Question
Workshop #5: Member and Helper operators V 0.9 (due date moved one day back to give students a chance to celebrate thanksgiving day ) v
Workshop #5: Member and Helper operators
V 0.9 (due date moved one day back to give students a chance to celebrate thanksgiving day ) v 1.0 (DIY: corrected typeconversion test bug in tester program)
Learning Outcomes
Upon successful completion of this workshop, you will have demonstrated the abilities to:
- overload binary member operators
- create and define type conversion operators
- create and define helper operators (without using the friendship of a C++ class)
- reuse member operators
- describe what you have learned in completing this workshop.
Submission Policy
This workshop is divided into two coding parts and one non-coding part:
- Part 1: A step-by-step guided workshop, worth 50% of the workshop's total mark that is due onThursdayFriday at 23:59:59of the week of your scheduled lab.
Please note that the part 1 section isnot to be started in your first session of the week. You should start it on your own before the day of your OOP244 class and join the first session of the week to ask for help and correct your mistakes (if there are any).
- Part 2 (DIY): A Do It Yourself type of workshop that is much more open-ended and is worth 50% of the workshop's total mark. This part is due onSundayMonday at 23:59:59of the week of your scheduled lab.
- reflection: non-coding part, to be submitted together withDIYpart. The reflection doesn't have marks associated with it but can incur apenalty of max 40% of the whole workshop's markif your professor deems it insufficient (you make your marks from the code, but you can lose some on the reflection).
- Submissions of part 2 that do not contain thereflection(that is thenon-coding part) are not considered valid submissions and are ignored.
If at the deadline the workshop is not complete, there is an extension ofone daywhen you can submit the missing parts.The code parts that are submitted late receive 0%.After this extra day, the submission closes; if the workshop is incomplete when the submission closes (missing at least one of the coding or non-coding parts),the mark for the entire workshop is 0%.
Every file that you submit must contain (as a comment) at the top: your name,your Seneca email,Seneca Student IDand thedatewhen you completed the work.
If the file contains only your work or work provided to you by your professor, add the following message as a comment at the top of the file:
I have done all the coding by myself and only copied the code that my professor provided to complete my workshops and assignments.
If the file contains work that is not yours (you found it online or somebody provided it to you),write exactly which part of the assignment is given to you as help, who gave it to you, or which source you received it from.By doing this you will only lose the mark for the parts you got help for, and the person helping you will be clear of any wrongdoing.
Compiling and Testing Your Program
All your code should be compiled using this command onmatrix:
g++ -Wall -std=c++11 -g -o ws file1.cpp file2.cpp ...
- -Wall: the compiler will report all warnings
- -std=c++11: the code will be compiled using the C++11 standard
- -g: the executable file will contain debugging symbols, allowingvalgrindto create better reports
- -o ws: the compiled application will be namedws
After compiling and testing your code, run your program as following to check for possible memory leaks (assuming your executable name isws):
valgrind ws
To check the output, use a program that can compare text files. Search online for such a program for your platform, or usediffavailable onmatrix.
Note: All the code written in workshops and the project must be implemented in thesddsnamespace.
LAB (part 1) (50%)
Your task for part one of this workshop is to complete the code of a class that represents anEgg Carton.
Develop your Egg Carton class in the"EggCarton"module provided in the workshop.
TheEggCartonclass encapsulates the size of the carton (i.e. the number of eggs it can hold), the number of eggs in the carton and whether the eggs arejumbosize orregular.
constant values
The followingconstantvalues are defined in the header file.
const int RegularEggWieght = 50;
const int JumboEggWieght = 75;
These wieghts are in Grams. (1000 Grams is one Kilo)
the constructor
EggCarton(int size=6, int noOfEggs=0, bool jumboSize=false)
The Egg Carton can be instantiated using itssize,number of eggsandif the eggs are jumbo size.
By default, the size of the carton is6, the number of eggs is0, and the eggs arenot jumbo size.
