Write a Java Program - Binary and Integer Conversions:
Note: No break or switch statements.
The program will ask the user to enter a choice of conversion they want to make and then either convert a number from base 10 to base 2 or from base 2 into base 10. Your task is to write the methods to validate the numbers that the user enters and to perform the actual conversion of values between the two bases.
Converting from Decimal to Binary The algorithm you will use in this assignment to convert from base 10 to base 2 is:
- If the decimal number is zero, then the binary value is "0"
- Otherwise, start with an empty String to contain your binary value
- While your decimal number is not zero
- Get the remainder of what your current decimal number would be when divided by 2.
- Prepend this result to the front of your String.
- Divide your decimal number by 2 and repeat.
Converting from Binary to Decimal To convert from a binary to a decimal representation, your code will need to take into account the place of each digit in the binary string. The algorithm we will use to do this for this project is:
- Start with a value of 0 for your decimal number
- Assign a value pow to be 1, the value of the power of the rightmost digit in your representation (i.e. 20)
- For each digit in your binary representation, working from right to left on the String
- Multiply the digit by your value for pow
- Add that result to your decimal total
- Multiply pow by 2
- Repeat until all digits in the String have been processed
Your job is to implement the missing methods in the skeleton of code given below. There are four methods you need to write - validInteger, validBinary, intToBinary and binaryToInt. The rest of the code is provided for you. DO NOT CHANGE ANY OF THE CODE PROVIDED.
The two conversion methods - intToBinary and binaryToInt - will implement the algorithms outlined above. Note that in the documentation for these methods there is a specific instruction about what values they are allowed to take as parameters when called - intToBinary requires that its integer parameter be a non-negative integer value while binaryToInt requires that its String parameter be composed of only '1' and '0' character values. The documentation says "the behavior of the method is undefined" if it is passed parameters that don't meet these requirements. This means that as the implementer of these methods, you should only worry about implementing the algorithm assuming correct input has been given - you do not need to worry about the cases where bad inputs are provided to these methods. The two validation methods will be used to ensure that only good inputs are passed to the methods in this program.
NOTE: For the method binaryToInt you are looking at the individual characters of a String. Remember that in order to use the numeric value of the character you MUST convert it to an integer value. Recall from previous projects that there are two methods that we've discussed for doing this - Character.getNumericValue() and Integer.parseInt(). The following two lines of code will convert the digit at index i in the string input into an integer value using getNumericValue():
char c = input.charAt(i);
int val = Character.getNumericValue(c);
And the following two lines of code do the same, using parseInt() instead:
String s = input.substring( i, i + 1 );
int val = Integer.parseInt(s);
IF YOU DO NOT EXPLICITLY CONVERT THE CHARACTER TO AN INTEGER VALUE YOU WILL NOT BE ABLE TO PASS TEST CASES. In addition, code that does not use one of these two methods to convert the character to an integer value will receive an additional deduction in points even if it does pass the test cases.
The two validation methods - validInteger and validBinary - each take a String as input and return true if the String is correctly formatted, false otherwise. Note that you will want to make use of the Character.isDigit(char c) method - this method returns true if the character it is passed as a parameter is a digit 0-9 and false otherwise. The following code segment shows how to use this method to print out a message telling the user whether or not the first character of their input is a digit.
Scanner in = new Scanner(System.in);
String str = in.nextLine();
char c = str.charAt(0);
if (Character.isDigit(c)) {
System.out.println("That's a digit!");
}
else {
System.out.println("That's NOT a digit!");
}
The skeleton for the code you need to implement starts here. Do not forget to update the header with the correct information about this program.
