Question
Write a class Assignment that has private attributes for Name, Possible Points and Earned Points Write a class Student that has private attributes for name
Write a class Assignment that has private attributes for Name, Possible Points and Earned Points
Write a class Student that has private attributes for name and a vector of assignments. The constructor method should require a name. Add a method for get total score that returns the total number of points earned divided by the total number of possible points for their assignments.
Write a class Gradebook that has a vector of students and methods for print highest scoring student, that prints the student with the highest score, print lowest scoring student, print class average, add student ( accept a student reference to add ), score student ( that asks for a student name and prompts for scores for their assignments ), add assignment ( that adds an assignment with a 0 score for all students if there already isn\'t an assignment with that name ), print student list summary ( print the list of students and total score), print student detail ( print the list of students and every assignment score ).
Allow the user to Save ( write a file ) or Load ( read a file ) a gradebook.
Add a main method that has a looping menu allowing them to Add Students to the Gradebook, Add Assignments to the Gradebook, and all the other Gradebook functions.
This is what i have so far:
#include <fstream>
#include <iostream>
#include <iomanip>
#include <cstring>
#include <vector>
using namespace std;
class Assignment
{
private:
string name;
int possiblePoints;
int earnedPOints;
public:
Assignment(string name,int possible,int earned)
{
this->name=name;
this->possiblePoints=possible;
this->earnedPOints=earned;
}
string getName()
{
return name;
}
int getPossiblePoints()
{
return possiblePoints;
}
int getEarnedPOints()
{
return earnedPOints;
}
void setName(string name)
{
this-> name = name;
}
void setPossiblePoints(int possible)
{
this-> possiblePoints = possible;
}
void setEarnedPOints(int earned)
{
this-> earnedPOints = earned;
}
};
class Student
{
private:
string name;
vector<Assignment> vec;
public:
Student(string name)
{
this-> name = name;
}
double getTotalScore()
{
int totPossible = 0,totEarned = 0;
for ( int i = 0; i < vec.size(); i++ )
{
totPossible += vec[i].getPossiblePoints();
totEarned += vec[i].getEarnedPOints();
}
return ( ( double ) totEarned / totPossible ) * 100;
}
string getName()
{
return name;
}
vector<Assignment> getStudentAssignments()
{
return vec;
}
int getSize()
{
return vec.size();
}
};
class GradeBook
{
private:
vector<Student> students;
public:
GradeBook()
{
}
void displayHighestScoreStudent()
{
double max = students[0].getTotalScore();
int indx = 0;
string name;
for ( int i = 0; i,students.size(); i++ )
{
if ( max < students[1].getTotalScore() )
{
max = students[i].getTotalScore();
indx = i;
}
}
cout << students[indx].getName() << " got the highest score " << max << endl;
}
void displayLowestScoreStudent()
{
double min = students[0].getTotalScore();
int indx = 0;
string name;
for ( int i = 0; i,students.size(); i++ )
{
if ( min > students[1].getTotalScore() )
{
min = students[i].getTotalScore();
indx = i;
}
}
cout << students[indx].getName() << " got the lowest score " << min << endl;
}
void displayClassAverage()
{
double tot = 0;
for ( int i = 0; i < students.size(); i++)
{
tot += students[i].getTotalScore();
}
cout << "Class Average :" << tot / students.size() << endl;
}
void addStudent(Student std)
{
students.push_back(std);
}
void printStudentList()
{
cout << endl << "Displaying the Student List#" << endl;
cout << setw(25) << left << "Name" << setw(8) << right << "Score" << endl;
for ( int i = 0; i < students.size(); i++ )
{
cout << setw(25) << left << students[i].getName() << setw(8) << right << students[i].getTotalScore() << endl;
}
}
void printStudentDetail()
{
for ( int i = 0; i < students.size(); i++ )
{
cout << students[i].getName() << endl;
for ( int j = 0; j < students[i].getSize(); i++)
{
vector<Assignment> vec1 = students[i].getStudentAssignments();
cout << vec1[i].getPossiblePoints() << "\\t" << vec1[i].getEarnedPOints() << endl;
}
}
}
};
int main()
{
int loop = 1;
while (loop != 0)
{
cout << "If you are done enter 0" << endl;
cin >> loop;
}
return 0;
}
Step by Step Solution
3.50 Rating (153 Votes )
There are 3 Steps involved in it
Step: 1
gradebookcpp include include include include include include include using namespace std class Assignment private string name int possiblePoints int earnedPoints public Assignmentstring nameint possib...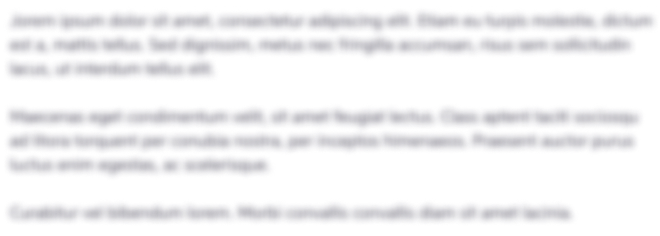
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started