Question
write a code in either matlab or c++ for this assignment. Problems In this lab, we will use MATLAB to implement primality tests, namely Fermats
write a code in either matlab or c++ for this assignment. Problems In this lab, we will use MATLAB to implement primality tests, namely Fermats little theorem and Wilsons Theorem. We will also implement Fermats Last Theorem. 1. Wilsons Theorem (25 points) Wilsons theorem states that a number p is prime if and only if the remainder of (p ? 1)! + 1 p is 0. Implement Wilsons Theorem to determine whether a number is prime or not. Hint: In MATLAB, when you receive your input variable, p, use the following statement p = uint64(p) to convert p from a double to an unsigned integer that ranges from 0 to 18,446,744,073,709,551,61. 2. Fermats Little Theorem (25 points) Fermats little theorem states that a number p is prime if for all numbers a such that 1 ? a < p, the remainder of a p?1 ? 1 p is 0. Implement Fermats Little Theorem. Hint: As done in Problem 1. 3. Speed Test (25 points) Use MATLABs tic and toc function to determine the speed of determining whether a number p is prime or not using MATLABs built-in isprime, MATLABs built-in factor function, Wilsons Theorem, and Fermats Little Theorem. Display the times of each function. 4. Fermats Last Theorem (25 points) Fermats Last Theorem states that no three positive integers a,b, and c can satisfy the equation a n + b n = c n for any integer value of n greater than two (From Wikipedia). For n = 2, we have the Pythagorean triples such as {3, 4, 5} where 32 + 42 = 52 . In MATLAB, empirically verify Fermats Last Theorem for bounded values of a, b, and c as seen below: ? ? 1 5 2 6 3 5 ? ? where a is the set of integers from 1 to 5, b is the set of integers from 2 to 6, and c is the set of integers from 3 to 5. To receive input from the user as a matrix, use the code below: >> data = str2num(input('Enter the bounds for a, b, and c: ', 's')); Enter the bounds for a, b, and c: 1 5; 2 6; 3 5 will save the matrix seen above in data. Also, take as input from the user the value of n. If the user inputs 2 for n, a sample output is given below: a a 2 b b 2 a 2 + b 2 c c 2 Equal? 1 1 2 4 5 3 9 False ... 3 9 4 16 25 5 25 True ... 5 25 6 36 61 5 25 False
Step by Step Solution
There are 3 Steps involved in it
Step: 1
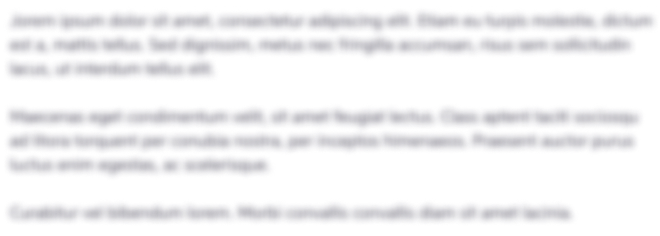
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started