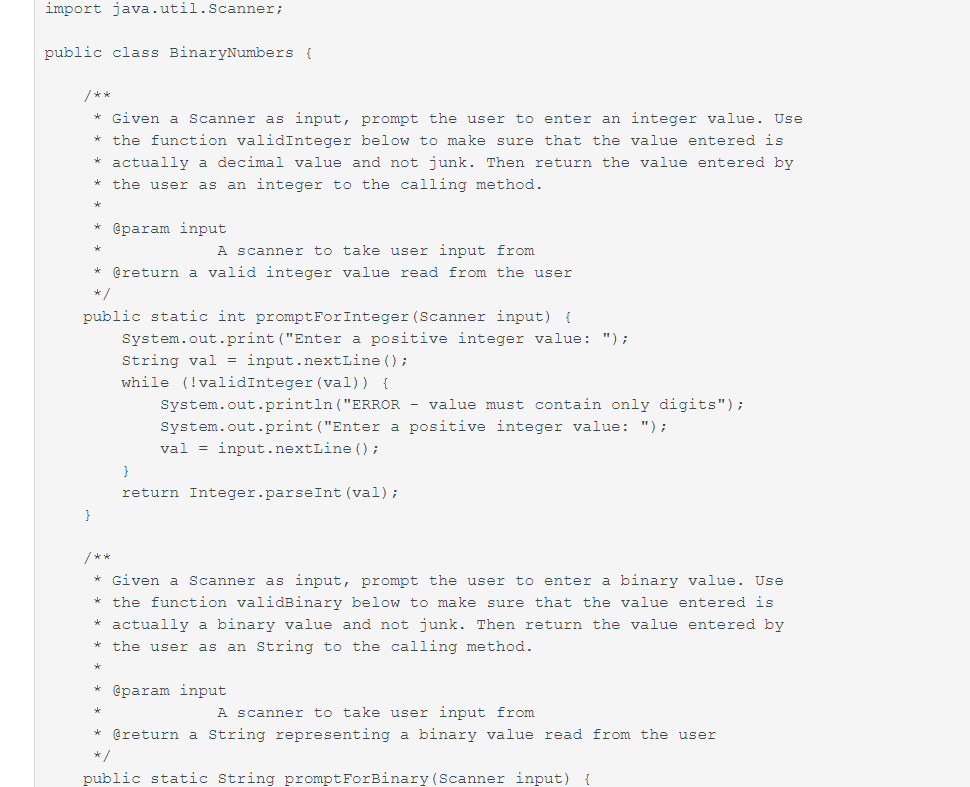
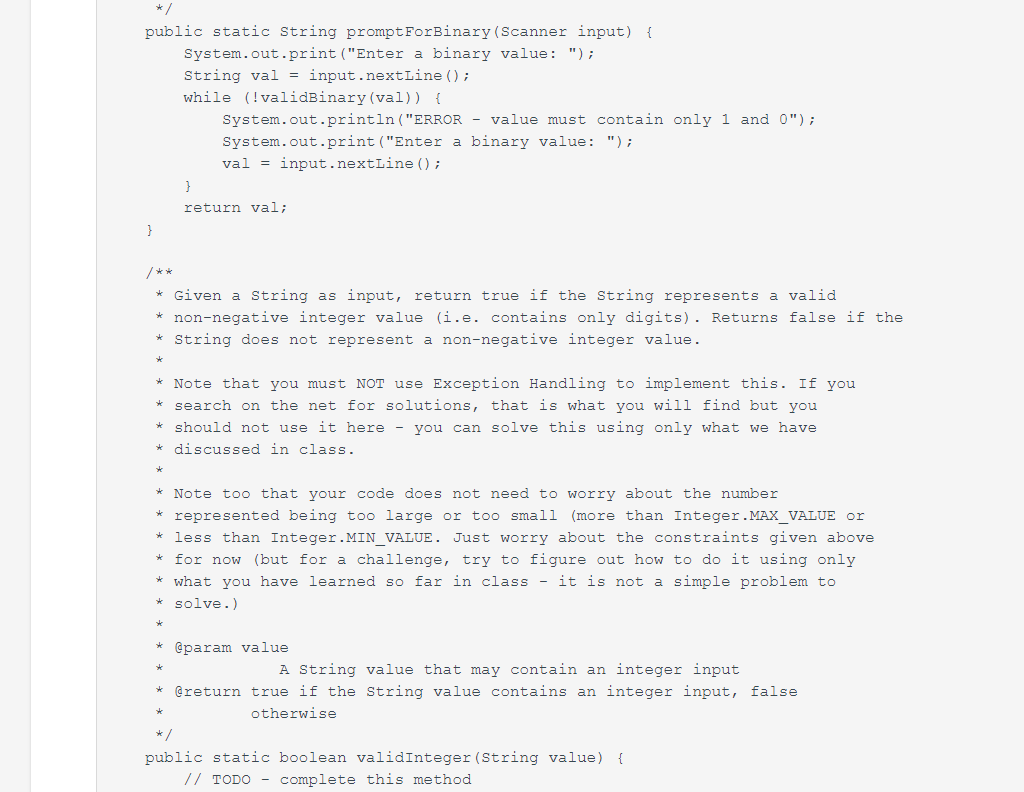
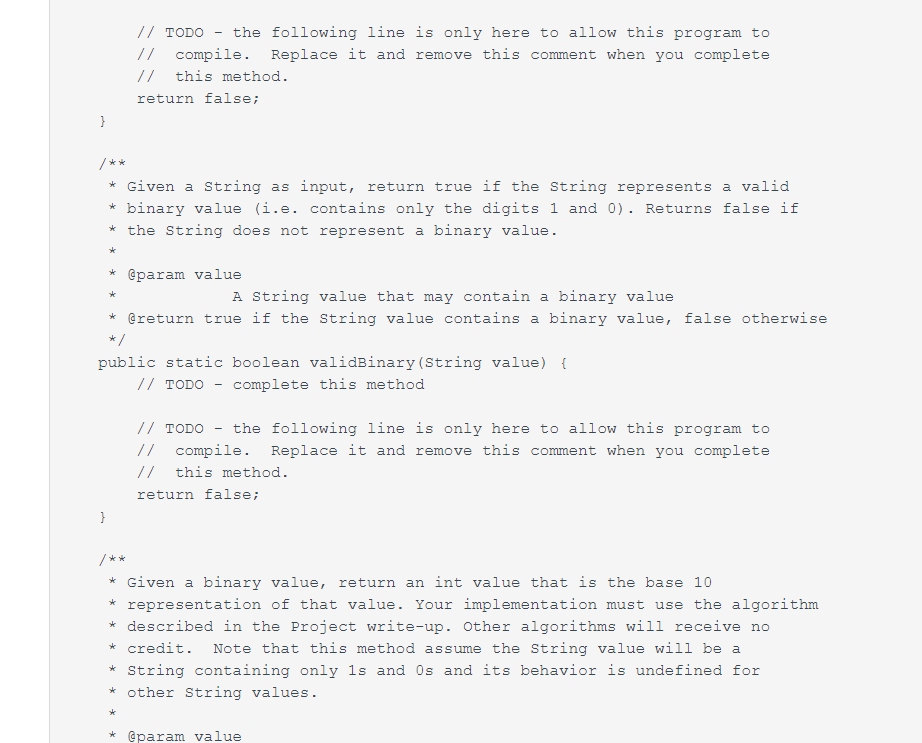
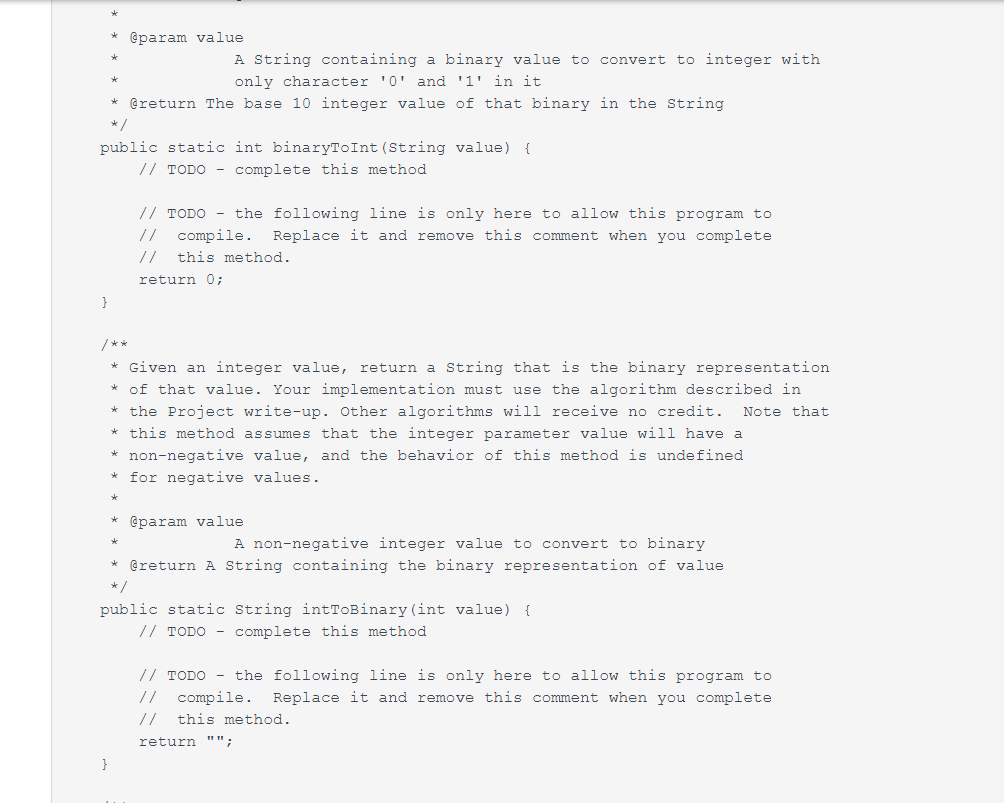
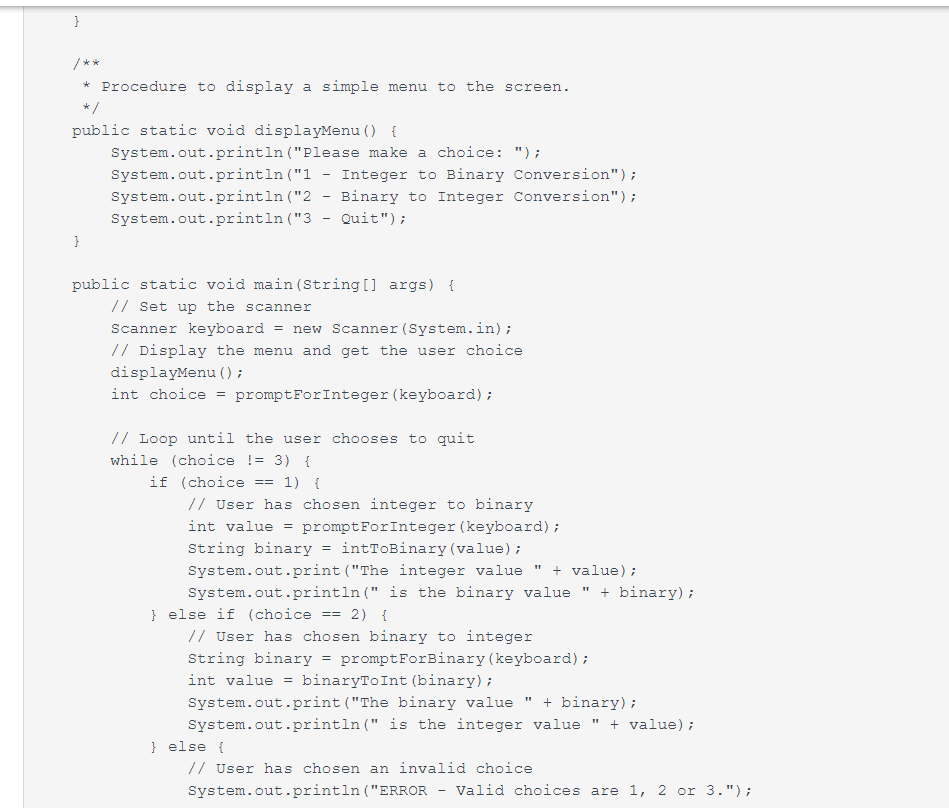
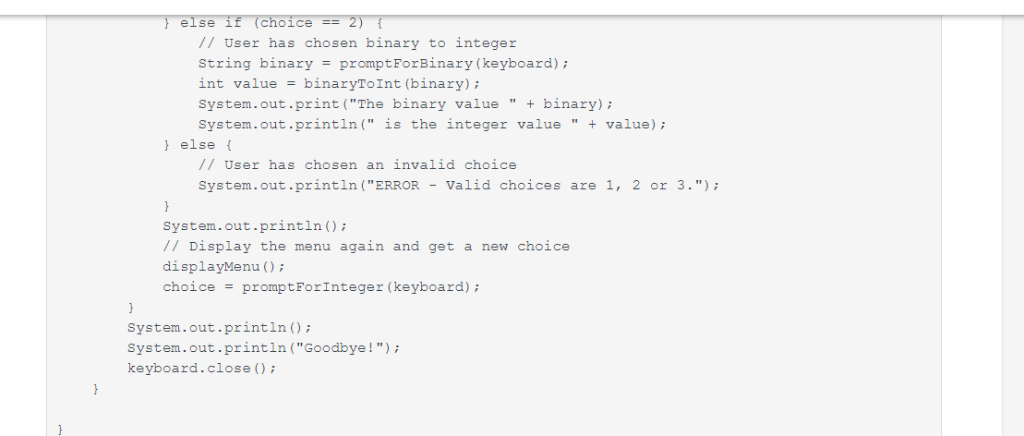
Sample output, user input in BOLD:
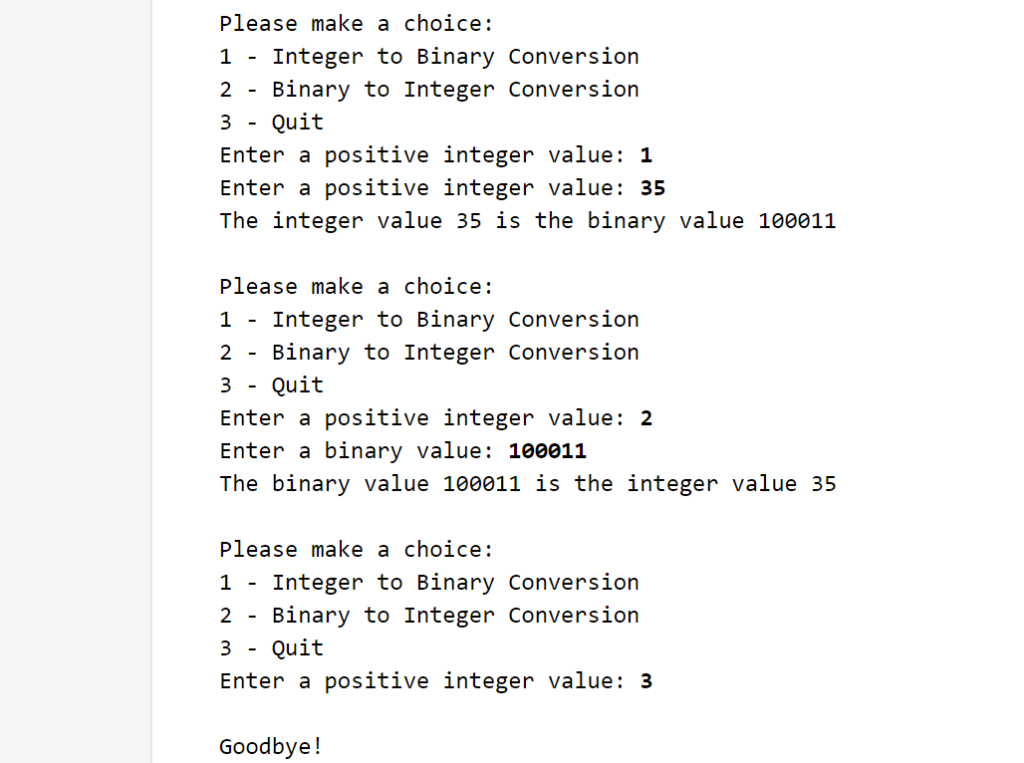
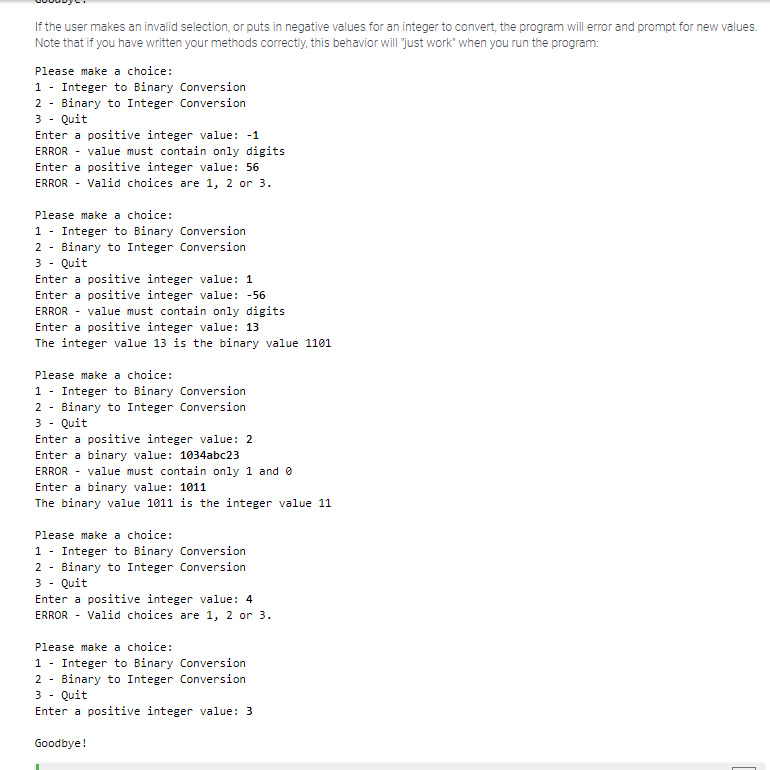
import java.util.Scanneri public class BinaryNumbers Given a Scanner as input, prompt the user to enter an integer value. Use *the function validInteger below to make sure that the value entered is *actually a decimal value and not junk. Then return the value entered by * the user as an integer to the calling method. *@param input A scanner to take user input from *@return a valid integer value read from the user public static int promptForInteger (Scanner input) ( System. out.print ("Enter a positive integer value:") string val-input.nextLine ); while (validInteger (val)) system . ut.printIn("ERROR- value must contain only digits"); System.out.print("Enter a positive integer value: ") valinput.nextLine ) return Integer.parseInt (val); *Given a Scanner as input, prompt the user to enter a binary value. Use *the function validBinary below to make sure that the value entered is *actually a binary value and not junk. Then return the value entered by *the user as an String to the calling method. *@param input A scanner to take user input fronm * @return a String representing a binary value read from the user public static String promptForBinary (Scanner input) public static string promptForBinary (Scanner input) System. out.print ("Enter a binary value: ") String val - input.nextLine) while (validBinary (val)) f System.out.println ("ERROR-value must contain only 1 and 0") System.out.print ("Enter a binary value: ") vai input. nextLine ( ) ; return val; *Given a String as input, return true if the String represents a valid *non-negative integer value (i.e. contains only digits). Returns false if the * String does not represent a non-negative integer value. * Note that you must NOT use Exception Handling to implement this. If you *search on the net for solutions, that is what you will find but you * should not use it here -you can solve this using only what we have * discussed in class *Note too that your code does not need to worry about the number *represented being too large or too small (more than Integer.MAX_VALUE or *less than Integer.MIN VALUE. Just worry about the constraints given above *for now (but for a challenge, try to figure out how to do it using only *what you have learned so far in class- it is not a simple problem to * solve.) @param value * @return true if the String value contains an integer input, false A String value that may contain an integer input otherwise public static boolean validInteger (String value) f // TOD -complete this meth d // TODO - the following line is only here to allow this program to // compile. Replace it and remove this comment when you complete /I this method. return false; *Given a String as input, return true if the String represents a valid binary value (i.e. contains only the digits 1 and 0). Returns false if the String does not represent a binary value. *@param value A String value that may contain a binary value @return true if the String value contains a binary value, false otherwise public static boolean validBinary (String value) [ // TODO -complete this method TODO-the following line is only here to allow this program to // compile. Replace it and remove this comment when you complete /I this method. return false; *Given a binary value, return an int value that is the base 10 *representation of that value. Your implementation must use the algorithm * described in the Project write-up. Other algorithms will receive no * credit. Note that this method assume the String value will be a string containing only 1s and 0s and its behavior is undefined for other string values * @param value *@param value A String containing a binary value to convert to integer with only character '0' and '1' in it * @return The base 10 integer value of that binary in the String public static int binaryToInt (String value) / TODO -complete this method // TODO - the following line is only here to allow this program to / compile. Replace it and remove this comment when you complete // this method. return 0 *Given an integer value, return a String that is the binary representation *of that value. Your implementation must use the algorithm described