Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Project Description Write a program that performs various unit conversions between metric and imperial systems. The program asks the user if he or she
Project Description Write a program that performs various unit conversions between metric and imperial systems. The program asks the user if he or she wants to convert one of the options below (the exact menu format is shown in the sample run below): 1. From feet and inches to meters and centimeters 2. From meters and centimeters to feet and inches 3. From pounds and ounces to kilograms and grams 4. From kilograms and grams to pounds and ounces The program then performs the desired conversion. Have the user respond by typing the integer (1,2,3, or 4) for the desired type of conversion. The program reads the user's answer and then executes a selection statement. Each branch of this selection statement will be a function call. Thus, you will need in your program at least four (4) functions. Also, include a loop that lets the user repeat this computation for new input values until the user says he or she wants to end the program. If the user requests an invalid option, the program displays a message and generates the same menu again. The demo at the end will show you some sample runs, to give a better (visual) clarification. The four functions that you must include in your code are: void ImperialToMetricL(); // requests English length measure, converts to metric, outputs both void Metric TolmperialL(); // request metric length measure, converts to English, outputs both void ImperialToMetricW(); // requests English mass measure, converts to metric, outputs both void MetricTolmperialW(); // request metric mass measure, converts to English, outputs both As you can see from the description of the functions above, they (each) perform several tasks, namely request (read input), convert, and output. Feel free to implement these sub tasks in separate functions. Important Tips You must follow the tips below to get a full mark: 1. Use meaningful identifier names (don't use int x and double y) 2. Use comments throughout your code and explain the purpose of each function 3. Use functions and minimize the amount of coding in your main function. Create at least the four functions mentioned above Useful Hints The imperial system is also known as the English or British measurement system. There are 0.3048 meters in a foot, 100 centimeters in a meter, and 12 inches in a foot. Since the metric system can naturally handle meters and centimeters as a real number, you can define it as a double value. For example, 1.75 meters is equal to 1 meter and 75 centimeters. There are 0.4536 kilogram in a pound, 1000 grams in a kilogram, and 16 ounces in a pound. Since the metric system can naturally handle kilograms and grams as a real number, you can define it as a double value. For example, 1.75 kilograms is equal to 1 kilogram and 750 grams. To make the program easier, we will assume the user can insert ounces value that is higher than a pound. For example, 1 pound and 20 ounces is an acceptable input, even though there is only 16 ounces in a pound. The same is applicable to the feet and inches. When converting, you should be careful that 2 pounds and 5 ounces is not 2.5 pounds. Grade Distribution When you submit your program include the code, a short report that lists and explains what kind of variables and functions you used, and screen shots of your output. Don't forget, you have to submit both electronic and printed copy of your work in order to get a grade. The 10 points will be distributed as follows: Code: 7 Pts. o Comments, proper coding, variable naming and general layout (1 Pt.) o Properly displaying the menu and repeating it within a loop (2 Pts.) Project Description Write a program that performs various unit conversions between metric and imperial systems. The program asks the user if he or she wants to convert one of the options below (the exact menu format is shown in the sample run below): 1. From feet and inches to meters and centimeters 2. From meters and centimeters to feet and inches 3. From pounds and ounces to kilograms and grams 4. From kilograms and grams to pounds and ounces The program then performs the desired conversion. Have the user respond by typing the integer (1,2,3, or 4) for the desired type of conversion. The program reads the user's answer and then executes a selection statement. Each branch of this selection statement will be a function call. Thus, you will need in your program at least four (4) functions. Also, include a loop that lets the user repeat this computation for new input values until the user says he or she wants to end the program. If the user requests an invalid option, the program displays a message and generates the same menu again. The demo at the end will show you some sample runs, to give a better (visual) clarification. The four functions that you must include in your code are: void ImperialToMetricL(); // requests English length measure, converts to metric, outputs both void Metric TolmperialL(); // request metric length measure, converts to English, outputs both void ImperialToMetricW(); // requests English mass measure, converts to