Question
Write a (POSIX) program in C++ that searches for a specified file, and if found, prints out the relative path of the file from the
Write a (POSIX) program in C++ that searches for a specified file, and if found, prints out the relative path of the file from the current working directory and its time stamp. Your program should take one command line argument, the filename to find.
If your executable file is searchFile and you run this program from the root directory (/) and search for a file named test.txt. Your output might be something like the following: $./searchFile test.txt
Searching for test.txt
Found test.txt in /home/user/CIS315/
Last Edited: Jun 1 10:30
To start searching inside the current working directory you need to obtain a pointer to a DIR structure using the opendir() system call. The DIR structure provides you a method of getting a pointer to a dirent structure (directory entry) for each file or directory within the directory represented by DIR. You access each dirent structure by repeatedly calling the readdir() system call. Each time you call this with a given DIR * the function returns a pointer to a different dirent structure until there are no more within the directory, in which case readdir() return NULL. Within the dirent structure is information concerning its type (regular file or directory), its name, size, and timestamp information. For example if I wanted to print out the name of every file or directory within /home/user/, the following code would do the trick:
DIR * directory;
directory = opendir(/home/user);
struct dirent * dptr;
while ( dptr = readdir(directory)){
cout << dptr->d_name << endl;
}
Of course, you will need to do a recursive search, in which case you will need to be able to determine if a given dirent structure is a directory or a file. To do this, you need to use the stat() system call, which takes a stat structure as a parameter along with the name of the file and fills in the stat structure. To determine if the file is a directory, use the stat.st_mode field and a bit mask as shown below:
struct stat statStruct;
stat(dptr->d_name, &statStruct);
if( (statStruct.st_mode & S_IFMT) == S_IFDIR){
cout << This is a directory << endl;
}
When you are doing your recursive search through directories, do not traverse the . And .. entries. They are the current directory (.) and the parent directory (..). You do not want to go into those two directories. In order to print out the timestamp, you may use the following function:
void getTimestamp(struct dirent *dptr, char * dateString, int strLen){
struct tm *tm;
struct stat statStruct;
stat(dptr->d_name, &statStruct)
tm = localtime(&statStruct.st_mtime);
strftime(datestring, sizeof(datestring), "%d %b %R", tm);
}
To summary, you will need to research the following system calls and data structures in order to complete this project. These system calls and data structures are ONLY AVAILABLE in the UNIX/Linux environment. Do NOT use Windows
Step by Step Solution
There are 3 Steps involved in it
Step: 1
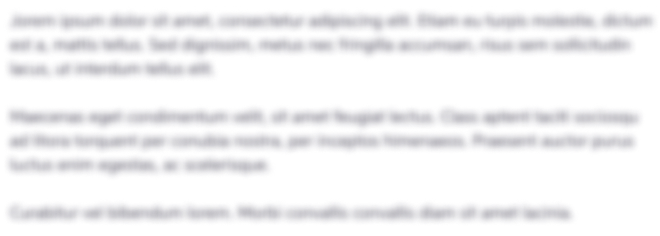
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started