in *the Project write-up. Other algorithms will receive no credit. Note that *this method assumes that the integer parameter value will havea * non-negative value , and the behavi r f this method is undefined * for negative values. *@param value A non-negative integer value to convert to binary *@return A String containing the binary representation of value public static string intToBinary (int value) // TODO - complete this method /7 TODO - the following line is only here to allow this program to / compile. Replace it and remove this comment when you complete // this method. return""; * Procedure to display a simple menu to the screen. public static void displayMenu) t System. out.println("Please make a choice: ") System.out.println("1 - Integer to Binary Conversion") System.out.println("2 - Binary to Integer Conversion"); system.out.printIn(" Quit'' ); public static void main (String[] args) // Set up the scanner Scanner keyboard- new Scanner (System.in); /7 Display the menu and get the user choice displayMenu ) int ch ice = promp tForinteger (keyboard) ; // Loop until the user chooses to quit while (choice3) t if (choice 1) ( /7 User has chosen integer to binary int value promptForInteger (keyboard); String binary- intToBinary (value) system . out.print ("The integer value " value); System.out.println(" is the binary value "+ binary); else if (choice 2) [ /7 User has chosen binary to integer string binarypromptForBinary (keyboard); int value = binaryTo Int (binary); System.out.print ("The binary value"binary) System.out.println(" is the integer value " value) else // User has chosen an invalid choice System.out.println ("ERROR-Valid choices are 1, 2 or 3.") else // User has chosen binary to integer string binary prptForBinary(keyboard); int value-binaryToInt (binary) system.out.print("The binary value"binary) System.out.println(" is the integer value " value) else // User has chosen an invalid choice System.out.println ("ERROR -Valid choices are 1, 2 or 3.") System.out.println) // Display the menu again and get a new choice displayMenu ) choice promptForinteger (keyboard) System.out.printlnO System.out.printin ("Goodbye!") keyboard.close ) Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 1 Enter a positive integer