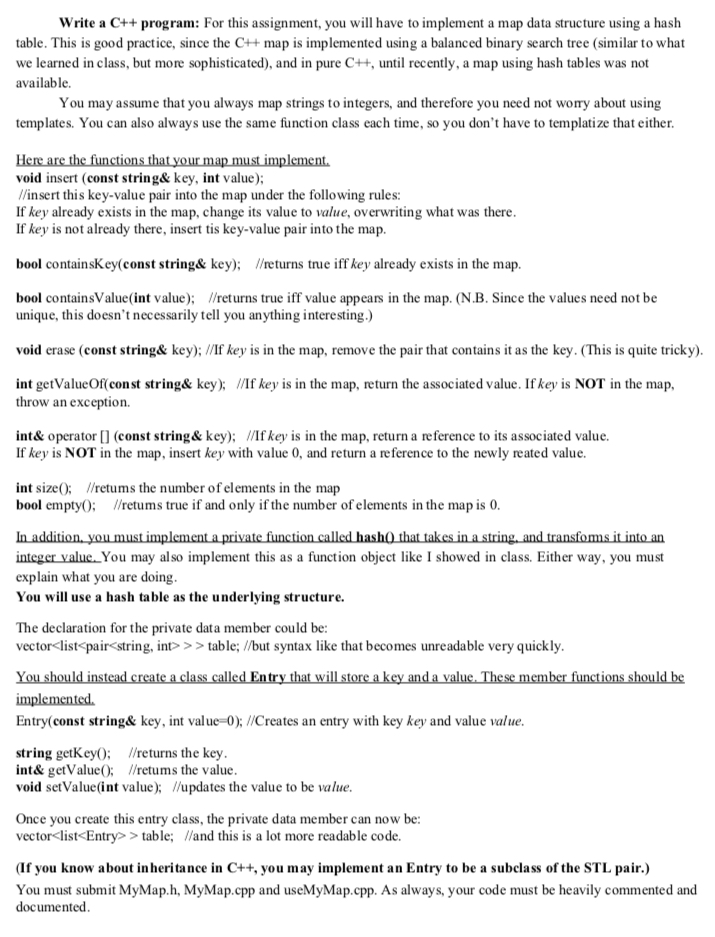
Write a C++ program: For this assignment, you will have to implement a map data structure using a hash table. This is good practice, since the C+map is implemented using a balanced binary search tree (similar to what we learned in class, but more sophisticated), and in pure C++, until recently, a map using hash tables was not available. You may assume that you always map strings to integers, and therefore you need not worry about using templates. You can also always use the same function class each time, so you don't have to templatize that either void insert (const string& key, int value); insert this key-value pair into the map under the following rules: If key already exists in the map, change its value to value, overwriting what was there If key is not already there, insert tis key-value pair into the map bool containsKey(const string& key); returns true iff key already exists in the map bool containsValue(int value /returns true iff value appears in the map. (N.B. Since the values need not be unique, this doesn't necessarily tell you anything interesting.) void erase (const string& key); /If key is in the map, remove the pair that contains it as the key. (This is quite tricky int getValueOf(con st string&key); /If key is in the map, return the associated value. If key is NOT in the map throw an exception. int& operator [] (const string& key); /If key is in the map, return a re ference to its associated value. If key is NOT in the map, insert key with value 0, and return a reference to the newly reated value. int size retums the number of elements in the map bool empty0: /retums true if and only if the number of elements in the map is 0 integer value. You may al so implement this as a function object like I showed in class. Either way, you must explain what you are doing You will use a hash table as the underlying structure. The declaration for the private data member could be: vector dist
> > table; but syntax like that becomes unreadable very quickly implemented Entry(const string& key, int value 0); //Creates an entry with key key and value value string getKey) /returns the key int& getValue0: /retums the value void setValue(int value) updates the value to be value Once you create this entry class, the private data member can now be: vector listEntrytable; /and this is a lot more readable code. (If you know about in heritance in C++,you may implement an Entry to be a subclass of the STL pair.) You must submit MyMap.h, MyMap.cpp and useMyMap.cpp. As always, your code must be heavily commented and documented Write a C++ program: For this assignment, you will have to implement a map data structure using a hash table. This is good practice, since the C+map is implemented using a balanced binary search tree (similar to what we learned in class, but more sophisticated), and in pure C++, until recently, a map using hash tables was not available. You may assume that you always map strings to integers, and therefore you need not worry about using templates. You can also always use the same function class each time, so you don't have to templatize that either void insert (const string& key, int value); insert this key-value pair into the map under the following rules: If key already exists in the map, change its value to value, overwriting what was there If key is not already there, insert tis key-value pair into the map bool containsKey(const string& key); returns true iff key already exists in the map bool containsValue(int value /returns true iff value appears in the map. (N.B. Since the values need not be unique, this doesn't necessarily tell you anything interesting.) void erase (const string& key); /If key is in the map, remove the pair that contains it as the key. (This is quite tricky int getValueOf(con st string&key); /If key is in the map, return the associated value. If key is NOT in the map throw an exception. int& operator [] (const string& key); /If key is in the map, return a re ference to its associated value. If key is NOT in the map, insert key with value 0, and return a reference to the newly reated value. int size retums the number of elements in the map bool empty0: /retums true if and only if the number of elements in the map is 0 integer value. You may al so implement this as a function object like I showed in class. Either way, you must explain what you are doing You will use a hash table as the underlying structure. The declaration for the private data member could be: vector dist > > table; but syntax like that becomes unreadable very quickly implemented Entry(const string& key, int value 0); //Creates an entry with key key and value value string getKey) /returns the key int& getValue0: /retums the value void setValue(int value) updates the value to be value Once you create this entry class, the private data member can now be: vector listEntrytable; /and this is a lot more readable code. (If you know about in heritance in C++,you may implement an Entry to be a subclass of the STL pair.) You must submit MyMap.h, MyMap.cpp and useMyMap.cpp. As always, your code must be heavily commented and documented