get hung up on what's inside the loop.
What I gave here inside the loop is just an example.
You can do whatever you want inside the loop.
For this example, "book" is always a reference to the current book in the list.
The loop goes through the entire list one book at a time.
For each book, you do what needs to be done.
It's a simple and very useful looping mechanism.
Your enhanced for loop for 9-2 will follow this pattern, but
its ArrayList is called carList and contains Auto objects.
Enhanced "for" loop vs. regular for loop
----------------------------------------
Let's compare the "enhanced for loop" to the "regular for loop" for
looping through an ArrayList.
On page 616 of your textbook, it provides the following "regular for
loop" example for an ArrayList:
Auto currentAuto;
for (int i = 0; i
{
currentAuto = listOfAutos.get(i);
// Do something with currentAuto
}
Now let's look at an equivalent "enhanced for loop" for this:
(note that I used "auto" instead of "currentAuto")
for (Auto auto : listOfAutos)
{
// Do something with auto
}
Both of these do the same thing. However, clearly the second
loop is simpler and more efficient to write than the first loop.
9-2 part 2
----------
In order to print the list of cars, you must loop through the list in order to include
each car.
An "enhanced for loop" allows you to access each car in the list.
For each car, you use its toString() function, which gives you its elements, each separated
by a space. Look in the Auto.java file to see the toString() function. You will use toString()
in conjunction with System.out.println() in order to show each car's elements on a separate line
on the system console.
9-2 part 3
----------
For 9-2 part 3, you are adding code inside the setModelValues(String model) function.
You should add your code between the comments there that tell you where your code
should start and end.
A model is passed into the function via the String parameter "model".
You are supposed to iterate through all the cars and set the model of each car
to be this passed in model. Look in the Auto class to see its setter function
that you will use.
Do not concern yourself with all the framework code. It's OK to look at that code,
but you are not expected to understand all of it. Your task for part 3 is just to
code the setModelValues function as instructed.
Do not forget the call to animate as instructed in the framework comments. It should
be inside your enhanced "for" loop right after you set the model.
9-2 part 3 setter function
--------------------------
A "setter" function is another name for what the book calls a "mutator" function.
If you look in the Auto.java file, you will see the following function:
// Mutator method:
// Allows client to set model
public void setModel( String newModel )
{
model = newModel;
}
If you have an Auto object, say named "car", you could set its model
by using this function, of course passing in the model as a String.
If "model" is a String that holds the model, then you would simply write:
car.setModel(model);
9-2 part 4
----------
To find a maximum, the standard pattern is to initialize your maximum
value variaable to the miles driven of the first car in the list.
However, for this program, since the miles driven are never negative,
it is simpler to initialize the maximum value to 0.
Here is freebie starter code that does everything except one small
part that you must figure out (prior to the call to animate):
int maximum = 0;
for (Auto car : carList)
{
// You figure out what code goes here:
animate(car, maximum);
}
return maximum;
9-2 part 5
----------
Each car in the list has a model, which is a String that identifies the
model of that car. The part 5 function receives a model value as a parameter.
It wants you to look through the list and count how many of the cars in
that list have a model name that matches the passed in parameter.
For each car, use its getModel() function to get its model as a String.
Then use that String object's equals method to compare the car's model to
the model value that was passed into the function.
