Question
Write a C++ program that will create a list of axis-aligned rectangles defined in a 2D space, i.e. the x-y space. An axis-aligned rectangle has
Write a C++ program that will create a list of axis-aligned rectangles defined in a 2D space,
i.e. the x-y space. An axis-aligned rectangle has two vertical sides (left and right) that
are parallel to the y-axis and two horizontal sides (top and bottom) that are parallel to
the x-axis.
Each rectangle is defined by the(x, y) location of its bottom left corner, its length (along the x-axis),
and its height (along the y-axis). In your program, each rectangle will also have its own unique name. After
the description of each rectangle is input from the user and stored in a list (vector of class
Rectangle), your program will compute each rectangle's area, perimeter, and midpoint. In
addition, you will scale each rectangle by a factor of 2 about its midpoint. Please use/complete the template
#include#include #include using namespace std; class Point { private: double px; double py; public: void setX(const double x); void setY(const double y); double getX() const; double getY() const; }; class Rectangle { private: string name; Point blPoint; double length, height; public: // member functions void setName(const string & inName); void setBottomLeft(const double x, const double y); void setDimensions(const double inLength, const double inHeight); string getName() const; Point getBottomLeft() const; double getLength() const; double getHeight() const; double area() const; double perimeter() const; Point midPoint() const; void scaleBy2(); void display() const; }; // FUNCTION PROTOTYPES GO HERE: void display_banner(); bool read_valid_name(const string & prompt, const string & errMessage, const string & errDuplicate, string & name, const vector & nameList); void read_point(const string & prompt, double & x, double & y); void read_dimensions(const string & prompt, double & length, double & height); void add_rec(const string & name, const double & x, const double & y, const double & length, const double & height, vector & list); void display_rectangles(vector & list); // MAIN FUNCTION GO HERE int main() {
// Define your local variables, e.g. a vector of class Rectangle
// Display welcome banner
/* Prompt user for first rectangle or 'stop' */
// WHILE user input is invalid // Display "Try again! "
// IF user input is not 'stop' // Extract rectangle name from user input // Prompt for bottom left point // Prompt for length and height // Add rectangle to the rectangle list /* Prompt user for next rectangle or 'stop' */ // WHILE user input is not 'stop' // Display "Thank you! "
// WHILE user input is invalid // Display "Try again! " // IF user input is not 'stop' // Extract rectangle name from user input // Prompt for bottom left point // Prompt for length and height // Add rectangle to the rectangle list
// IF the rectangle list is not empty // Display all rectangles in the rectangle list // ELSE // Display that no rectangles are in the list
} // FUNCTION DEFINITIONS GO HERE: // CLASS MEMBER FUNCTION DEFINITIONS GO HERE:
The following is a sample output of the solution.exe file, with two purposefully included errors:
Welcome! Create your own list of rectangles. You will be asked to provide information about each rectangle in your list by name. Type the word 'stop' for the rectangle name when you are done.
Enter the name of the first rectangle: test Invalid input. Type 'rec' followed by the name or 'stop' if done. Try again! Enter the name of the first rectangle: rec test Enter test's bottom left x and y coords: 10 20 Enter test's length and height: 5 5
Thank you! Enter the name of the next rectangle: test 2 Invalid input. Type 'rec' followed by the name or 'stop' if done. Try again! Enter the name of the next rectangle: rec test 2 Enter test 2's bottom left x and y coords: 20 30 Enter test 2's length and height: 10 10
Thank you! Enter the name of the next rectangle: stop
You have 2 rectangle(s) in your list:
Rectangle 'test': Location is (10, 20), Length is 5, Height is 5; Area is 25, Perimeter is 20, Midpoint is located at (12.5, 22.5) After scale by 3: Location is (5, 15), Length is 15, Height is 15; Area is 225, Perimeter is 60, Midpoint is located at (12.5, 22.5)
Rectangle 'test 2': Location is (20, 30), Length is 10, Height is 10; Area is 100, Perimeter is 40, Midpoint is located at (25, 35) After scale by 3: Location is (10, 20), Length is 30, Height is 30; Area is 900, Perimeter is 120, Midpoint is located at (25, 35)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
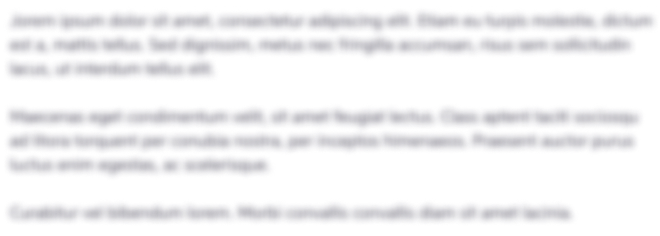
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started