Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a Java program which will input sequences of characters representing infix expressions involving the binary (dyadic) operators +, -, *, and plus parentheses
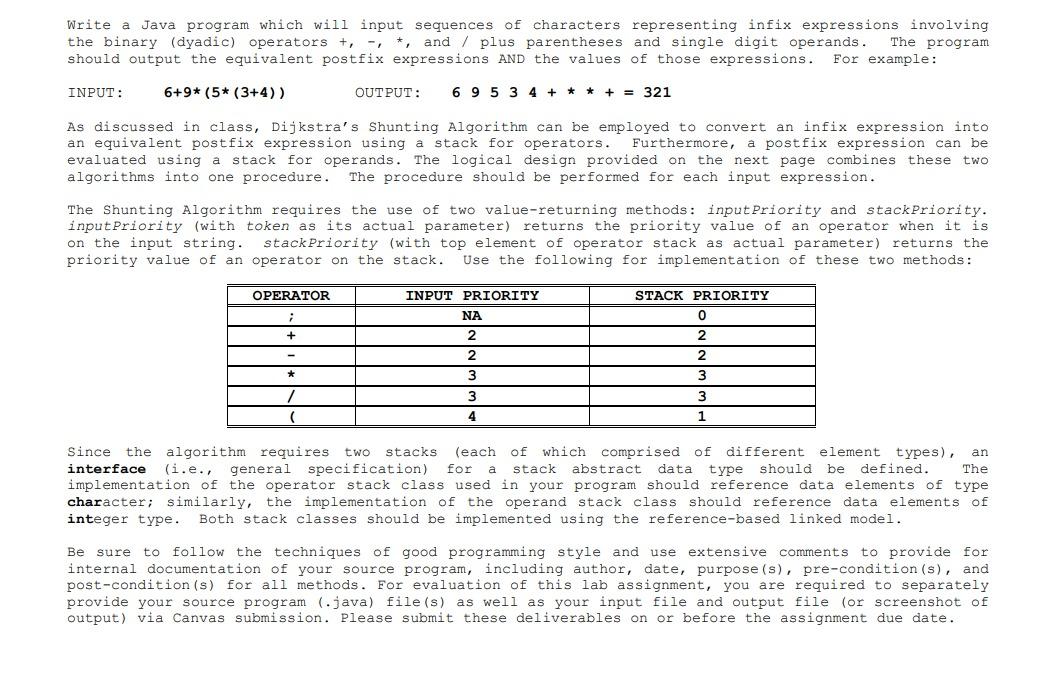
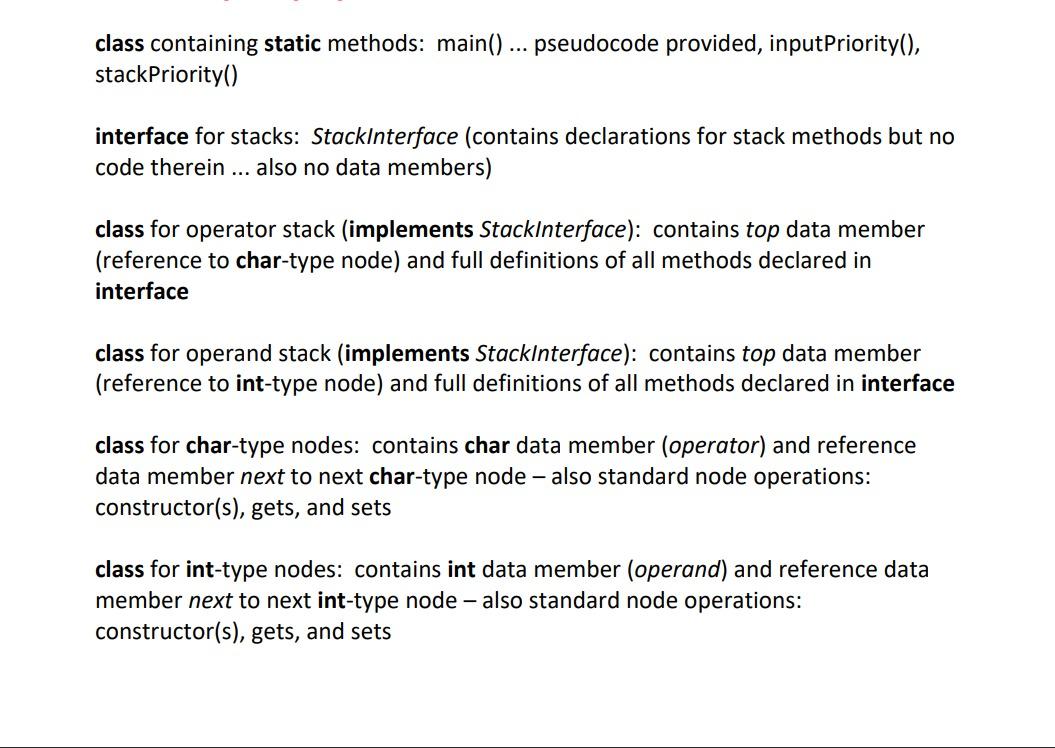
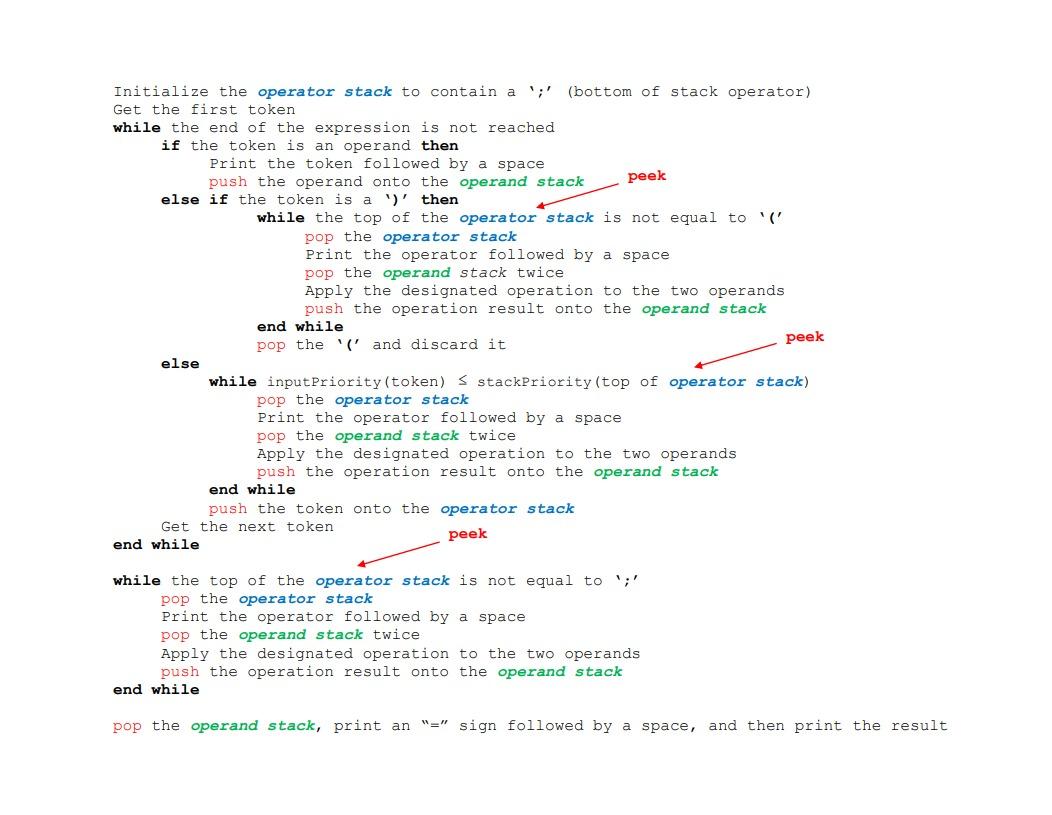
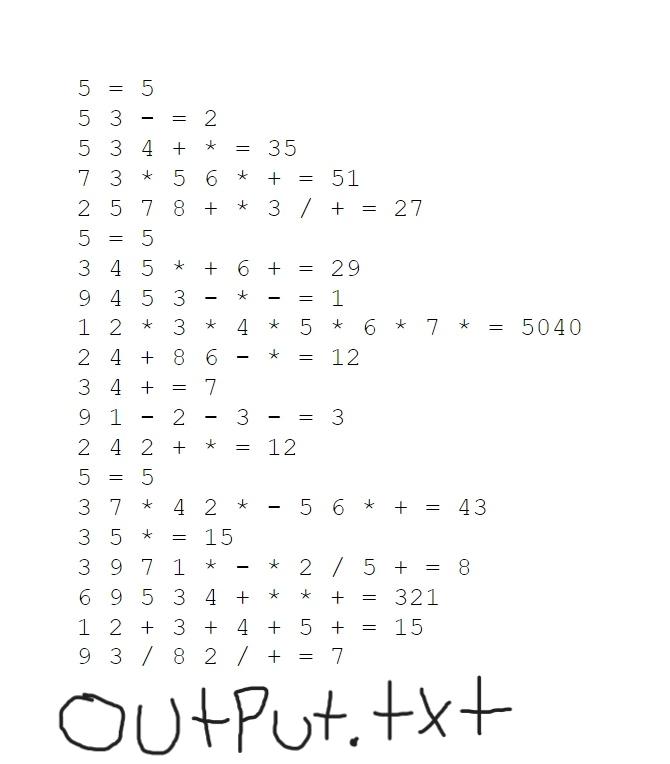
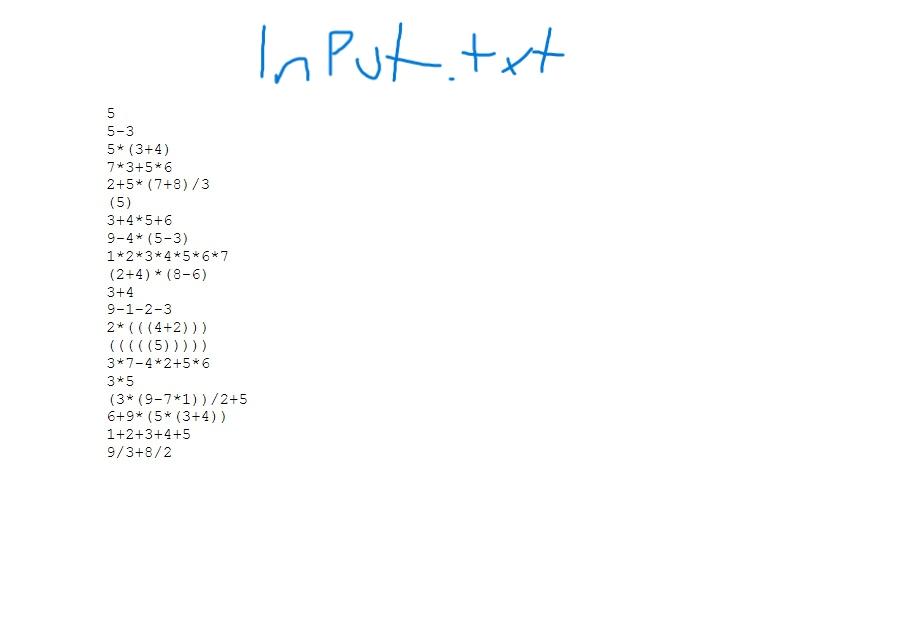
Write a Java program which will input sequences of characters representing infix expressions involving the binary (dyadic) operators +, -, *, and plus parentheses and single digit operands. The program should output the equivalent postfix expressions AND the values of those expressions. For example: INPUT: 6+9* (5* (3+4)) OUTPUT: 6 9 5 3 4 + += 321 As discussed in class, Dijkstra's Shunting Algorithm can be employed to convert an infix expression into an equivalent postfix expression using a stack for operators. Furthermore, a postfix expression can be evaluated using a stack for operands. The logical design provided on the next page combines these two algorithms into one procedure. The procedure should be performed for each input expression. The Shunting Algorithm requires the use of two value-returning methods: input Priority and stackPriority. input Priority (with token as its actual parameter) returns the priority value of an operator when it is on