When created the following validation is done on the arguments of the constructor:
- sizemust be a coefficient of 6
- sizemust be between 6 and 36 (inclusively)
- the number of eggsshould be between 0 and thesizeof the Carton (inclusively)
If the values do not match the above criteria, the Carton is considered broken and unusable. This is done by calling the"setBroken()"privte method that sets thesizeand number of eggs in the carton to-1.
ostream& display(std::ostream& ostr) const
displays the Carton on the screen using theostrargument and the following code snippet:
// displays an Egg Carton
int cartonWidth = m_size == 6 ? 3 : 6;
for (int i = 0; i < m_size; i++) {
cout << ((i < m_noOfEggs) ? (m_jumbo ? 'O' : 'o') : '~');
if ((i + 1) % cartonWidth == 0) cout << endl;
}
The Carton is displayed only if it is not broken (seeoperator bool()overload), otherwise the message:"Broken Egg Carton!"< In the end, theostrreference is returned. istream& read(std::istream& istr) Reads comma-separated values fromistrto set theattributesofthe Egg Cartonand then returns theistrreference as follows: Type Conversion Operator overloads Boolean conversion. operator bool() const; Thisconstant conversionreturnstrueif thesizeof the Carton isgreater than zero(i.e. Returns true if the Carton is not broken) otherwise, it will returnfalse. Integer conversion operator int() const; Thisconstant conversionreturns thenumber of eggsif the Carton is not broken (use boolean conversion) otherwise, it will return-1 Double Conversion operator double() const; Thisconstant conversionreturns thetotal weightof the eggs using theconstant weight valuesdefined in the header file inKilos. (noOfEggs x WeightOfOnEgg)/1000.0 If the Carton is broken this conversion returns-1.0. Unary Operator overloads: prefixoperator-- If the Carton isnot brokenand thenumber of eggsis more thanzero, it will reduce it by one. In the end it returns the reference of thecurrent EggCarton object(*this). prefixoperator++ If the Carton isnot brokenit will add one thenumber of eggs. If thenumber of eggsexceeds thesizeof the carton, the Carton will be consideredbroken and unusable. In the end, return the reference of thecurrent EggCarton object(*this). postfixoperator-- Creates a local copyof the current EggCarton object (*this), calls the prefixoperator--and returns the local copyby value. postfixoperator++ Creates a local copyof the current EggCarton object (*this), calls the prefixoperator++and returns the local copyby value. Binary Member Operators EggCarton& operator=(int value) Sets the number of eggs tothe integer valueand If thenumber of eggsexceeds thesize, it will set the Carton tobroken. Returns a reference to thecurrent objectat the end. EggCarton& operator+=(int value) Addthe valueto thenumber of eggsif the Carton is not broken. If thenumber of eggsexceeds thesize, it will set the Carton to broken. Returns a reference to the current object at the end. EggCarton& operator+=(EggCarton& right) If the Carton is not broken it willmovethe eggs from therightCarton to the empty spots of thecurrent Cartonas much as it can. If there is not enough space in the current Carton, the rest of the eggs are left inthe right one. For example, if the current Carton has 3 empty spots and there are 7 eggs in the right Carton after this operator runs, the current Carton will be full and the right Carton will have 4 eggs in it. In the end, return a reference to the current object. bool operator==(const EggCarton& right) const Returntrueif thedifferencebetween