value: 35 The integer value 35 is the binary value 100011 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 2 Enter a binary value: 1e0011 The binary value 100011 is the integer value 35 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 - Quit Enter a positive integer value: 3 Goodbye! If the user makes an invalid selection, or puts in negative values for an integer to convert, the program will error and prompt for new values. Note that if you have written your methods correctly, this behavior will just work" when you run the program Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 1 ERROR value must contain only digits Enter a positive integer value: 56 ERROR Valid choices are 1, 2 or 3 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 1 Enter a positive integer value: -56 ERROR value must contain only digits Enter a positive integer value: 13 The integer value 13 is the binary value 1101 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 2 Enter a binary value: 1034abc23 ERROR value must contain only 1 ande Enter a binary value: 1011 The binary value 1011 is the integer value 11 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 4 ERROR Valid choices are 1, 2 or 3 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 3 Goodbye! import java.util.Scanneri public class BinaryNumbers Given a Scanner as input, prompt the user to enter an integer value. Use *the function validInteger below to make sure that the value entered is *actually a decimal value and not junk. Then return the value entered by * the user as an integer to the calling method. *@param input A scanner to take user input from *@return a valid integer value read from the user public static int promptForInteger (Scanner input) ( System. out.print ("Enter a positive integer value:") string val-input.nextLine ); while (validInteger (val)) system . ut.printIn("ERROR- value must contain only digits"); System.out.print("Enter a positive integer value: ") valinput.nextLine ) return Integer.parseInt (val); *Given a Scanner as input, prompt the user to enter a binary value. Use *the function validBinary below to make sure that the value entered is *actually a binary value and not junk. Then return the value entered by *the user as an