Question
Write a Java program: Write a Java application that reads three integers from the user and displays the sum, product, average, smallest, largest, and median
Write a Java program:
Write a Java application that reads three integers from the user and displays the sum, product, average, smallest, largest, and median of the numbers. Use the following sample code as a guide.
import java.util.Scanner;
public class IntegerOps {
public static void main(String[] args)
{
int num1, num2, num3;
int sum,;
// Use Scanner class to read the three integer
sum = sum(num1, num2, num3); //The sum method calculates the sum
//Call product method to calculate the product
//Call product method to calculate the average (real number)
//Call product method to calculate the smallest number
//Call product method to calculate the largest number
//Call product method to calculate the median of the 3 numbers
System.out.println("Sum = " + sum);
// Print the product, average, smallest, largest, and median in separate lines
}
/**
* This method calculates the sum of 3 integers
* @param x the first integer
* @param y the second integer
* @param z the third integer
* @return the sum of the 3 integers
*/
public static int sum(int x, int y, int z)
{
return x + y + z;
}
}
Below is an example of how to calculate the median of 3 integers.
public class Test {
public static void main(String[] args) {
int x= 6;
int y = 4;
int z = 8;
int larger1, smaller1, larger2, smaller2, median;
smaller1 = x; //assuming the smaller of x & y is x
larger1 = y; //assuming the larger of x & y is y
smaller2 = x; //assuming the smaller of x & z is x
larger2 = z; //assuming the larger of x and z is z
if(y < x) //fix the above assumptions of the x & y if needed
{
smaller1 = y;
larger1 = x;
}
if(z < x) //fix the above assumptions of the x & z if needed
{
smaller2 = z;
larger2 = x;
}
median = smaller2; //assume the median is smaller2. We will fix it later if needed
if (smaller1 == smaller2) //if the smaller of the two pairs is the same, then the median is one of the following
{
if(larger1 < larger2) //the median cannot be the largest number, so it is the smaller of the two largest
median = larger1;
else
median = larger2;
}
else if (smaller1 > smaller2) //if the smaller of the two pairs are not equal, then the larger of the two is the median
median = smaller1;
//could have added an else here, but is really the above assumption of median=smaller2 so it is omitted.
System.out.printf("median=%d", median);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
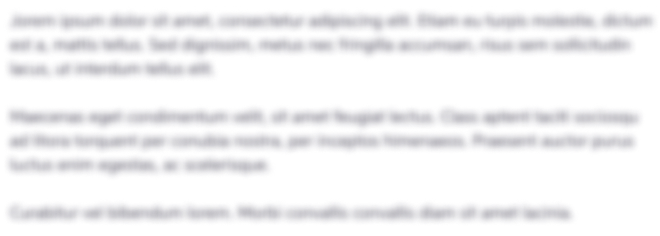
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started