Question
Write a Java program to test for palindrome integers and strings. A number/string is a palindrome if its reversal is the same as itself. In
Write a Java program to test for palindrome integers and strings.
A number/string is a palindrome if its reversal is the same as itself.
In the object class Palindrome, write the following methods:
- public int reverse(int number) - returns the reversal of an integer, i.e., reverse(456) will return 654
- public boolean isPalindrome(int number) - return true if the number received as parameter is a palindrome. Use the reverse method to implement isPalindrome.
Write a testPalindrome class that prompts the user to enter an integer and reports whether the integer is a palindrome.
Example of output for input 1221:
The number 1221 is a palindrome
Example output for input 1234:
The number 1234 is not a palindrome
Example output for input 91419:
The number 91419 is not a palindrome
Expand the Palindrome object class by overloading the reverse and isPalindrome methods. Do this by adding another reverse method that will reverse and return the reversed string. Also add another isPalindrome that will receive a string instead of an integer to test for being a palindrome.
In the test class, ask the user to enter a string and display the result.
Example of output for the input dad:
The word dad is a palindrome
Example of output for the input add:
The word add is not a palindrome
Example of output for the input racecar:
The word racecar is a palindrome
Hint! Prompt the user first if they want to enter a word or a number, then continue based on their response.
This is the code
Reverse code for integer int result = 0; while (number != 0) { int remainder = number % 10; result = result * 10 + remainder; number = number / 10; } Reverse code for string String result = ""; for (int i = 0; i < str.length(); i++) { result = str.charAt(i) + result; } IsPalindrome code for integer boolean result = true; if (number != reverse(number)) result = false; IsPalindrome code for string boolean result = true; if (!(str.equals(reverse(str)))) result = false;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
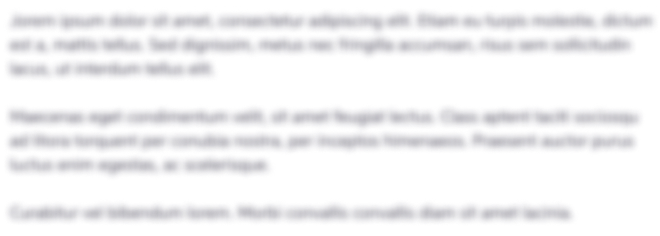
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started