the input string. stack Priority (with top element of operator stack as actual parameter) returns the priority value of an operator on the stack. Use the following for implementation of these two methods: OPERATOR INPUT PRIORITY + * NA 2 2 3 3 4 STACK PRIORITY 0 2 2 3 3 1 ( Since the algorithm requires two stacks (each of which comprised of different element types), an interface (i.e., general specification) for a stack abstract data type should be defined. The implementation of the operator stack class used in your program should reference data elements of type character; similarly, the implementation of the operand stack class should reference data elements of integer type. Both stack classes should be implemented using the reference-based linked model. Be sure to follow the techniques of good programming style and use extensive comments to provide for internal documentation of your source program, including author, date, purpose (s), pre-condition (s), and post-condition (s) for all methods. For evaluation of this lab assignment, you are required to separately provide your source program (.java) file(s) as well as your input file and output file (or screenshot of output) via Canvas submission. Please submit these deliverables on or before the assignment due date. class containing static methods: main() ... pseudocode provided, inputPriority(), stackPriority() interface for stacks: StackInterface (contains declarations for stack methods but no code therein... also no data members) class for operator stack (implements StackInterface): contains top data member (reference to char-type node) and full definitions of all methods declared in interface class for operand stack (implements StackInterface): contains top data member (reference to int-type node) and full definitions of all methods declared in interface class for char-type nodes: contains char data member (operator) and reference data member next to next char-type node - also standard node operations: constructor(s), gets, and sets class for int-type nodes: contains int data member (operand) and reference data member next to next int-type node - also standard node operations: constructor(s), gets, and sets Initialize the operator stack to contain a ';' (bottom of stack operator) Get the first token while the end of the expression is not reached if the token is an operand then Print the token followed by a space push the operand onto the operand stack else if the token is a ')' then else peek while the top of the operator stack is not equal to '(' pop the operator stack Print the operator followed by a space pop the operand stack twice Apply the designated operation to the two operands push the operation result onto the operand stack end while pop the '(' and discard