Question
Write a MATLAB program that allows numbers to be entered in decimal (base 10), hexadecimal (base 16), or binary (base 2), and then prints out
Write a MATLAB program that allows numbers to be entered in decimal (base 10), hexadecimal (base 16), or binary (base 2), and then prints out the number again in all three bases. To distinguish between different bases we'll say that hexadecimal numbers should always be preceded by a "$" and binary numbers by a "%". Other numbers are assumed to be decimal. When the program is run it should repeatedly allow a number to be entered and then print it out in all three bases until "q" is typed in. Here's an example of how your program should operate:
Enter #: 16
Decimal: 16
Hexadecimal: 10
Binary: 10000
Enter #: %1111
Decimal: 15
Hexadecimal: F
Binary: 1111
Enter #: $6D
Decimal: 109
Hexadecimal: 6D
Binary: 1101101
Enter #: q
Approach
A good way to structure this program is to read in each "number" as a string of characters, look at the first character to determine what kind of number it is (hex $, binary %, decimal ), and then call an appropriate function to convert the string into a hex, binary, or decimal number Then, print those numbers out next to each of their corresponding bases.
You will have to use these two functions and their variations to convert between bases: dec2bin(integer input); % e.g. 4 0100
hex2dec(string input); % e.g. 1D 14
Also, use one or more of the following functions to convert from a string to a number and vice versa: str2num(string input); num2str(numeric input);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
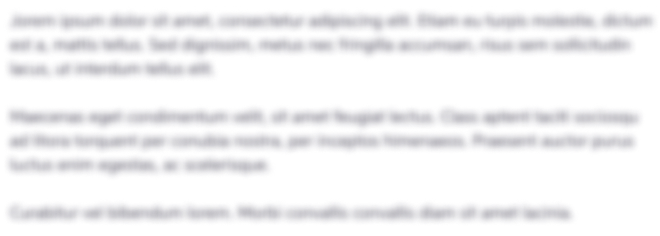
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started