Question
Write a function, def complete_tree(seq: list) -> BinaryTree: Complete_tree() function takes a list of elements as the argument. Create a complete linked binary tree based
Write a function, def complete_tree(seq: list) -> BinaryTree: Complete_tree() function takes a list of elements as the argument. Create a complete linked binary tree based on the given sequence. Test case: seq: [5, 1, 3, 7, 2, 6, 4, 8]. This function should return the tree below.
Python: To answer this question please use method from code below. Thank you very much. Comments on simple words will be appreciated.
class Node:
def __init__(self, element=None, parent=None, left=None, right=None): self.element = element self.parent = parent self.left = left self.right = right
class BinaryTree:
def __init__(self): self.root = None self.size = 0
def size(self): return self.size
def is_empty(self): return self.size == 0
def root(self): return self.root
@staticmethod def parent(n: Node): return n.parent
@staticmethod def children(n: Node): if n.left is not None: yield n.left if n.right is not None: yield n.right
@staticmethod def num_children(n: Node): num = 0 if n.left is not None: num += 1 if n.right is not None: num += 1 return num
@staticmethod def is_leaf(n: Node): return BinaryTree.num_children(n) == 0
def is_root(self, n: Node): return n is self.root def nodes(self): return
@staticmethod def left(n: Node): return n.left
@staticmethod def right(n: Node): return n.right
@staticmethod def sibling(n: Node): p = n.parent if p is None: return None if n is p.left: return p.right if n is p.right: return p.left
def depth(self, n: Node): if n is self.root: return 0 else: return 1 + self.depth(n.parent)
def height(self): return self.__height_helper(self.root)
# recursive method def __height_helper(self, n:Node): if BinaryTree.is_leaf(n): return 0 else: return 1 + max(self.__height_helper(c) for c in BinaryTree.children(n)) def add_root(self, e): # special case if self.root is not None: raise ValueError("Root exists already") self.root = Node(e) self.size += 1
def add_left(self, n:Node, e): if n.left is not None: raise ValueError("n has a left child already") n.left = Node(e) self.size += 1
def add_right(self, n:Node, e): if n.right is not None: raise ValueError("n has a right child already") n.right = Node(e) self.size += 1
def set(self, n:Node, e): old = n.element n.element = e return old
def attach(self, n:Node, t1:BinaryTree, t2:BinaryTree): # check if n is a leaf if not BinaryTree.is_leaf(n): raise ValueError("n must be a leaf") self.size = self.size + t1.size + t2.size if not t1.is_empty(): n.left = t1.root t1.root.parent = n if not t2 is_empty(): n.right = t2.root t2.root.parent = n def remove(self, n:Node): if BinaryTree.num_children(n) == 2: raise ValueError("n has two children!") if n.left is not None: child = n.left else: child = n.right if child is not None: child.parent = n.parent if n is self.root: self.root = child else: parent = n.parent if n is parent.left: parent.left = child else: parent.right = child self.size -= 1 return n.element
## instantiate BinaryTree class tree = BinaryTree() ## create a node with element 10 ## make it the root of the tree node1 = Node(10) tree.root = node1 node2 = Node(20) tree.root.left = node2 node2.parent = node1 node3 = Node(30) tree.root.right = node3 node3.parent = node1 print(BinaryTree.left(node1).element) print(BinaryTree.right(node1).element) print(BinaryTree.sibling(node1)) print(BinaryTree.sibling(node2).element) print(BinaryTree.sibling(node3).element) print(tree.depth(node1))
Step by Step Solution
There are 3 Steps involved in it
Step: 1
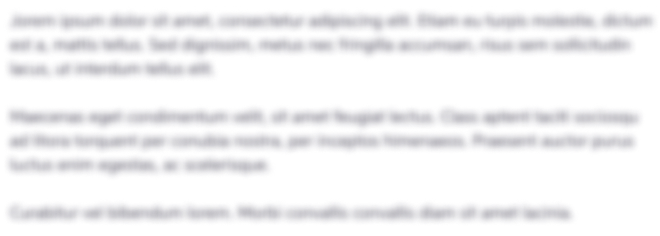
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started