String to the calling method. *@param input A scanner to take user input fronm * @return a String representing a binary value read from the user public static String promptForBinary (Scanner input) public static string promptForBinary (Scanner input) System. out.print ("Enter a binary value: ") String val - input.nextLine) while (validBinary (val)) f System.out.println ("ERROR-value must contain only 1 and 0") System.out.print ("Enter a binary value: ") vai input. nextLine ( ) ; return val; *Given a String as input, return true if the String represents a valid *non-negative integer value (i.e. contains only digits). Returns false if the * String does not represent a non-negative integer value. * Note that you must NOT use Exception Handling to implement this. If you *search on the net for solutions, that is what you will find but you * should not use it here -you can solve this using only what we have * discussed in class *Note too that your code does not need to worry about the number *represented being too large or too small (more than Integer.MAX_VALUE or *less than Integer.MIN VALUE. Just worry about the constraints given above *for now (but for a challenge, try to figure out how to do it using only *what you have learned so far in class- it is not a simple problem to * solve.) @param value * @return true if the String value contains an integer input, false A String value that may contain an integer input otherwise public static boolean validInteger (String value) f // TOD -complete this meth d // TODO - the following line is only here to allow this program to // compile. Replace it and remove this comment when you complete /I this method. return false; *Given a String as input, return true if the String represents a valid binary value (i.e. contains only the digits 1 and 0). Returns false if the String does not represent a binary value. *@param value A String value that may contain a binary value @return true if the String value contains a binary value, false otherwise public static boolean validBinary (String value) [ // TODO -complete this method TODO-the following line is only here to allow this program to // compile. Replace it and remove this comment when you complete /I this method. return false; *Given a