Write a main.cpp file containing:
A class definition for a Power class.
A class definition for a Fighter class.
Implementations for the member functions of the Power class.
Implementations for the member functions of the Fighter class.
Implementation for a main function used to test the Fighter class and the Power class
Include the following libraries: vector, iostream, string and use the standard namespace.
Must follow specification. Avoid changing the class specification. Avoid modifying the main function provided. Avoid excessively unnecessary steps in algorithms, writing excessively more code than needed, using excessive memory. Use appropriate variable names and whitespace for readability. Write useful comments documenting what is being tested on each test in the main function provided. There are 17 error cases-each one should have a comment. Write useful comments on blocks of code. Write useful comments to describe variables and named constants (be sure to add documentation to main; several variables are created with no comments describing them.) Write a purpose/precondition/postconditon for each method you define (you can draw some of this from the specification, but you will need to add anything that is missing.)
Do not use "go to" statements in this argument
Do not use "break" to get out of a loop. Control loops with the loop condition statements/flags instead.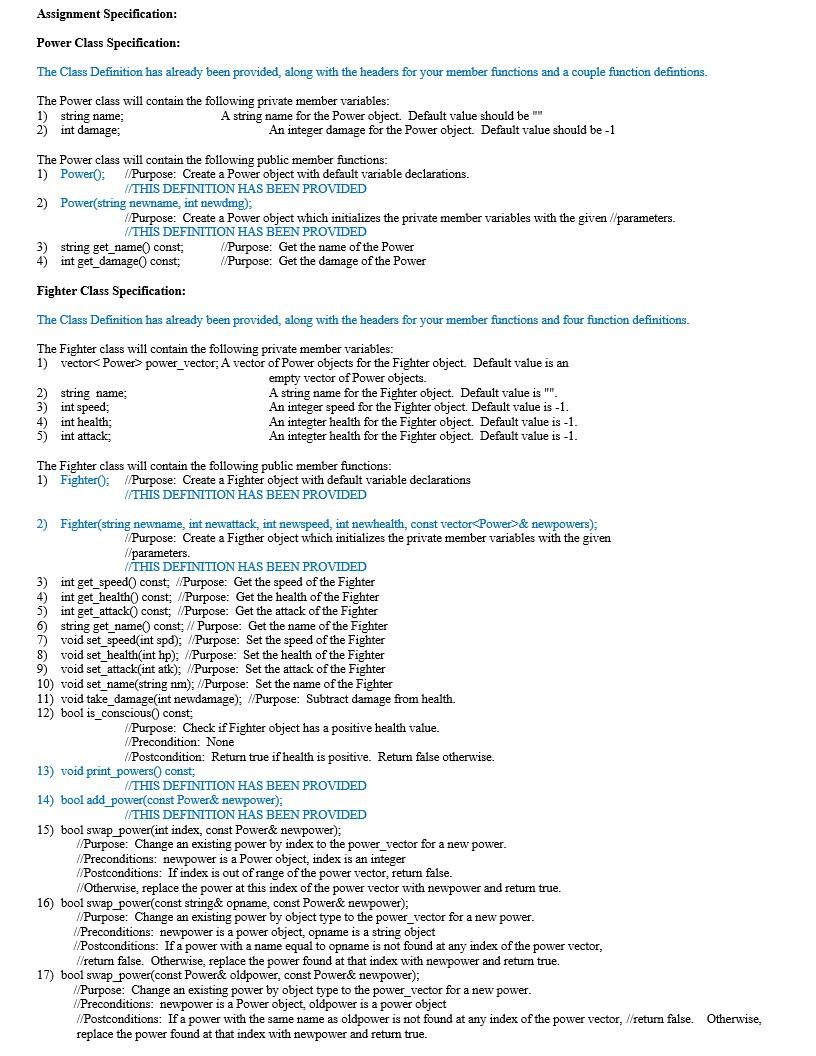
Assignment Specification: Power Class Specification: The Class Definition has already been provided, along with the headers for your member functions and a couple function defintions. The Power class will contain the following private member variables: 1) string name: A string name for the Power object. Default value should be 2) int damage An integer damage for the Power object. Default value should be - 1 The Power class will contain the following public member functions 1) Power(); //Purpose: Create a Power object with default variable declarations. //THIS DEFINITION HAS BEEN PROVIDED 2) Power(string newname, int newdmg): /Purpose: Create a Power object which initializes the private member variables with the given //parameters. //THIS DEFINITION HAS BEEN PROVIDED 3) string get_name() const; //Purpose: Get the name of the Power 4) int get_damage const; //Purpose: Get the damage of the Power Fighter Class Specification: The Class Definition has already been provided, along with the headers for your member functions and four function definitions. The Fighter class will contain the following private member variables: 1) vector
power_vector. A vector of Power objects for the Fighter object. Default value is an empty vector of Power objects. 2) string name; A string name the Fighter object. Default value is ". 3) int speed; An integer speed for the Fighter object. Default value is -1. 4) int health; An integter health for the Fighter object. Default value is -1. 5) int attack; An integter health for the Fighter object. Default value is -1. The Fighter class will contain the following public member functions: 1) Fighter(); //Purpose: Create a Fighter object with default variable declarations //THIS DEFINITION HAS BEEN PROVIDED 8) 2) Fighter(string newname, int newattack, int newspeed, int newhealth, const vector& newpowers) /Purpose: Create a Figther object which initializes the private member variables with the given //parameters. //THIS DEFINITION HAS BEEN PROVIDED 3) int get_speed const: //Purpose: Get the speed of the Fighter 4) int get health const; //Purpose: Get the health of the Fighter 5) int get_attack() const; //Purpose: Get the attack of the Fighter 6) string get_name() const: // Purpose: Get the name of the Fighter 7) void set_speedint spd); //Purpose: Set the speed of the Fighter void set_health(int hp); //Purpose: Set the health of the Fighter 9) void set_attack(int atk); //Purpose: Set the attack of the Fighter 10) void set_name(string nm); //Purpose: Set the name of the Fighter 11) void take_damage(int newdamage); //Purpose: Subtract damage from health 12) bool is_conscious() const; //Purpose: Check if Fighter object has a positive health value. 1/Precondition: None //Postcondition: Return true if health is positive. Return false otherwise. 13) void print powers const; //THIS DEFINITION HAS BEEN PROVIDED 14) bool add_power(const Power& newpower): //THIS DEFINITION HAS BEEN PROVIDED 15) bool swap_power(int index, const Power& newpower); //Purpose: Change an existing power by index to the power_vector for a new power. /Preconditions: newpower is a Power object, index is an integer // Postconditions: If index is out of range of the power vector, return false. //Otherwise, replace the power at this index of the power vector with newpower and return true. 16) bool swap_power(const string& opname, const Power& newpower); 1 Purpose: Change an existing power by object type to the power_vector for a new power. 