Question
Write a function which implements the Sieve of Eratosthenes. The algorithm follows the steps outlined below: 1. Create a list of integers from 2 to
Write a function which implements the Sieve of Eratosthenes. The algorithm follows the steps outlined below:
1. Create a list of integers from 2 to n (e.g. 2, 3, 4, . . . , n)
2. For integers p in the range [2, n 2 ], do the following: (a) If p is crossed off, skip the following step (b) Otherwise, cross off all multiples of p in the list not including p itself - some of these numbers may already be crossed off and thats okay (e.g. 2p, 3p, 4p, . . .)
3.Each number in the list that wasnt crossed off is prime
For example, if we run this algorithm on n = 10, we get the following steps:
2 3 4 5 6 7 8 9 10 inital list
2 3 /4 5 /6 7 /8 9 ///10 cross off multiples of two
2 3 /4 5 /6 7 /8 /9 ///10 cross off multiples of three (six already crossed, nine now crossed)
2 3 /4 5 /6 7 /8 /9 ///10 skip - four is already crossed out
At the end of this algorithm the numbers 2, 3, 5, and 7 are prime. You should implement this method using an ArrayList of Integers. You can simulate crossing out a number from the list by removing that element from the list.
Be aware that ArrayList implements two different remove methods. remove(int) will remove an element at the index specified by the argument, and remove(Integer) will remove the first element equal to the object specified by the argument. Be careful which one you are calling!
You are also responsible for writing a driver which will match the following sample output.
Enter n: 15
Initial: [2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
Pass #1:[2, 3, 5, 7, 9, 11, 13, 15]
Pass #2:[2, 3, 5, 7, 11, 13]
Pass #3:[2, 3, 5, 7, 11, 13]
Pass #4:[2, 3, 5, 7, 11, 13]
Pass #5:[2, 3, 5, 7, 11, 13]
Pass #6:[2, 3, 5, 7, 11, 13]
Final: [2, 3, 5, 7, 11, 13]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
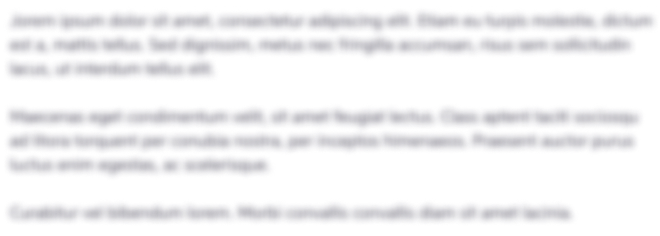
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started