Question
Write a C++ program that uses while loops to perform the following steps: a. Prompt the user to input two positive integers. Variables: firstNum and
Write a C++ program that uses while loops to perform the following steps:
a. Prompt the user to input two positive integers. Variables: firstNum and secondNum (firstNum must be less than secondNum) (use while loop); create a user-defined function called validateUserInput() to validate the user's input. Use Call-by-Value. validateUserInput() is a value returning function.
b. Output all odd numbers between firstNum and secondNum. (use while loop); create a user-defined function called oddNumbers(). Use Call-by-Value. oddNumbers() is a void function.
c. Output the sum of all even numbers between firstNum and secondNum. (use while loop); create a user-defined function called sumEvenNumbers(). Use Call-by-Value. Declare a variable called sumEven in the main() for the sumEvenNumbers(). sumEvenNumbers() is a value returning function. Use sumEven to hold a returned value.
d. Output the numbers and their squares between 1 and 10. (use while loop): create a user-defined function called displaySquareNumbers(). Call-by-Value. displaySquareNumbers() is a void function.
e. Output the sum of the square of the odd numbers between firstNum and secondNum. (use while loop); create a user-defined function called sumSqureOddNumbers(). Use Call-by-Value. Declare a variable called sumSquareOdd in the main(), for the sumSqureOddNumbers(). sumSqureOddNumbers() is a value returning function. Use sumSquareOdd to hold a returned value.
f. Output all uppercase letters. (use while loop); create a user-defined function called displayUppercaseLetters(). Use Call-by-Value. displayUppercaseLetters() is a void function.
Follow Program Layout Exactly!!!
int main()
{
//a
validateUserInput();
//b
oddNumbers();
//c
sumEvenNumbers();
//d
displaySquareNumbers();
//e
sumSqureOddNumbers();
//f
displayUppercaseLetters();
}
***Collect the user's inputs in the main() and pass them to the other functions.
***Per item d and f, make sure you pass a value to the functions from main().
***Validate the user's inputs; in case the user types characters.
***Allow the user to use upper case or lower case.
***Allow the user to repeat the program. (use while loop).
***No for loop(s) and do while loop(s).
***Do not use any pre-defined functions that were not covered in the class.
***Use only topics that were covered in the class.
***No object-oriented programming.
***Match the output below (OUTPUTS section).
Submit your source code (file name is Assignment_11_yourLastName.cpp). Make sure that it compiles using Code::Blocks.
If it doesn't compile, you will lose 50%. Improper indentation: -20%
If it doesn't produce the required results: -30%. Formatting output: -20%
Do NOT compress the file.
**************************************************************************************
MATCH OUTPUTS EXACTLY:!!!
**************************************************************************************
Enter two positive integer numbers. First number must be less than the second number: Enter numbers: 8 a
Incorrect Input. Please try again.
Enter two positive integer numbers. First number must be less than the second number: Enter numbers:
**************************************************************************************
Enter two positive integer numbers. First number must be less than the second number: Enter numbers: a 8
Incorrect Input. Please try again.
Enter two positive integer numbers. First number must be less than the second number: Enter numbers:
**************************************************************************************
Enter two positive integer numbers. First number must be less than the second number: Enter numbers: 8 2
First number must be less than the second number! Please try again.
Enter two positive integer numbers. First number must be less than the second number: Enter numbers:
**************************************************************************************
Enter two positive integer numbers. First number must be less than the second number: Enter numbers: -2 8
No negative numbers! Please try again.
Enter two positive integer numbers. First number must be less than the second number: Enter numbers:
**************************************************************************************
Enter two positive integer numbers. First number must be less than the second number you enter Enter numbers: 2 8
Odd integers between 2 and 8 are: 3 5 7
Sum of even integers between 2 and 8 = 20
Number Square of Number 1 1 2 4 3 9 4 16 5 25 6 36 7 49 8 64 9 81 10 100
Sum of the squares of odd integers between 2 and 8 = 83
Upper case letters are: A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
Do you want to repeat this program? y/n > y
**************************************************************************************
Enter two positive integer numbers. First number must be less than the second number you enter Enter numbers: 1 9
Odd integers between 1 and 9 are: 1 3 5 7 9
Sum of even integers between 1 and 9 = 20
Number Square of Number 1 1 2 4 3 9 4 16 5 25 6 36 7 49 8 64 9 81 10 100
Sum of the squares of odd integers between 1 and 9 = 165
Upper case letters are: A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
Do you want to repeat this program? y/n > y
**************************************************************************************
Enter two positive integer numbers. First number must be less than the second number you enter Enter numbers: 11 15
Odd integers between 11 and 15 are: 11 13 15
Sum of even integers between 11 and 15 = 26
Number Square of Number 1 1 2 4 3 9 4 16 5 25 6 36 7 49 8 64 9 81 10 100
Sum of the squares of odd integers between 11 and 15 = 515
Upper case letters are: A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
Do you want to repeat this program? y/n > n
Step by Step Solution
There are 3 Steps involved in it
Step: 1
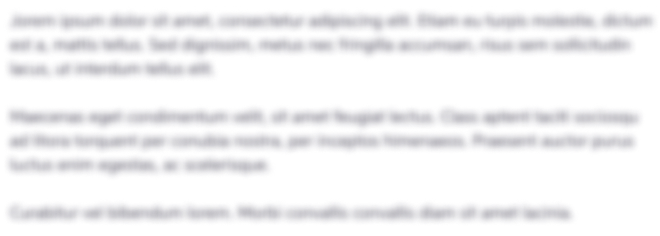
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started