Question
Write a C++ program that computes the value of a simple arithmetic expression consisting of two integer operands (range between 0 and 100, inclusive) along
Write a C++ program that computes the value of a simple arithmetic expression consisting of two integer operands (range between 0 and 100, inclusive) along with one of the standard arithmetic operators (such as +, -, *, / and %). Your program should input the operands as integers along with the operator as a character.
As a general outline, your program should:
prompt the user to enter the first operand
prompt the user to enter an arithmetic operator: +, -, *, / or %
prompt the user to enter the second operand
determine if the expression is valid or invalid according to the rules stated later in this document
compute the value of the expression if the expression is valid, and print out the value of the expression
if the expression is not valid, display a message stating that the expression is not valid as shown in later sample runs
------------------------------------------
Below is a sample run in interactive mode, e.g., running from Visual Studio. Items in red are typed in by the user. Notice the extra blank lines they are inserted when you hit the Enter key after each typed value.
Enter the first operand [0-100]: 5
Enter an arithmetic operator (+, -, *, / or %): +
Enter the second operand: 3
The value of the expression you entered is 8.00
------------------------------------------
In batch mode (e.g., what you get back from the Grader), the output would look like:
Enter the first operand [0-100]: 5
Enter an arithmetic operator (+, -, *, / or %): +
Enter the second operand: 3
The value of the expression you entered is 8.00
The red items are not shown from the Grader's output.
An invalid expression is defined as:
an expression consisting either or both operands out of the required range;
an expression that contains an operator other than +, -, *, /, %,
an expression in which division or modulus by 0 is attempted.
Requirements
As a minimal output and to receive credit for doing program 1, your program MUST get the correct output for test cases 1 - 8 (see below).
You must use two int type variables for the both input operands, -2 points if you don't, a char variable for your input operator and a float (not double) for the output variable.
You must use type casting when performing division (/) operation in order to get the precise result. Make sure that you are not doing integer divisions.
You MUST follow the programming ground rules!
You should thoroughly test your program prior to submitting it using a variety of inputs. Loops, arrays and functions are NOT allowed in this program. You will lose two points if you violate this requirement.
See the input and output samples for exact wordings for the prompts and outputs. You must match the output exactly.
If you need help, see a PAL or a lab assistant or your instructor.
You are allowed at most 30 submittals to the Grader and will lose one point for each additional submittal.
You are NOT allowed to use an else statement, -3 points if you do. You can use multiple if statements, some with compound conditions.
At the top of your main program, be sure to include the following to format the output consistently:
cout << fixed << showpoint << setprecision(2);
The output for % operation should be a whole number, without showing the decimal point, to undo the output format of the above, use the following before output:
cout << resetiosflags(ios::fixed | ios::showpoint);
Examples
Below are 8 sample runs in interactive mode:
Sample run #1:
Enter the first operand [0-100]: 5
Enter an arithmetic operator (+, -, *, / or %): +
Enter the second operand [0-100]: 3
The value of the expression is 8.00
Sample run #2:
Enter the first operand [0-100]: 5
Enter an arithmetic operator (+, -, *, / or %): -
Enter the second operand [0-100]: 8
The value of the expression is -3.00
Sample run #3:
Enter the first operand [0-100]: 11
Enter an arithmetic operator (+, -, *, / or %): *
Enter the second operand [0-100]: 5
The value of the expression is 55.00
Sample run #4:
Enter the first operand [0-100]: 99
Enter an arithmetic operator (+, -, *, / or %): /
Enter the second operand [0-100]: 5
The value of the expression is 19.80
Sample run #5:
Enter the first operand [0-100]: 33
Enter an arithmetic operator (+, -, *, / or %): %
Enter the second operand [0-100]: 7
The value of the expression is 5
Sample run #6:
Enter the first operand [0-100]: -20
Enter an arithmetic operator (+, -, *, / or %): *
Enter the second operand [0-100]: 2
The expression that you entered is not valid.
Sample run #7:
Enter the first operand [0-100]: 8
Enter an arithmetic operator (+, -, *, / or %): /
Enter the second operand [0-100]: 0
The expression that you entered is not valid.
Sample run #8:
Enter the first operand [0-100]: 10
Enter an arithmetic operator (+, -, *, / or %): |
Enter the second operand [0-100]: 6
The expression that you entered is not valid.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
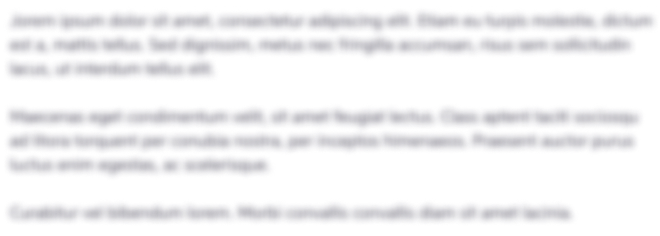
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started