Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a main function to test your heap sort implementation: - Initialize an array of integers, e . g . , int arr [ ]
Write a main function to test your heap sort implementation:
Initialize an array of integers, eg int arr;
Print the original array.
Sort the array using the heap sort function.
Print the sorted array.
Sample Output:
Original array:
Sorted array:
In C Language Please!!
Heap Sort Function:
#include
Function to swap two elements
void swapint a int b
int temp a;
a b;
b temp;
Function to heapify a subtree rooted with node i
void heapifyint arr int n int i
int largest i; Initialize largest as root
int left i ; left child index
int right i ; right child index
If left child is larger than root
if left n && arrleft arrlargest
largest left;
If right child is larger than largest so far
if right n && arrright arrlargest
largest right;
If largest is not root
if largest i
swap&arri &arrlargest;
Recursively heapify the affected subtree
heapifyarr n largest;
Function to perform heap sort
void heapSortint arr int n
Build heap rearrange array
for int i n ; i ; i
heapifyarr n i;
One by one extract an element from heap
for int i n ; i ; i
Move current root to end
swap&arr &arri;
Call heapify on the reduced heap
heapifyarr i;
Function to print an array
void printArrayint arr int n
for int i ; i n; i
printfd arri;
printf
;
int main
int arr;
int n sizeofarr sizeofarr;
printfOriginal array: ;
printArrayarr n;
heapSortarr n;
printfSorted array: ;
printArrayarr n;
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
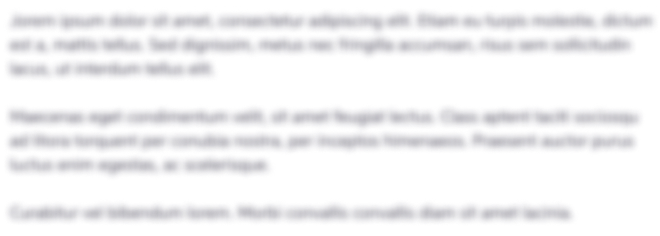
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started