Question
write a function oddSum that calculates and returns the sum of all odd positive integers less than a given number . So, if we call
write a function oddSum that calculates and returns the sum of all odd positive integers less than a given number. So, if we call the function with 6 as its argument, we should get 9 (since 1 + 3+ 5 = 9). This should require the use of a loop.
//////////////////////////////////////////////////////////// // Included headers //////////////////////////////////////// #include
//////////////////////////////////////////////////////////// // Function declaration for Assignment 3 ///////////////////
/** * Function to calculate and return the sum of all odd * positive integers less than the given number. * * @param num - the target number we sum up to @pre > 0 * * @returns sum of all odd positive integers < num */ int oddSum(int num);
//////////////////////////////////////////////////////////// // Main function with some basic tests ///////////////////// int main() { // Test case 1: sum of odd numbers less than 6 // is 1 + 3 + 5 == 9. double res = oddSum(6); if (res == 9) { cout << "Test case 1 OK" << endl; } else { cout << "Test case 1 FAIL: calculated " << res << " instead of 9" << endl; } // Test case 2: sum of odd numbers < 17 is // 1 + 3 + 5+ 7 + 9 + 11 + 13 + 15 = 64 res = oddSum(17) if (res == 64) { cout << "Test case 2 OK" << endl; } else { cout << "Test case 2 FAIL: calculated " << res << " instead of 64" << endl; } // Try some more test cases yourself... // Program done return 0; }
//////////////////////////////////////////////////////////// // Implementations/definitions of your functions ///////////
// Your code to complete the assignment goes here!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
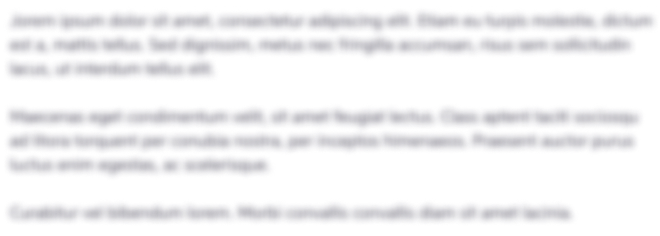
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started