binary value, return an int value that is the base 10 *representation of that value. Your implementation must use the algorithm * described in the Project write-up. Other algorithms will receive no * credit. Note that this method assume the String value will be a string containing only 1s and 0s and its behavior is undefined for other string values * @param value *@param value A String containing a binary value to convert to integer with only character '0' and '1' in it * @return The base 10 integer value of that binary in the String public static int binaryToInt (String value) / TODO -complete this method // TODO - the following line is only here to allow this program to / compile. Replace it and remove this comment when you complete // this method. return 0 *Given an integer value, return a String that is the binary representation *of that value. Your implementation must use the algorithm described in *the Project write-up. Other algorithms will receive no credit. Note that *this method assumes that the integer parameter value will havea * non-negative value , and the behavi r f this method is undefined * for negative values. *@param value A non-negative integer value to convert to binary *@return A String containing the binary representation of value public static string intToBinary (int value) // TODO - complete this method /7 TODO - the following line is only here to allow this program to / compile. Replace it and remove this comment when you complete // this method. return""; * Procedure to display a simple menu to the screen. public static void displayMenu) t System. out.println("Please make a choice: ") System.out.println("1 - Integer to Binary Conversion") System.out.println("2 - Binary to Integer Conversion"); system.out.printIn(" Quit'' ); public static void main (String[] args) // Set up the scanner Scanner keyboard- new Scanner (System.in); /7 Display the menu and get the user choice displayMenu ) int ch ice = promp tForinteger (keyboard) ; // Loop until the user chooses to quit while (choice3) t if (choice 1) ( /7 User has chosen integer to binary int value promptForInteger (keyboard); String binary- intToBinary (value) system . out.print ("The integer value " value); System.out.println(" is the binary