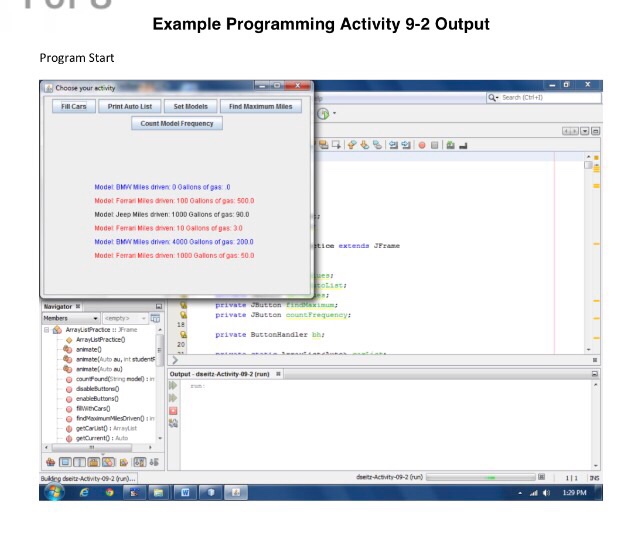
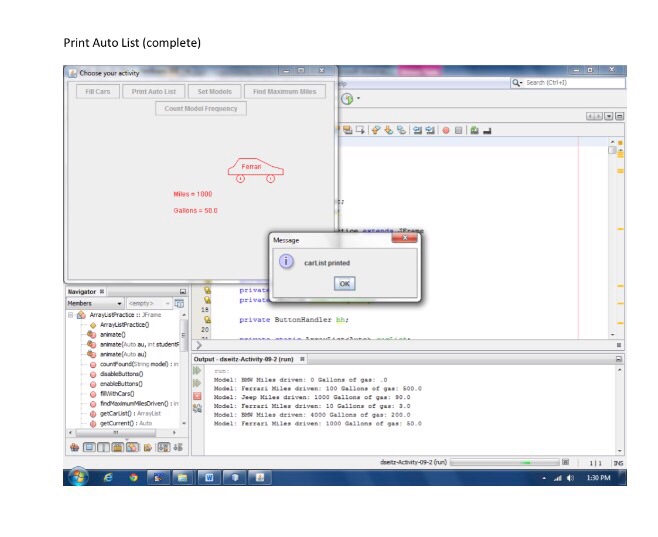
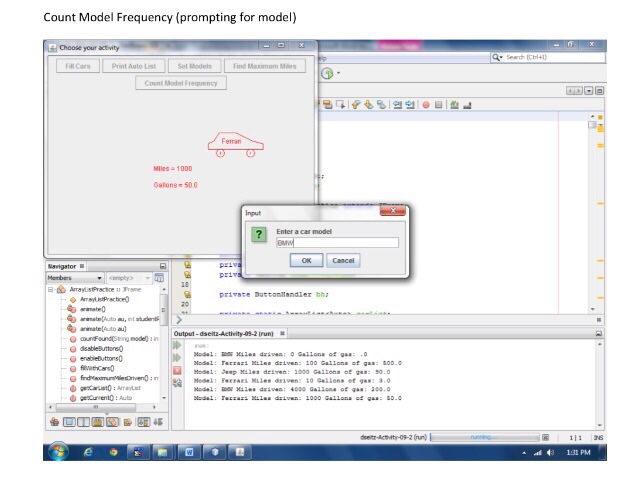
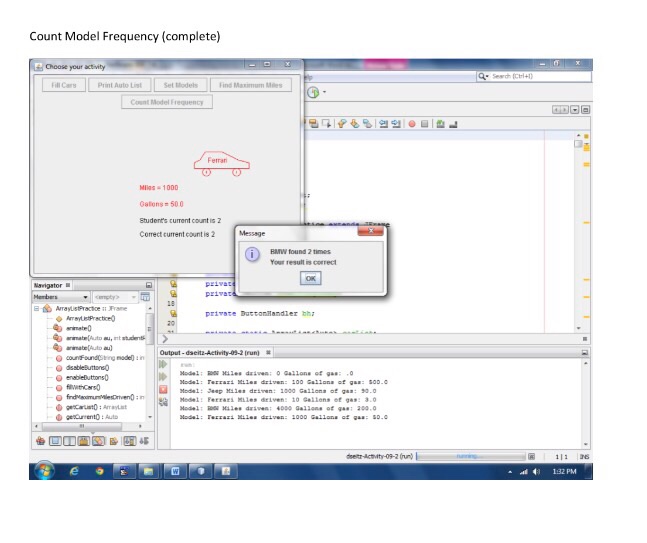
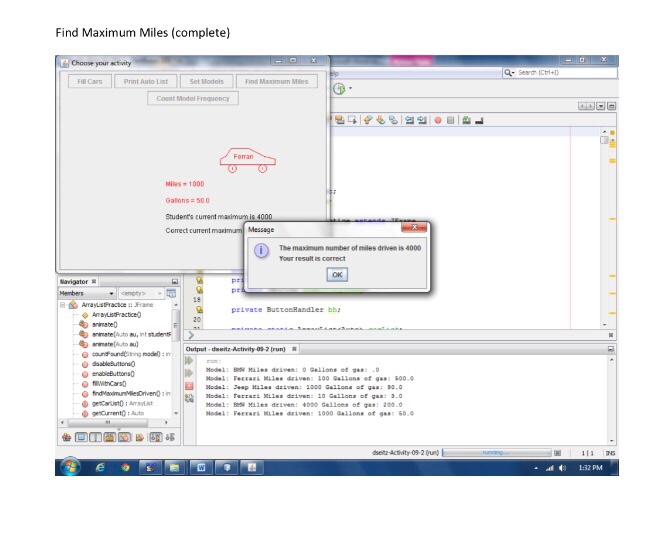
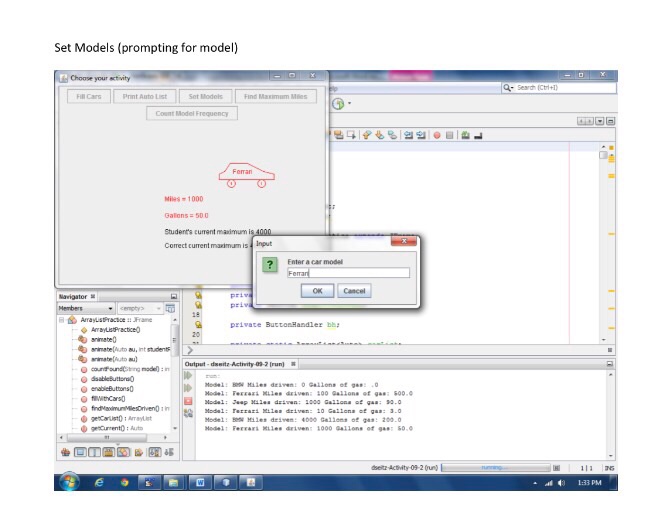
