Question
Write a Java Program that does the following... Display a message to enter a principal amount between 1 and 500000. If an amount outside this
Write a Java Program that does the following... Display a message to enter a principal amount between 1 and 500000. If an amount outside this range is entered, the message should again be displayed until a valid amount is entered. -Display a message to enter a term between 1 and 30. If an term outside this range is entered, the message should again be displayed until a valid term is entered. -Display a message to enter an interest rate between 1 and 15 percent. If an interest rate outside this range is entered, the message should again be displayed until a valid term is entered. -A MortgageCalcs object should be created with the values obtained above -Your client should then call the appopriate methods in MortgageCalcs and display the resulting payment, future value, and interest payment. Your client output should look like this: Principal: 100000.00 Future Value: 134736.12 Term of loan: 10.00 Interest Rate: 6.25 Interest Charge: 34736.12 Payment: 1122.80 -Your client should then display a message to enter a number of months. This parameter should then be passed to the MortgageCalcs amortize method. If the amortize method returns false, then you should display the message "Number of Months too big". Otherwise, amortize will display the amortization table. You should continue to display a message to enter a number of months and call the amortize method until -1 is entered. At this point your program should terminate. Notes: -Input and output should be done using a Scanner object and System.out.print/ln -Your client should follow the steps in the order outlined above exactly. -All varaibles used in calculations should be of type double -You should name your client TestMyMortgage.java -Remember to include comments that contain your name and student ID as well as documenting varaibles and general description of intent of code
MortgageCalc.clas Below
package mortgage;
import java.text.DecimalFormat;
public class MortgageCalcs {
// private instance variables
private double interestRate;
private double term;
private double principal;
DecimalFormat df = new DecimalFormat("0.00");
// Constructor Method. Accepts values for interest rate, term, and principal
// and places
// these values into the corresponding instance variable
public MortgageCalcs(double interestRate, double term, double principal) {
this.interestRate = interestRate;
this.term = term;
this.principal = principal;
}
// this method calculates and returns the Monthly Payment.
public double calcPayment() {
double monthlyPayment = (principal * intCharge() * Math.pow(1 + intCharge(), term))
/ (Math.pow(1 + intCharge(), term) - 1);
return monthlyPayment;
}
// this method calculates and returns the Future Value.
public double futureValue() {
double monthlyInterest = principal * intCharge();
double debtPaid = calcPayment() - monthlyInterest;
principal = principal - debtPaid;
return principal;
}
// this method calculates and returns the Interest Charge.
public double intCharge() {
return interestRate / (12 * 100);
}
// get methods for each instance variable
public double getInterestRate() {
return interestRate;
}
public double getTerm() {
return term;
}
public double getPrincipal() {
return principal;
}
// this method produces an amortization table for the loan.
public boolean amortize(int months) {
double monthlyPayment = calcPayment();
double interestCharge = intCharge();
int i = 1;
//Checks for the number of months entered and principal remaining is not less than monthly emi
while (principal >= monthlyPayment && i <= months) {
double monthlyInterest = principal * interestCharge;
double debtPaid = monthlyPayment - monthlyInterest;
principal = principal - debtPaid;
System.out.println(i + " \t $" + df.format(monthlyPayment) + " \t $" + df.format(monthlyInterest) + " \t $"
+ df.format(debtPaid) + " \t $" + df.format(principal));
i++;
}
//If principal amount is less than monthly payment
if (i <= months)
System.out.println(i + " \t $" + df.format(principal) + " \t $" + df.format(principal) + " \t $"
+ df.format(principal) + " \t $0.00");
return true;
}
}
MainTest.java
package mortgage;
public class MainTest {
//Main method to create Mortgage class and use amortize method.
public static void main(String[] args) {
double principal = 20000;
double interestRate = 7.5;
int term = 60;
MortgageCalcs mortgage = new MortgageCalcs(interestRate, term, principal);
System.out.println("Month# \t Amount \t interest \t Principal \t Balance");
System.out.println("====== \t ============= \t ============= \t ========= \t ============");
mortgage.amortize(10);
}
}
Sample Output
Month# Amount interest Principal Balance
====== ============= ============= ========= ============
1 $400.76 $125.00 $275.76 $19724.24
2 $400.76 $123.28 $277.48 $19446.76
3 $400.76 $121.54 $279.22 $19167.54
4 $400.76 $119.80 $280.96 $18886.58
5 $400.76 $118.04 $282.72 $18603.86
6 $400.76 $116.27 $284.48 $18319.38
7 $400.76 $114.50 $286.26 $18033.11
8 $400.76 $112.71 $288.05 $17745.06
9 $400.76 $110.91 $289.85 $17455.21
10 $400.76 $109.10 $291.66 $17163.55
Step by Step Solution
There are 3 Steps involved in it
Step: 1
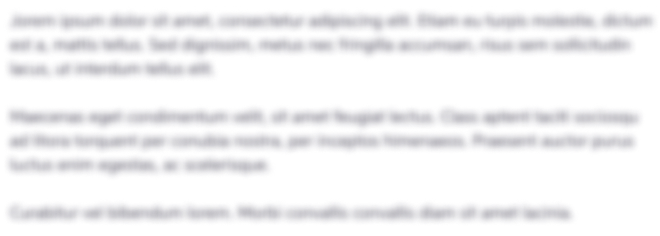
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started