metric, outputs both void MetricTolmperialW(); // request metric mass measure, converts to English, outputs both As you can see from the description of the functions above, they (each) perform several tasks, namely request (read input), convert, and output. Feel free to implement these sub tasks in separate functions. Important Tips You must follow the tips below to get a full mark: 1. Use meaningful identifier names (don't use int x and double y) 2. Use comments throughout your code and explain the purpose of each function 3. Use functions and minimize the amount of coding in your main function. Create at least the four functions mentioned above Useful Hints The imperial system is also known as the English or British measurement system. There are 0.3048 meters in a foot, 100 centimeters in a meter, and 12 inches in a foot. Since the metric system can naturally handle meters and centimeters as a real number, you can define it as a double value. For example, 1.75 meters is equal to 1 meter and 75 centimeters. There are 0.4536 kilogram in a pound, 1000 grams in a kilogram, and 16 ounces in a pound. Since the metric system can naturally handle kilograms and grams as a real number, you can define it as a double value. For example, 1.75 kilograms is equal to 1 kilogram and 750 grams. To make the program easier, we will assume the user can insert ounces value that is higher than a pound. For example, 1 pound and 20 ounces is an acceptable input, even though there is only 16 ounces in a pound. The same is applicable to the feet and inches. When converting, you should be careful that 2 pounds and 5 ounces is not 2.5 pounds. Grade Distribution When you submit your program include the code, a short report that lists and explains what kind of variables and functions you used, and screen shots of your output. Don't forget, you have to submit both electronic and printed copy of your work in order to get a grade. The 10 points will be distributed as follows: Code: 7 Pts. o Comments, proper coding, variable naming and general layout (1 Pt.) o Properly displaying the menu and repeating it within a loop (2 Pts.) o Implementing the required Functions correctly. You must have the four functions listed below with the same name (4 Pts.): void ImperialToMetricl(); // requests imperial length measure, converts to metric, outputs both void Metric TolmperialL(); // request metric length measure, converts to imperial, outputs both void ImperialToMetricW(); // requests imperial mass measure, converts to metric, outputs both void Metric TolmperialW(); // request metric mass measure, converts to imperial, outputs both 44 Report (1.5 Pts.): Brief report that describes your approach in solving the problem, and lists what kind of variables and how many functions you used. Output Screenshots (1.5 Pts.): At least one large screenshot that shows 4 conversion options. Notice that all these grades are linked to your ability to orally explain your code. Each group member should expect to be asked to explain, modify and run his or her code. Failing to do so will result in a zero. Sample Run (user input in red) Welcome to my units conversion program Please choose one of the options below: 1. Convert Imperial to Metric Length 2. Convert Metric to Imperial Length 3. Convert Imperial to Metric Mass 4. Convert Metric to Imperial Mass Invalid option Y or y allows another choice of conversion. any other quits y Welcome to my units conversion program Please choose one of the options below: 1. Convert Imperial to Metric Length 2. Convert Metric to Imperial Length 3. Convert Imperial to Metric Mass 4. Convert Metric to Imperial Mass 1 Enter feet as an integer: 2 Enter inches as a double: 11 0.89 meters corresponds to 2 feet, 11.00 inches Y or y allows another choice of conversion. any other quits y Welcome to my units conversion program Please choose one of the options below: 1. Convert Imperial to Metric Length 2. Convert Metric to Imperial Length 3. Convert Imperial to Metric Mass 4. Convert Metric to Imperial Mass 2 Enter a number of meters as a double 1.75 1.75 meters corresponds to 5 feet, 8.90 inches Yor y allows another choice of conversion. any other quits y Welcome to my units conversion program Please choose one of the options below: 1. Convert Imperial to Metric Length 2. Convert Metric to Imperial Length 3. Convert Imperial to Metric Mass 4. Convert Metric to Imperial Mass 3 Enter pounds as an integer: 2 Enter ounces as a double: 4 1.02 kilograms corresponds to 2 pounds, 4.00 ounces Y or y allows another choice of conversion. any other quits y Welcome to my units conversion program Please choose one of the options below: 1. Convert Imperial to Metric Length 2. Convert Metric to Imperial Length 3. Convert Imperial to Metric Mass 4. Convert Metric to Imperial Mass 4 Enter a number of kilograms as a double 3.75 3.75 kilograms corresponds to 8 pounds, 4.28 ounces Y or y allows another choice of conversion. any other quits n Thank You!
Step by Step Solution
★★★★★
3.43 Rating (159 Votes )
There are 3 Steps involved in it
Step: 1
1 the required sourcecode is given below include using namespace std void Imperi...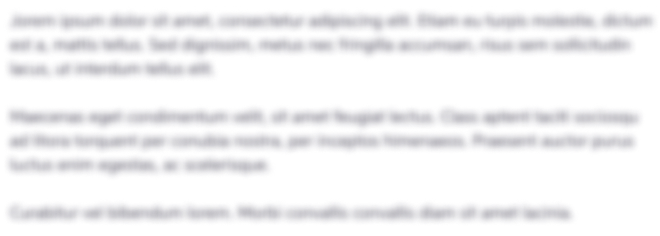
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started