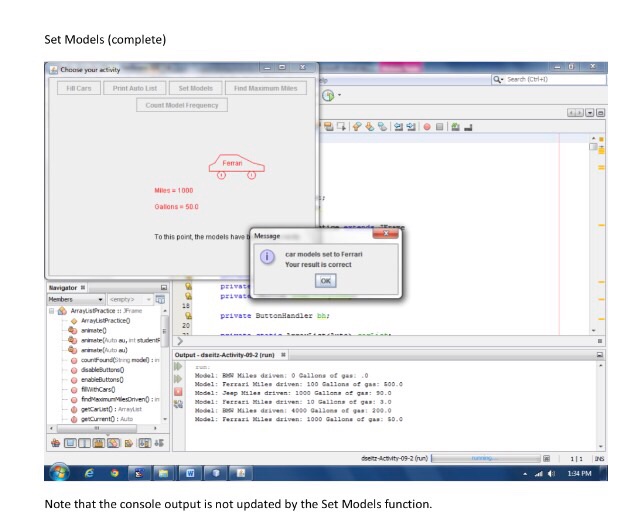
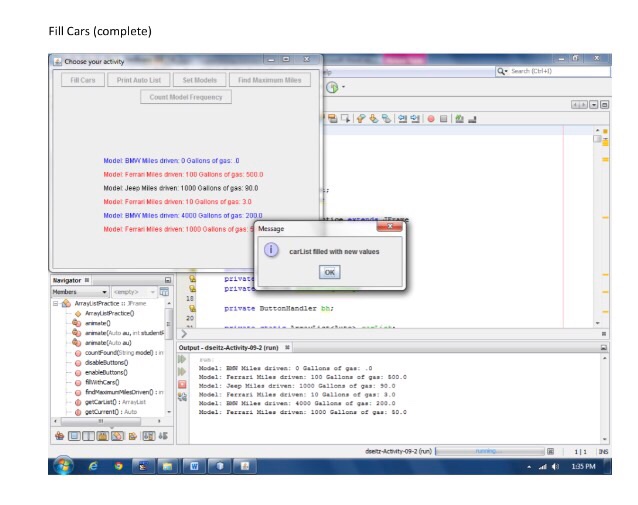
Step by Step Solution
There are 3 Steps involved in it
Step: 1
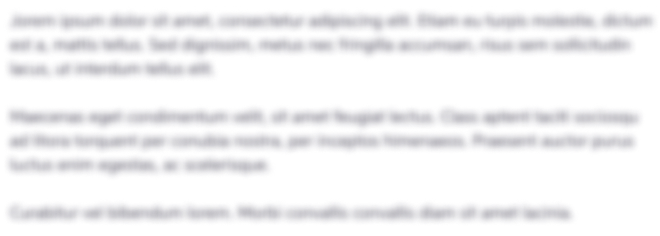
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started