Question
Write a program class with (2) subroutines and a main routine. The program needs to be a part of the Firstsubroutines class and I want
Write a program class with (2) subroutines and a main routine.
The program needs to be a part of the Firstsubroutines class and I want you to name your project Firstsubroutines if you are using Netbeans.
Your program needs to prompt the user to enter a string.
The program must then test the string entered by the user to determine whether it is a palindrome.
A palindrome is a string that reads the same backwards and forwards, such as "madam ", "racecar", and "able was I ere I saw elba".
It is customary to ignore spaces, punctuation, and capitalization when looking for palindromes.
For example, "A man, a plan, a canal. Panama!" is considered to be a palindrome.
To determine whether a string is a palindrome, you can: convert the string to lower case; remove any non-letter characters from the string; and compare the resulting string with the reverse of the same string. If they are equal, then the original string is considered to be a palindrome.
Heres the code for computing the reverse of str: String reverse; int i; reverse = ""; for (i = str.length() - 1; i >= 0; i--) { reverse = reverse + str.charAt(i); } As part of your assignment, you must write a static subroutine that finds the reverse of a string. The subroutine should have one parameter of type String and a return value of type String.
You must also write a second subroutine that takes a String as a parameter and returns a String. This subroutine should make a new string that is a copy of the parameter string, except that all the non-letters have been omitted. The new string should be returned as the value of the subroutine. You can tell that a character, ch is a lower-case letter by testing if (ch >= 'a' && ch <= 'z') (Note that for the operation of converting str to lower case, you can simply use the built-in toLowerCase subroutine by saying: str = str.toLowerCase();) You should write a descriptive comment before each subroutine, saying what it does.
Finally, write a main() routine that will read in a string from the user and determine whether or not it is a palindrome. The main routine should use The program should print the string converted to lower case and stripped of any non-letter characters.
Then it should print the reverse string.
Finally, it should say whether the string is a palindrome. (Use the two subroutines to process the user's string.) For example, if the user's input is "Hello World!", then the output might be: stripped: helloworld reversed: dlrowolleh This is NOT a palindrome. and if the input is "Campus motto: Bottoms up, Mac!", the output might be: stripped: campusmottobottomsupmac reversed: campusmottobottomsupmac This IS a palindrome.
You must complete your program, test, debug, and execute it. You should test two different cases, one that is a palindrome and another one that is not a palindrome.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
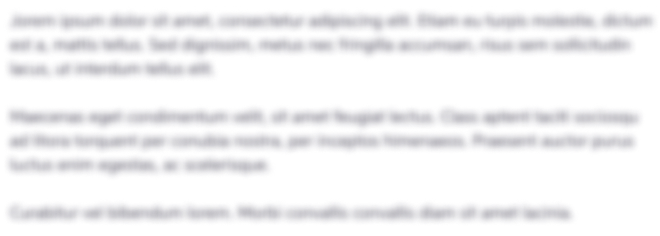
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started