theweight of the currentCarton and theweight of the rightCarton is between -0.001 and 0.001 kilos otherwise, returnfalse. Helper Binary Operator Overload int operator+(int left, const EggCarton& right) If the right operand is not broken it will return the sum of"left"and number of eggs in the"right". Otherwise, it will return the value of theleftonly. ostream& operator<<(ostream& ostr, const EggCarton& right) displays the EggCarton and returnsostr; istream& operator>>(istream& istr, EggCarton& right) Reads the specs from the console into therightCarton and returnsistr. Tester program: // Workshop #5: // Version: 0.9 // Date: 2021/10/06 // Author: Fardad Soleimanloo // Description: // This file tests the lab section of your workshop /////////////////////////////////////////////////// #include #include "EggCarton.h" using namespace std; using namespace sdds; void IOTest(); void typeConversionTest(); void unaryOperatorTest(); void BinaryOperators(); int main() { IOTest(); typeConversionTest(); unaryOperatorTest(); BinaryOperators(); return 0; } void BinaryOperators() { EggCarton e1, e2(6, 4), e3(6, 5), bad(40); cout << endl << "Binary Member operator tests" << endl; cout << "e1: " << int(e1) << ", e2: " << int(e2) << ", e3: " << int(e3) << endl; e1 = e2 += e3; cout << "e1 = e2 += e3;" << endl; cout << "e1: " << int(e1) << ", e2: " << int(e2) << ", e3: " << int(e3) << endl; bad += e3; cout << "bad += e3;" << endl; cout << "bad: " << int(bad) << ", e3: " << int(e3) << endl; bad += e3; cout << "---------------------------------------------" << endl; e1 = 20; e2 = 2; cout << "e1 = 20; e2 = 2;" << endl; cout << "e1: " << int(e1) << ", e2: " << int(e2) << endl; e1 = 2; cout << "e1 = 2;" << endl; cout << "e1: " << int(e1) << endl; cout << "---------------------------------------------" << endl; e1 += 2; e2 += 1; e3 += 4; cout << "e1 += 1; e2 += 1; e3 += 4;" << endl; cout << "e1: " << int(e1) << ", e2: " << int(e2) << ", e3: " << int(e3) << endl; cout << "---------------------------------------------" << endl; e1 = EggCarton(6, 2, true); e2 = EggCarton(6, 3, false); e3 = EggCarton(18, 18, true); cout << "e1: " << int(e1) << ", e2: " << int(e2) << ", e3: " << int(e3) << endl; cout << "e1:" << endl << e1 << "e2:" << endl << e2 << "e3:" << endl < cout << "e1 is " << double(e1) << " kgs and e2 is " << double(e2) << " kgs therefore thier weights are " << (e1 == e2 ? "equal" : "different") << endl; cout << "e1 is " << double(e1) << " kgs and e3 is " << double(e3) << " kgs therefore thier weights are " << (e1 == e3 ? "equal" : "different") << endl; cout << "---------------------------------------------" << endl; cout << endl << "Binary non-member operator test" << endl; cout << "I have 5 eggs and there are " << int(e3) << " eggs in the Carton." << endl << "In total I have " << 5 + e3 << " eggs!" << endl; } void unaryOperatorTest() { EggCarton e1(6, 4), e2; cout << endl << "Unary operator tests" << endl; cout << "e1: " << int(e1) << endl; cout << "e2: " << int(e2) << endl; e2 = --e1; cout << "e2 = --e1;" << endl; cout << "e1: " << int(e1) << endl; cout << "e2: " << int(e2) << endl; cout << "--------------------------------" << endl; e2 = ++e1; cout << "e2 = ++e1;" << endl; cout << "e1: " << int(e1) << endl; cout << "e2: " << int(e2) << endl; cout << "--------------------------------" << endl; e2 = e1--; cout << "e2 = e1--;" << endl; cout << "e1: " << int(e1) << endl; cout << "e2: " << int(e2) << endl; cout << "--------------------------------" << endl; e2 = e1++; cout << "e2 = e1++;" << endl; cout << "e1: " << int(e1) << endl; cout << "e2: " << int(e2) << endl; cout << "End Unary operator tests" << endl; } void typeConversionTest() { EggCarton eggs[] = { {}, {36,20,true}, {36,20,false}, {42} }; cout << endl << "Type Conversion operator tests" << endl; for (int i = 0; i < 4; i++) { cout.setf(ios::fixed); cout.precision(2); if (eggs[i]) { cout << int(eggs[i]) << " Eggs:" << endl; cout << double(eggs[i]) << " kgs" << endl << "---------------" << endl; } else { cout << "Bad or Broken Carton" << endl; } } cout << "END Type Conversion operator tests" << endl; } void IOTest() { cout << endl << "Operator <<, Operator >>, display and read test" << endl; EggCarton eggs[] = { {}, {10}, {10,5}, {6,-1}, {42,10}, {36,37}, {6,4,true}, {6,4,false}, {18,10,true}, {18,10,false} }; for (int i = 0; i < 10; i++) { cout << eggs[i] << "----------" << endl; } cout << "Enter the following valid values:" << endl << " j,24,20" << endl << ">"; cin >> eggs[0]; cout << eggs[0] << "----------" << endl; cout << "Enter the following valid values:" << endl << " r,24,20" << endl << ">"; cin >> eggs[0]; cout << eggs[0] << "----------" << endl; cout << "Enter the following invalid values:" << endl << " j,44,20" << endl << ">"; cin >> eggs[0]; cout << eggs[0] << "----------" << endl; cout << "Enter the following invalid values:" << endl << " j,24,40" << endl << ">"; cin >> eggs[0]; cout << eggs[0] << "----------" << endl; cout << "END Operator <<, Operator >>, display and read test" << endl; } Execution Sample Operator <<, Operator >>, display and read test ~~~ ~~~ ---------- Broken Egg Carton! ---------- Broken Egg Carton! ---------- Broken Egg Carton! ---------- Broken Egg Carton! ---------- Broken Egg Carton! ---------- OOO O~~ ---------- ooo o~~ ---------- OOOOOO OOOO~~ ~~~~~~ ---------- oooooo oooo~~ ~~~~~~ ---------- Enter the following valid values: j,24,20 >j,24,20 OOOOOO OOOOOO OOOOOO OO~~~~ ---------- Enter the following valid values: r,24,20 >r,24,20 oooooo oooooo oooooo oo~~~~ ---------- Enter the following invalid values: j,44,20 >j,44,20 Broken Egg Carton! ---------- Enter the following invalid values: j,24,40 >j,24,40 Broken Egg Carton! ---------- END Operator <<, Operator >>, display and read test Type Conversion operator tests 0 Eggs: 0.00 kgs --------------- 20 Eggs: 1.50 kgs --------------- 20 Eggs: 1.00 kgs --------------- Bad or Broken Carton END Type Conversion operator tests Unary operator tests e1: 4 e2: 0 e2 = --e1; e1: 3 e2: 3 -------------------------------- e2 = ++e1; e1: 4 e2: 4 -------------------------------- e2 = e1--; e1: 3 e2: 4 -------------------------------- e2 = e1++; e1: 4 e2: 3 End Unary operator tests Binary Member operator tests e1: 0, e2: 4, e3: 5 e1 = e2 += e3; e1: 6, e2: 6, e3: 3 bad += e3; bad: -1, e3: 3 --------------------------------------------- e1 = 20; e2 = 2; e1: -1, e2: 2 e1 = 2; e1: -1 --------------------------------------------- e1 += 1; e2 += 1; e3 += 4; e1: -1, e2: 3, e3: -1 --------------------------------------------- e1: 2, e2: 3, e3: 18 e1: OO~ ~~~ e2: ooo ~~~ e3: OOOOOO OOOOOO OOOOOO e1 is 0.15 kgs and e2 is 0.15 kgs therefore thier weights are equal e1 is 0.15 kgs and e3 is 1.35 kgs therefore thier weights are different --------------------------------------------- Binary non-member operator test I have 5 eggs and there are 18 eggs in the Carton. In total I have 23 eggs!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
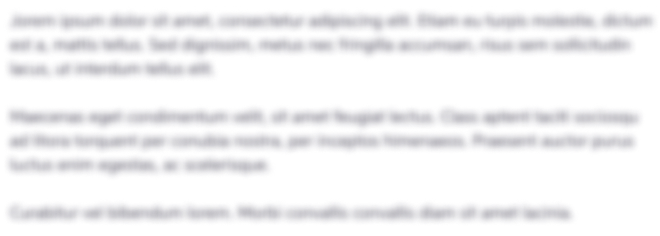
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started