Question
Write a class ArrayBag, which includes the following operations: -Get the current number of entries in this bag. -See whether this bag is empty. -Add
Write a class ArrayBag, which includes the following operations:
-Get the current number of entries in this bag.
-See whether this bag is empty.
-Add a new entry to this bag.
-Remove one unspecified entry from this bag, if possible.
-Remove all occurrences of a given entry from this bag.
-Remove all entries from this bag. */
-Count the number of times a given entry appears in this bag.
-Test whether this bag contains a given entry.
-Retrieve all entries that are in this bag
The bag specification "BagInterface.java" and a test driver "ArrayBagTest.java" are given. Please read them carefully and complete your class implementation "ArrayBag.java".
CODES:
ArrayBag:
/** * */ /** * @author gaok * */ public final class ArrayBagimplements BagInterface { private final T[] bag; private int numberOfEntries; private static final int DEFAULT_CAPACITY=25; /** Creates an empty bag whose initial capacity is 25. */ public ArrayBag() { this (DEFAULT_CAPACITY); } // end default constructor /** Creates an empty bag having a given capacity. @param desiredCapacity The integer capacity desired. */ public ArrayBag(int desiredCapacity) { bag=(T[]) new Object[desiredCapacity]; numberOfEntries=0; } // end constructor @Override public int getCurrentSize() { // TODO Auto-generated method stub return 0; } @Override public boolean isEmpty() { // TODO Auto-generated method stub return false; } @Override public boolean add(T newEntry) { // TODO Auto-generated method stub return false; } @Override public T remove() { // TODO Auto-generated method stub return null; } @Override public boolean remove(T anEntry) { // TODO Auto-generated method stub return false; } @Override public void clear() { // TODO Auto-generated method stub } @Override public int getFrequencyOf(T anEntry) { // TODO Auto-generated method stub return 0; } @Override public boolean contains(T anEntry) { // TODO Auto-generated method stub return false; } @Override public T[] toArray() { // TODO Auto-generated method stub return null; } }
ArrayBagTest:
/** * A test of the constructors and the methods add, toArray, isEmpty, getCurrentSize, * getFrequencyOf, and contains for the class ArrayBag. */ public class ArrayBagTest { public static void main(String[] args) { String[] contentsOfBag = {"One", "One", "Two", "One", "Three", "One"}; // Tests on an empty bag BagInterfaceaBag = new ArrayBag<>(contentsOfBag.length); System.out.println("Testing an initially empty bag:"); System.out.println("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~"); testIsEmpty(aBag, true); String[] testStrings1 = {"", "Two"}; testFrequency(aBag, testStrings1); testContains(aBag, testStrings1); testRemove(aBag, testStrings1); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println(); // Adding strings System.out.println("Adding " + contentsOfBag.length + " strings to an initially empty bag with" + " the capacity to hold more than " + contentsOfBag.length + " strings:"); System.out.println("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~"); testAdd(aBag, contentsOfBag); // Tests on a bag that is not empty testIsEmpty(aBag, false); String[] testStrings2 = {"One", "Two", "Three", "Four", "XXX"}; testFrequency(aBag, testStrings2); testContains(aBag, testStrings2); // Removing strings String[] testStrings3 = {"", "Two", "One", "Three", "XXX"}; testRemove(aBag, testStrings3); System.out.println(" Clearing the bag:"); aBag.clear(); testIsEmpty(aBag, true); displayBag(aBag); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println(); // Filling an initially empty bag to capacity System.out.println(" Testing an initially empty bag that " + " will be filled to capacity:"); System.out.println("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~"); aBag = new ArrayBag (7); String[] contentsOfBag2 = {"One", "Two", "One", "Three", "Two", "Three", "Four"}; testAdd(aBag, contentsOfBag2); System.out.println("Try to add XXX to the full bag:"); if (aBag.add("XXX")) System.out.println("Added a string beyond the bag's capacity: ERROR!"); else System.out.println("The method add cannot add another string: OK"); } // end main // Tests the method add. private static void testAdd(BagInterface aBag, String[] content) { System.out.print("Adding "); for (int index = 0; index < content.length; index++) { aBag.add(content[index]); System.out.print(content[index] + " "); } // end for System.out.println(); displayBag(aBag); } // end testAdd // Tests the two remove methods. private static void testRemove(BagInterface aBag, String[] tests) { for (int index = 0; index < tests.length; index++) { String aString = tests[index]; if (aString.equals("") || (aString == null)) { // test remove() System.out.println(" Removing a string from the bag:"); String removedString = aBag.remove(); System.out.println("remove() returns " + removedString); } else { // test remove(aString) System.out.println(" Removing \"" + aString + "\" from the bag:"); boolean result = aBag.remove(aString); System.out.println("remove(\"" + aString + "\") returns " + result); } // end if displayBag(aBag); } // end for } // end testRemove // Tests the method isEmpty. // correctResult indicates what isEmpty should return. private static void testIsEmpty(BagInterface aBag, boolean correctResult) { System.out.print("Testing isEmpty with "); if (correctResult) System.out.println("an empty bag:"); else System.out.println("a bag that is not empty:"); System.out.print("isEmpty finds the bag "); if (correctResult && aBag.isEmpty()) System.out.println("empty: OK."); else if (correctResult) System.out.println("not empty, but