Question
Write a birthday lookup program. Read a data set of 1000 names and birthdays from a file that contains this information. Your program will allow
Write a birthday lookup program. Read a data set of 1000 names and birthdays from a file that contains this information. Your program will allow the user to enter a date and print the names of every person whose birthday is that date.
Input file: contactdata.txt
MUST INCLUDE COMMENTS
The program has to do two things: read in the data and handle user queries.
- The data is one file. For each person, the name is listed on one line and the birthday is listed on the next line. The birthdays use month/day format, so, for example, December 1 is 12/1 and July 4 is 7/4.
- The program should prompt the user with this message: Please enter a date, or * to quit. The user enters a date in the month/day format, and the program prints the names of all people in the data set with the specified birthday. If a match cannot be found, the program should print No one has that birthday. Repeat this until the user enters *.
Hint: Create a Contact class to hold the name and the birthday of a single person.
Hint: Since you are given the number of contacts (1000), you can use an array of Contacts or an ArrayList of Contacts, your choice.
Hint: As you read in the data, for every two lines, create a new Contact object and add it to the array or ArrayList.
Hint: This problem has two main steps: read the data, and process user queries. Therefore, we can break this down into two methods, and call them both from the main method:
public static void main(String[] args) { // declare your array or ArrayList called contacts readData(contacts); processQueries(contacts); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
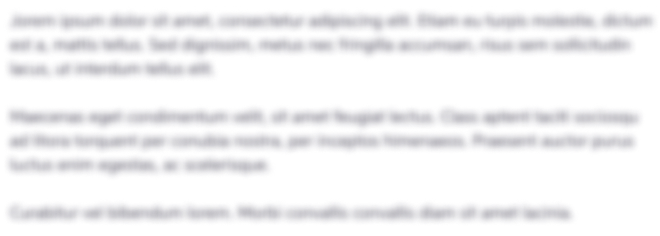
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started