1/Preconditions: newpower is a power object, opname is a string object //Postconditions: If a power with a name equal to opname is not found at any index of the power vector, //return false. Otherwise, replace the power found at that index with newpower and return true. 17) bool swap_power(const Power& oldpower, const Power& newpower); 1/Purpose: Change an existing power by object type to the power_vector for a new power. //Preconditions: newpower is a Power object, oldpower is a power object //Postconditions: If a power with the same name as oldpower is not found at any index of the power vector, //return false. Otherwise, replace the power found at that index with newpower and return true. Assignment Specification: Power Class Specification: The Class Definition has already been provided, along with the headers for your member functions and a couple function defintions. The Power class will contain the following private member variables: 1) string name: A string name for the Power object. Default value should be 2) int damage An integer damage for the Power object. Default value should be - 1 The Power class will contain the following public member functions 1) Power(); //Purpose: Create a Power object with default variable declarations. //THIS DEFINITION HAS BEEN PROVIDED 2) Power(string newname, int newdmg): /Purpose: Create a Power object which initializes the private member variables with the given //parameters. //THIS DEFINITION HAS BEEN PROVIDED 3) string get_name() const; //Purpose: Get the name of the Power 4) int get_damage const; //Purpose: Get the damage of the Power Fighter Class Specification: The Class Definition has already been provided, along with the headers for your member functions and four function definitions. The Fighter class will contain the following private member variables: 1) vector power_vector. A vector of Power objects for the Fighter object. Default value is an empty vector of Power objects. 2) string name; A string name the Fighter object. Default value is ". 3) int speed; An integer speed for the Fighter object. Default value is -1. 4) int health; An integter health for the Fighter object. Default value is -1. 5) int attack; An integter health for the Fighter object. Default value is -1. The Fighter class will contain the following public member functions: 1) Fighter(); //Purpose: Create a Fighter object with default variable declarations //THIS DEFINITION HAS BEEN PROVIDED 8) 2) Fighter(string newname, int newattack, int newspeed, int newhealth, const vector& newpowers) /Purpose: Create a Figther object which initializes the private member variables with the given //parameters. //THIS DEFINITION HAS BEEN PROVIDED 3) int get_speed const: //Purpose: Get the speed of the Fighter 4) int get health const; //Purpose: Get the health of the Fighter 5) int get_attack() const; //Purpose: Get the attack of the Fighter 6) string get_name() const: // Purpose: Get the name of the Fighter 7) void set_speedint spd); //Purpose: Set the speed of the Fighter void set_health(int hp); //Purpose: Set the health of the Fighter 9) void set_attack(int atk); //Purpose: Set the attack of the Fighter 10) void set_name(string nm); //Purpose: Set the name of the Fighter 11) void take_damage(int newdamage); //Purpose: Subtract damage from health 12) bool is_conscious() const; //Purpose: Check if Fighter object has a positive health value. 1/Precondition: None //Postcondition: Return true if health is positive. Return false otherwise. 13) void print powers const; //THIS DEFINITION HAS BEEN PROVIDED 14) bool add_power(const Power& newpower): //THIS DEFINITION HAS BEEN PROVIDED 15) bool swap_power(int index, const Power& newpower); //Purpose: Change an existing power by index to the power_vector for a new power. /Preconditions: newpower is a Power object, index is an integer // Postconditions: If index is out of range of the power vector, return false. //Otherwise, replace the power at this index of the power vector with newpower and return true. 16) bool swap_power(const string& opname, const Power& newpower); 1 Purpose: Change an existing power by object type to the power_vector for a new power. 1/Preconditions: newpower is a power object, opname is a string object //Postconditions: If a power with a name equal to opname is not found at any index of the power vector, //return false. Otherwise, replace the power found at that index with newpower and return true. 17) bool swap_power(const Power& oldpower, const Power& newpower); 1/Purpose: Change an existing power by object type to the power_vector for a new power. //Preconditions: newpower is a Power object, oldpower is a power object //Postconditions: If a power with the same name as oldpower is not found at any index of the power vector, //return false. Otherwise, replace the power found at that index with newpower and return true