value "+ binary); else if (choice 2) [ /7 User has chosen binary to integer string binarypromptForBinary (keyboard); int value = binaryTo Int (binary); System.out.print ("The binary value"binary) System.out.println(" is the integer value " value) else // User has chosen an invalid choice System.out.println ("ERROR-Valid choices are 1, 2 or 3.") else // User has chosen binary to integer string binary prptForBinary(keyboard); int value-binaryToInt (binary) system.out.print("The binary value"binary) System.out.println(" is the integer value " value) else // User has chosen an invalid choice System.out.println ("ERROR -Valid choices are 1, 2 or 3.") System.out.println) // Display the menu again and get a new choice displayMenu ) choice promptForinteger (keyboard) System.out.printlnO System.out.printin ("Goodbye!") keyboard.close ) Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 1 Enter a positive integer value: 35 The integer value 35 is the binary value 100011 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 2 Enter a binary value: 1e0011 The binary value 100011 is the integer value 35 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 - Quit Enter a positive integer value: 3 Goodbye! If the user makes an invalid selection, or puts in negative values for an integer to convert, the program will error and prompt for new values. Note that if you have written your methods correctly, this behavior will just work" when you run the program Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 1 ERROR value must contain only digits Enter a positive integer value: 56 ERROR Valid choices are 1, 2 or 3 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 1 Enter a positive integer value: -56 ERROR value must contain only digits Enter a positive integer value: 13 The integer value 13 is the binary value 1101 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 2 Enter a binary value: 1034abc23 ERROR value must contain only 1 ande Enter a binary value: 1011 The binary value 1011 is the integer value 11 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 4 ERROR Valid choices are 1, 2 or 3 Please make a choice: 1 Integer to Binary Conversion 2 Binary to Integer Conversion 3 Quit Enter a positive integer value: 3 Goodbye