it peek while inputPriority (token) stackPriority (top of operator stack) pop the operator stack Print the operator followed by a space pop the operand stack twice Apply the designated operation to the two operands push the operation result onto the operand stack end while push the token onto the operator stack Get the next token end while peek while the top of the operator stack is not equal to ';' pop the operator stack Print the operator followed by a space pop the operand stack twice Apply the designated operation to the two operands push the operation result onto the operand stack end while pop the operand stack, print an "=" sign followed by a space, and then print the result 5 = 5 3 5 - 5 3 4 = 2 + 73 * 56 = 35 * * +3 = 51 3 / + +6+ = 29 = 1 2 5 7 8 + 5 = . 5 3 4 5 * 9453 - * 1 2 3 * 4 * = 27 567 * : = 5040 = 12 2 48 34 + = 9 1 | - ||2 + 25 242 + 5 3 = 7 3 5 * * 19 6 - - * 3 = 42* = 3 9 7 1 15 * - 6 9 5 3 4 + * - 12 = 3 - 56 = 43 * 2 / 5 + = 8 + 12+ 3+ 4+ 5+ = 321 = 15 9 3/8 2 / + = 7 Output, +x+ 5 5-3 5* (3+4) 7*3+5*6 2+5* (7+8)/3 (5) 3+4*5+6 9-4 (5-3) 1*2*3*4*5*6*7 (2+4)* (8-6) 3+4 9-1-2-3 2*(((4+2))) (((((5))))) 3*7-4*2+5*6 3*5 (3* (9-7*1))/2+5 6+9 (5* (3+4)) 1+2+3+4+5 9/3+8/2 In Put.txt
Step by Step Solution
There are 3 Steps involved in it
Step: 1
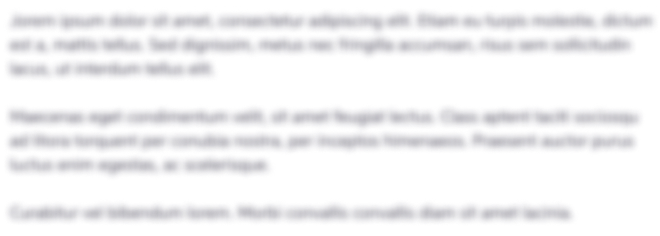
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started