Question
write a C++ program to read two distances for each face, compute the ratios, and then determine which two images have the closest ratio. this
write a C++ program to read two distances for each face, compute the ratios, and then determine which two images have the closest ratio.
this code is in C I need to convert it to C++
#include
#include
int main(void)
{
/* Declare variables. */
double eyes_1, eyes_2, eyes_3, nose_chin_1, nose_chin_2,
nose_chin_3, ratio_1, ratio_2, ratio_3, diff_1_2,
diff_2_3, diff_1_3;
/* Get user input from the keyboard. */
printf("Enter values in cm. ");
printf("Enter eye distance and nose-chin distance for image 1: ");
scanf("%lf %lf",&eyes_1,&nose_chin_1);
printf("Enter eye distance and nose-chin distance for image 2: ");
scanf("%lf %lf",&eyes_2,&nose_chin_2);
printf("Enter eye distance and nose-chin distance for image 3: ");
scanf("%lf %lf",&eyes_3,&nose_chin_3);
/* Compute ratios. */
ratio_1 = eyes_1/nose_chin_1;
ratio_2 = eyes_2/nose_chin_2;
ratio_3 = eyes_3/nose_chin_3;
/* Compute differences. */
diff_1_2 = fabs(ratio_1 ratio_2);
diff_1_3 = fabs(ratio_1 ratio_3);
diff_2_3 = fabs(ratio_2 ratio_3);
/* Find minimum difference and print image numbers. */
if ((diff_1_2 <= diff_1_3)) && (diff_1_2 <= diff_2_3)
printf("Best match is between images 1 and 2 ");
if ((diff_1_3 <= diff_1_2)) && (diff_1_3 <= diff_2_3)
printf("Best match is between images 1 and 3 ");
if ((diff_2_3 <= diff_1_3)) && (diff_2_3 <= diff_1_2)
printf("Best match is between images 2 and 3 ");
/* Exit program. */
return 0;
}
/**/
5. Testing
We first test the program with the data from the hand example. This generates the following interaction:
Enter values in cm.
Enter eye distance and nose-chin distance for image 1: 5.7 5.3
Enter eye distance and nose-chin distance for image 2: 6.0 5.0
Enter eye distance and nose-chin distance for image 3: 6.0 5.6
Best match is between images 1 and 3
-----------------------------------------------------------------------------------------------------------------------------------------------------
1-Modify the program so that it also prints out all three differences. This gives additional information about the quality of the match; if the differences are small, the quality of the match is better.
2-What would the output be if two of the ratios were the same minimum value? What would the output be if all three of the ratios were the same value? Generate data to make these cases happen and check your answers.
3-Modify the program to use a nested if statement in the solution instead of three independent if statements. Does the answer to Problem 2 change with this solution?
4-Modify the program to print the image numbers for the two images that are the most different.
5-Modify the program to print the best match between four images. Note that this causes the program to get longer, because with four images there are six possibilities.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
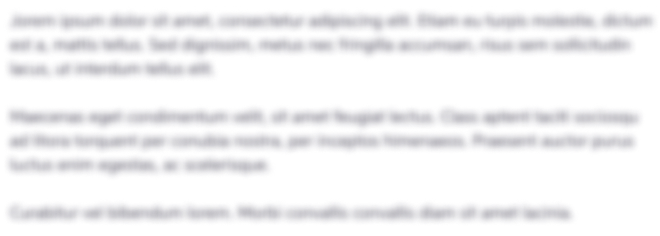
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started