it is empty: ERROR."); else if (!correctResult && aBag.isEmpty()) System.out.println("empty, but it is not empty: ERROR."); else System.out.println("not empty: OK."); System.out.println(); } // end testIsEmpty // Tests the method getFrequencyOf. private static void testFrequency(BagInterface aBag, String[] tests) { System.out.println(" Testing the method getFrequencyOf:"); for (int index = 0; index < tests.length; index++) { String aString = tests[index]; if (!aString.equals("") && (aString != null)) { System.out.println("In this bag, the count of " + tests[index] + " is " + aBag.getFrequencyOf(tests[index])); } // end if } // end for } // end testFrequency // Tests the method contains. private static void testContains(BagInterface aBag, String[] tests) { System.out.println(" Testing the method contains:"); for (int index = 0; index < tests.length; index++) { String aString = tests[index]; if (!aString.equals("") && (aString != null)) { System.out.println("Does this bag contain " + tests[index] + "? " + aBag.contains(tests[index])); } // end if } // end for } // end testContains // Tests the method toArray while displaying the bag. private static void displayBag(BagInterface aBag) { System.out.println("The bag contains " + aBag.getCurrentSize() + " string(s), as follows:"); Object[] bagArray = aBag.toArray(); for (int index = 0; index < bagArray.length; index++) { System.out.print(bagArray[index] + " "); } // end for System.out.println(); } // end displayBag } // end ArrayBagTest
BagInterface:
/** An interface that describes the operations of a set of objects * This interface will be used for both Project 2 and Project 3*/ public interface BagInterface{ /** Gets the current number of entries in this bag. @return The number of entries currently in the bag. */ public int getCurrentSize(); /** Sees whether this bag is empty. @return True if the bag is empty, or false if not. */ public boolean isEmpty(); /** Adds a new entry to this bag. @param newEntry The object to be added as a new entry. @return True if the addition is successful, or false if not. */ public boolean add(T newEntry); /** Removes one unspecified entry from this bag, if possible. @return Either the removed entry, if the removal was successful, or null. */ public T remove(); /** Removes all occurrences of a given entry from this bag. @param anEntry The entry to be removed. @return True if the removal was successful, or false if not. */ public boolean remove(T anEntry); /** Removes all entries from this bag. */ public void clear(); /** Counts the number of times a given entry appears in this bag. @param anEntry The entry to be counted. @return The number of times anEntry appears in the bag. */ public int getFrequencyOf(T anEntry); /** Tests whether this bag contains a given entry. @param anEntry The entry to locate. @return True if the bag contains anEntry, or false if not. */ public boolean contains(T anEntry); /** Retrieves all entries that are in this bag. @return A newly allocated array of all the entries in the bag. Note: If the bag is empty, the returned array is empty. */ public T[] toArray();
EXAMPLE OF OUTPUT:
Testing an initially empty bag: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Testing isEmpty with an empty bag: isEmpty finds the bag empty: OK.
Testing the method getFrequencyOf: In this bag, the count of Two is 0
Testing the method contains: Does this bag contain Two? false
Removing a string from the bag: remove() returns null The bag contains 0 string(s), as follows:
Removing "Two" from the bag: remove("Two") returns false The bag contains 0 string(s), as follows:
+++++++++++++++++++++++++++++++++++++++++++++++++++
Adding 6 strings to an initially empty bag with the capacity to hold more than 6 strings: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Adding One One Two One Three One The bag contains 6 string(s), as follows: One One Two One Three One Testing isEmpty with a bag that is not empty: isEmpty finds the bag not empty: OK.
Testing the method getFrequencyOf: In this bag, the count of One is 4 In this bag, the count of Two is 1 In this bag, the count of Three is 1 In this bag, the count of Four is 0 In this bag, the count of XXX is 0
Testing the method contains: Does this bag contain One? true Does this bag contain Two? true Does this bag contain Three? true Does this bag contain Four? false Does this bag contain XXX? false
Removing a string from the bag: remove() returns One The bag contains 5 string(s), as follows: One One Two One Three
Removing "Two" from the bag: remove("Two") returns true The bag contains 4 string(s), as follows: One One Three One
Removing "One" from the bag: remove("One") returns true The bag contains 1 string(s), as follows: Three
Removing "Three" from the bag: remove("Three") returns true The bag contains 0 string(s), as follows:
Removing "XXX" from the bag: remove("XXX") returns false The bag contains 0 string(s), as follows:
Clearing the bag: Testing isEmpty with an empty bag: isEmpty finds the bag empty: OK.
The bag contains 0 string(s), as follows:
+++++++++++++++++++++++++++++++++++++++++++++++++++
Testing an initially empty bag that will be filled to capacity: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Adding One Two One Three Two Three Four The bag contains 7 string(s), as follows: One Two One Three Two Three Four Try to add XXX to the full bag: The method add cannot add another string: OK
A framework of the class implementation is given in the attached "ArrayBag.java". But you need to work out for those 9 class functi
Step by Step Solution
There are 3 Steps involved in it
Step: 1
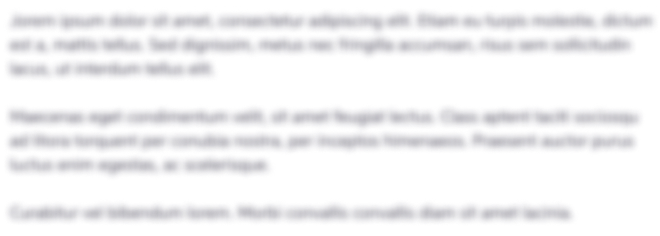
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started