Question
Write a class CuteCreature, such that each CuteCreature object has the following instance variables: Species Level a positive integer that indicates how powerful the creature
Write a class CuteCreature, such that each CuteCreature object has the following instance variables: Species
Level a positive integer that indicates how powerful the creature is
Current hit points a measure of how much damage the creature can take before being incapacitated
Maximum hit points
Attack damage a measure of how much damage this creature inflicts when it attacks another creature
Experience points a measure of how close the creature is to leveling up and becoming more powerful
Experience value how many experience points this creature is worth when defeated by another creature
isSpecial this is a boolean variable that determines whether this creature is considered special. Internally, special creatures are exactly the same as non-special creatures. However, they have a slightly different appearance and are somehow very highly prized by the players of your game.
CuteCreature should include at least the methods below. Feel free to add other methods if you feel its necessary. A constructor that allows you to specify the species, maximum hit points, attack damage, experience value, and special status when a CuteCreature object is first created. A newly created CuteCreature should have a level of 1, current hit points equal to the maximum hit points, and zero experience points. public void takeDamage(int dmg) This method allows the CuteCreature to take the specified amount of damage to its current hit points. Negative hit points are not allowed, so this method should ensure that the current hit points cannot go below zero. This method should also display some text when called, to indicate the amount of damage taken. If the damage is enough to bring the current hit points to zero, display some text to indicate that the CuteCreature has been incapacitated. private void levelUp() This method allows the CuteCreature to level up. Leveling up increases the creatures level by 1, in addition to the following changes: o If the new level is between 2-10 (inclusive): Current and maximum hit points increase by 4, attack damage increases by 3 o If the new level is 11 or over: Current and maximum hit points increase by 1, attack damage increases by 1 o For all level ups: Experience value increases by 10 levelUp is declared private since its meant to be called only from this classs gainExp method below. This method should also display some text when called, to indicate that the creature is leveling up. public void gainExp(int exp) This method allows the CuteCreature to gain the specified number of experience points, leveling up if necessary. Note that more than one level may be gained from a single call of this method, if a sufficiently large argument is provided! Assume that 250 experience points are required to advance from level 1 to level 2, and the experience per level increases by 50 for each level thereafter. To illustrate how this works, heres a chart of the experience needed for the first several levels: Current Level Experience Points Needed for Next Level Total Experience Points Accumulated at Next Level 1 250 250 2 300 550 3 350 900 4 400 1300 5 450 1750 This method should also display some text when called, to indicate the amount of experience gained. public void attack(CuteCreature c) This method allows the calling CuteCreature to make a single attack against another CuteCreature. Assume the attacking creature has a 80% chance to hit with the attack, a 5% chance to score a critical hit, and a 15% chance of missing altogether. If it hits, the attack damages the target creature by a random amount within 20% of the attack damage of the attacking creature. For example, if the attacking creature has an attack damage of 10, a hit could do anywhere between 8-12 damage to the target. A critical hit doubles the usual damage. If it misses, nothing happens. If the target creature is defeated (i.e, has its current hit points brought down to zero) by the attack, the method should also make the attacking creature gain the appropriate amount of experience. This method should also display some text when called, to indicate which creature is attacking which and the results of that attack. A toString method that shows all the instance variables of the CuteCreature. For example, if you make a new CuteCreature with the code CuteCreature c = new CuteCreature("Bowlbasore", 40, 5, 70, true); then calling System.out.println(c); might result in something like Level 1 Bowlbasore ------------------ *** Special! *** HP: 40/40 Attack Dmg: 5 XP: 0/250 XP Value: 70
Step by Step Solution
There are 3 Steps involved in it
Step: 1
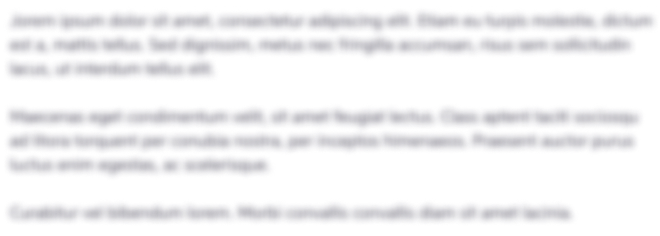
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started