Question
Write a class called Movie that will store some basic information about a certain movie. It should have a field for the title, the year
Write a class called Movie that will store some basic information about a certain movie. It should have a field for the title, the year it was released, and a rating between 0 and 10. The class should have the following methods.
// Fields
private String title;
private int releaseYear;
private double rating;
// Inside of the constructor verify that the parameters are valid.
// The title shouldn't be an empty string, the releaseYear can't
// be in the future, and the rating has to be between 0 and 10. If
// any of the parameters are invalid, then print out an error to the console
public Movie(String title, int releaseYear, double rating)
// This should return a string that represents this movie object.
// It should be in the following format: TITLE (YEAR), RATING out of 10
public String toString()
// This class should also include getters for all of the fields.
Next, using the Movie class, create a MovieDatabase class that will handle any number of movies. The movies inside the MovieDatabase should be stored as an array. The MovieDatabase class should have the following methods:
// Fields
private Movie[] movies;
// For this constructor set the Movie array equal to the one passed in
public MovieDatabase(Movie[] m)
// Returns the highest rated movie
public Movie getHighestRatedMovie()
// Returns the newest movie (by year)
public Movie getNewestMovie()
// Returns the Movie at the index passed in. If the index is invalid,
// then return null.
public Movie getMovieAt(int index)
// Returns the number of movies in the database
public int getMovieCount()
Then, create a tester class which uses the array below. Create a MovieDatabase object which uses that array. Finally, test all the methods from the MovieDatabase class.
Movie[] test =
{
new Movie("The Dark Knight", 2008, 8.9),
new Movie("Fight Club", 1999, 8.8),
new Movie("12 Angry Men", 1957, 8.9),
new Movie("The Godfather", 1972, 9.2),
new Movie("The Lord of the Rings: The Return of the King", 2003, 8.9),
new Movie("The Godfather: Part II", 1974, 9.0),
new Movie("Pulp Fiction", 1994, 8.9),
new Movie("Schindler's List", 1993, 8.9),
new Movie("The Shawshank Redemption", 1994, 9.2),
new Movie("The Good, the Bad and the Ugly", 1966, 8.9)
};
Write in Java and at a simple level
Step by Step Solution
There are 3 Steps involved in it
Step: 1
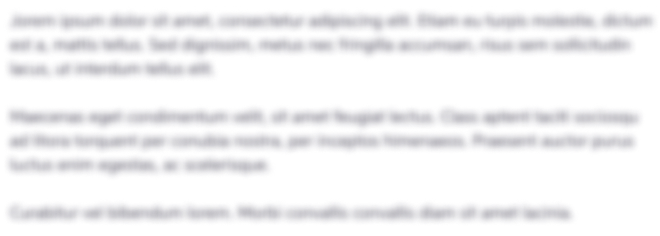
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started