Question
Would really appreciate a small explanation or how you got to the solution for some of these. Do not know how to think about these
Would really appreciate a small explanation or how you got to the solution for some of these. Do not know how to think about these solutions on my own. Do I use truth tables? How can I figure these out?
* bitOr - x|y using only ~ and &
* Example: bitOr(6, 5) = 7
* Legal ops: ~ &
* Max ops: 8
* Rating: 1
*/
int bitOr(int x, int y) {
return 0;
}
int bitXor(int x, int y) {
//& is always the difference between Xor (by definition)
return 0;
}
* setFirst - returns value with n upper bits set to 1
* and 32-n lower bits set to 0
* You may assume 0 <= n <= 32
* Example: setFirst(4) = 0xF0000000
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 10
* Rating: 2
*/
int setFirst(int n) {
return 2;
}
* fourthBits - return word with every fourth bit (starting from the LSB) set to 1
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 8
* Rating: 1
*/
int fourthBits(void) {
return 2;
}
* rotate4 - Rotate x to the left by 4
* Examples: rotate4(0x87654321) = 0x76543218
* Legal ops: ~ & ^ | + << >> !
* Max ops: 10
* Rating: 2
*/
int rotate4(int x) {
return 2;
}
/*
* logicalShift - shift x to the right by n, using a logical shift
* Can assume that 0 <= n <= 31
* Examples: logicalShift(0x87654321,4) = 0x08765432
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 20
* Rating: 3
*/
int logicalShift(int x, int n) {
return 2;
}
* bitParity - returns 1 if x contains an odd number of 0's
* Examples: bitParity(5) = 0, bitParity(7) = 1
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 20
* Rating: 4
*/
int bitParity(int x) {
return 2;
}
* tmin2 - return second smallest two's complement integer
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 6
* Rating: 2
*/
int tmin2(void) {
return 2;
}
/*
* isZero - returns 1 if x == 0, and 0 otherwise
* Examples: isZero(5) = 0, isZero(0) = 1
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 2
* Rating: 1
*/
int isZero(int x) {
return 2;
}
/*
* is0orMore - return 1 if x >= 0, return 0 otherwise
* Example: is0orMore(-1) = 0. is0orMore(0) = 1.
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 6
* Rating: 3
*/
int is0orMore(int x) {
return 2;
}
/*
* isNotEqual - return 0 if x == y, and 1 otherwise
* Examples: isNotEqual(5,5) = 0, isNotEqual(4,5) = 1
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 6
* Rating: 2
*/
int isNotEqual(int x, int y) {
return 2;
}
/*
* conditional - same as x ? y : z
* Example: conditional(2,4,5) = 4
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 16
* Rating: 3
*/
int conditional(int x, int y, int z) {
return 2;
}
/*
* isSmaller - if x < y then return 1, else return 0
* Example: isSmaller(4,5) = 1.
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 24
* Rating: 3
*/
int isSmaller(int x, int y) {
return 2;
}
/*
* satMul4 - multiplies by 4 but but when positive overflow occurs, returns
* maximum possible value (TMax), and when negative overflow occurs,
* it returns minimum positive value (TMin).
* Examples: satMul4(0x10000000) = 0x40000000
* satMul4(0x20000000) = 0x7FFFFFFF (saturate to TMax)
* satMul4(0x80000000) = 0x80000000 (saturate to TMin)
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 20
* Rating: 3
*/
int satMul4(int x) {
return 2;
}
/*
* subOK - Determine if can compute x-y without overflow
* Example: subOK(0x80000000,0x80000000) = 1,
* subOK(0x80000000,0x70000000) = 0,
* Legal ops: ! ~ & ^ | + << >>
* Max ops: 20
* Rating: 3
*/
int subOK(int x, int y) {
return 2;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
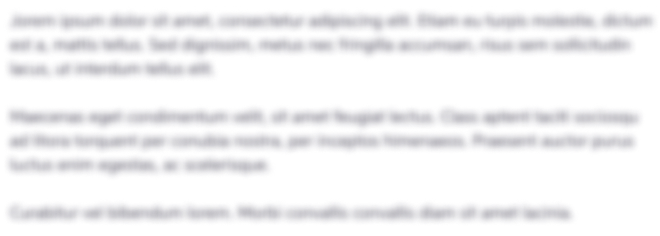
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started