Question
Write a C program for LNMIIT Library to maintain a link list of the books. The program should allow the librarian to add a book,
Write a C program for LNMIIT Library to maintain a link list of the books. The program should allow the librarian to add a book, issue a particular book to a student and display the number of issued and unissued books available in the library.
typedef struct{ char *studentName; int studentID; }Student; struct Book{ int ID; // Unique ID of the book char *title // Title of the book char *author; // Author(s) of the book int isIssued; // If the book is issued, it's value is 1, else 0 Student student; // Store details of the student to whom the book has been issued struct Book *nextBook; // Pointer to the next Book };
You may need to create a link list of Book to store information of various books in the library. Your program shall show a menu containing 4 options and shall ask user to enter one choice (a, b, c, d). Any other choice should prompt the user and allows them to re-enter their choice. You may write a function void Library_menu() to display the menu options.
The menu items and operations to be performed upon entering each of the choices are described below.
a. Add Book. Selecting this option will prompt the user to enter Book details (ID, title and author). This should add a record of the book in the link list. While inserting the details of a new book, make sure that the book with the same ID is not present in the link list. If the book with the same ID is present, prompt the user and allow them to enter the book details with a different ID. Once the insertion is done, print the message " is successfully added" (Example: How to program in C is successfully added). Again, the original menu containing the 4 options will be displayed.
b. Display. Selecting this option will provide the details of all the issued and unissued books available in the library. Again, the original menu containing the 4 options will be displayed.
c. Issue a book. Selecting this option will prompt the user to enter the book title that he/she wants to issue. Check if the book with that title is available for issue. If multiple books with the same title are avaiable for issue, issue any available book to the student and print a message that , with book ID has been issued to (ID: ). For example: How to program in C, with book ID 1123 has been issued to xyz(ID: 112). After that again display the menu containing 4 options.
d. Exit Menu. Selecting this option will terminate the program with a Good-Bye message
its solution is
// Name- Vaibhav silmana //roll no - 20mmt004
#include
struct Student{ char *studentname; int studentID; }; struct Book{ int ID; char *title; char *author; int issueID; struct Student student; struct Book *nextBook; }; struct Book *head; struct Book *save; struct Bok *p; struct Book *createnode(int n,char *a,char *b,int c,int d) { struct Book *ptr = (struct Book*)malloc(sizeof(struct Book)); ptr->ID=n; ptr->title=a; ptr->author=b; ptr->nextBook=NULL; ptr->issueID=0; return ptr; } void insert(struct Book *np) { if(head==NULL) { head=np; } else { save=head; head=np; np->nextBook=save; } } void display(struct Book *ptr) { while(ptr!=NULL) { printf("%d,%s,%s",ptr->ID,ptr->title,ptr->author); ptr=ptr->nextBook; } } void issue(char *name) { int c=0; if(head==NULL) { printf("no books available"); } else { while(head!=NULL) { if(head->title==name) { printf("book %s with book id: %d has been issued to ",head->title,head->ID); head->issueID=head->ID; } head=head->nextBook; c++; } } printf("total issued books are : %d",c); } int main() { head=NULL; char ch; struct Book *p=(struct Book*)malloc(sizeof(struct Book)); p=struct Book *createnode(int n,char *a,char *b,int c,int d); A: printf("enter a choice"); scanf("%c",&ch); switch(ch) { case 'a': insert(p); break; case 'b' : display(p); break; case 'c':; char *name; scanf("enter book title to be issued: %s",name); issue(name); break; case 'd': printf("good-bye"); exit(0); default: printf("enter valid choice!!"); break; } goto A; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
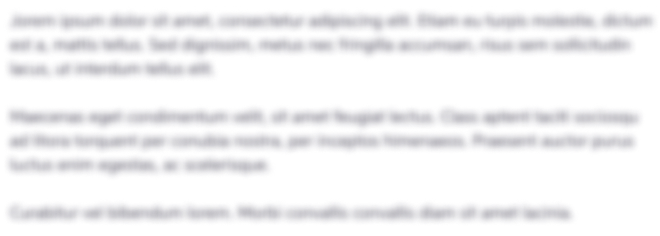
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started