Question
Write a C++ program: Create the Todo_item Class In a3.cpp, underneath the code you wrote for Date, write a class called Todo_item that inherits from
Write a C++ program:
Create the Todo_item Class
In a3.cpp, underneath the code you wrote for Date, write a class called Todo_item that inherits from the Todo_item_base class inTodo_item_base.h:
// a3.cpp // ... #include "Todo_item_base.h" // ... code for Date ... class Todo_item : public Todo_item_base { private: // ... public: // ... };
In the private part of To_do_item, add the variables for the item's due date (use the Date class you just wrote), a string description (such as "study for midterm"), and whether or not it has been completed (an item is either completed, or not completed).
In the public part of To_do_item, add a constructor that takes a string description and Date due date as input. Use those to initialize the variables in the private part using an initializer list. The item should always be initialized as not done.
Then add all the getters and setters, as listed in Todo_item_base.
Test what you've done by adding this function to a3.cpp:
void step_3_1_test() { Date xmas(25, 12, 2018); Todo_item buy_gifts("Buy gifts", xmas); assert(buy_gifts.get_description() == "Buy gifts"); assert(buy_gifts.get_due_date().get_day() == xmas.get_day()); assert(buy_gifts.get_due_date().get_month() == xmas.get_month()); assert(buy_gifts.get_due_date().get_year() == xmas.get_year()); assert(!buy_gifts.is_done()); buy_gifts.set_done(); assert(buy_gifts.is_done()); buy_gifts.set_not_done(); assert(!buy_gifts.is_done()); Date earlier(20, 12, 2018); buy_gifts.set_due_date(earlier); assert(buy_gifts.get_due_date().get_day() == earlier.get_day()); assert(buy_gifts.get_due_date().get_month() == earlier.get_month()); assert(buy_gifts.get_due_date().get_year() == earlier.get_year()); buy_gifts.set_description("Buy gifts for family"); assert(buy_gifts.get_description() == "Buy gifts for family"); cout << "All step_3_1 tests passed! "; }
Call it in main() to check the results. Make sure to also call all previous tests in main().
Step 3.2: Add to_string
Uncomment the to_string method in Todo_item_base.h. In it's body, add code to that creates and returns a string representation of the item. It should have this format: "dd/mm/yyyy? description". The first 10 characters are the due date (from Date::to_string). The ? is either the character @ (meaning the item is done), or the character ! (meaning the item is not done). Then there is one space, followed by a description that continues to the end of the line.
Test what you've done by adding this function to a3.cpp:
void step_3_2_test() { Date xmas(25, 12, 2018); Todo_item buy_gifts("Buy gifts", xmas); assert(buy_gifts.to_string() == "25/12/2018! Buy gifts"); buy_gifts.set_done(); assert(buy_gifts.to_string() == "25/12/2018@ Buy gifts"); cout << "All step_3_2 tests passed! "; }
Call it in main() to check the results. Make sure to also call all previous tests in main().
Step 3.3: Add to_html
Uncomment the to_html method in Todo_item_base.h. In its body, write code that creates and returns a string that can be used as an item in an HTML. The returned string has one of these formats:
In both cases, the string is wrapped by
Notice that there is no @ or ! character after the date in the output of to_html.
Test what you've done by adding this function to a3.cpp:
void step_3_3_test() { Date xmas(25, 12, 2018); Todo_item buy_gifts("Buy gifts", xmas); assert(buy_gifts.to_html_item() == "
Call it in main() to check the results. Make sure to also call all previous tests in main().
Step 3.4: Add operator<
Implement operator< for Todo_item. When comparing two items, only the due date is used in the comparison (the description and completed status are ignored). So if a and b are Todo_items, then a < b is true if the due date of a is before the due date of b, and false otherwise.
Test what you've done by adding this function to a3.cpp:
void step_3_4_test() { Date halloween(31, 10, 2018); Date xmas(25, 12, 2018); Date easter(21, 4, 2019); Todo_item carve_pumpkin("Carve pumpkin", halloween); Todo_item buy_gifts("Buy gifts", xmas); Todo_item weave_basket("Weave basket", easter); assert(carve_pumpkin < buy_gifts); assert(buy_gifts < weave_basket); assert(carve_pumpkin < weave_basket); assert(!(carve_pumpkin < carve_pumpkin)); assert(!(buy_gifts < carve_pumpkin)); assert(!(weave_basket < buy_gifts)); assert(!(weave_basket < carve_pumpkin)); cout << "All step_3_4 tests passed! "; }
Call it in main() to check the results. Make sure to also call all previous tests in main().
Step 3.5: Add a Constructor from a String
Add another constructor to Todo_item that takes a string as input. The string is formatted exactly the same as the output of Todo_item::to_string. For example, Todo_item("25/12/2018! Buy gifts") creates a Todo_item with the due date 25/12/2018, the description "Buy gifts", and a completion status of not done.
Test what you've done by adding this function to a3.cpp:
void step_3_5_test() { Todo_item a("01/01/0000@ buy a hamster"); assert(a.get_description() == "buy a hamster"); assert(a.get_due_date().get_day() == 1); assert(a.get_due_date().get_month() == 1); assert(a.get_due_date().get_year() == 0); assert(a.is_done()); Todo_item b("01/01/2018! sell hamster"); assert(b.get_description() == "sell hamster"); assert(b.get_due_date().get_day() == 1); assert(b.get_due_date().get_month() == 1); assert(b.get_due_date().get_year() == 2018); assert(!b.is_done()); assert(a < b); assert(!(b < a)); cout << "All step_3_5 tests passed! "; }
The todo_item_base.h has been given below:
// Todo_item_base.h
//////////////////////////////////////////////////////////////////////////// // // Todo_item_base class // ////////////////////////////////////////////////////////////////////////////
// tell the compiler that Todo_item, Date, and string are classes class Todo_item;
class Todo_item_base { public: // virtual destructor virtual ~Todo_item_base() {}
// getters virtual string get_description() const = 0; virtual bool is_done() const = 0; virtual Date get_due_date() const = 0;
// setters virtual void set_description(const string& new_description) = 0; virtual void set_done() = 0; virtual void set_not_done() = 0; virtual void set_due_date(const Date& new_due_date) = 0;
// printing // virtual string to_string() const = 0; // virtual string to_html_item() const = 0;
}; // class Todo_item_base
bool operator<(const Todo_item& t1, const Todo_item& t2);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
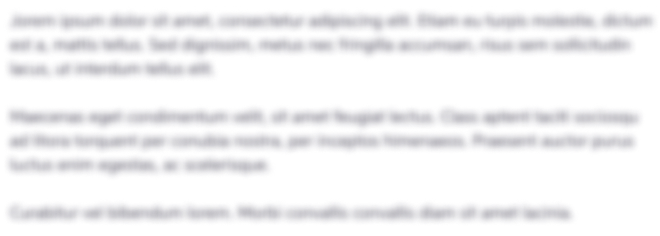
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started