Question
Write a program CustomerAccountArray that manages an array of CustomerAccount objects by performing these steps: Asks the user how many different CustomerAccounts to create Make
Write a program CustomerAccountArray that manages an array of CustomerAccount objects by performing these steps:
Asks the user how many different CustomerAccounts to create
Make a new array to hold the specified number of CustomerAccount objects
In a loop for the specified number of accounts, ask the user for the customer name, customer account number, and initial customer balance, construct a new CustomerAccount object with the data, and put the new CustomerAccount object in the array
After the user has entered all the customer account data, use a for loop (or a for each loop) to compute the sum of balances of all the customer accounts in the array.
Print the computed sum of the balances.
Use the provided CustomerAccount.java class for your CustomerAccount objects. Implement the main() method in the CustomerAccountArray.java class to do the five steps outlined above. Do not assume there will always be 3 customer accounts. If you do not use the exact class names (with the capital letters specified), the automatic testing program will not be able to run your program and points will be lost. Be sure to read the input values in exact order as shown so the automatic testing program can provide the input correctly to your program. Be sure to enter your name in the @author in the comment at the top of the CustomerAccountArray. Sample run (user input in bold font): Enter the number of customer accounts: 3
Enter customer name: Applied Ag
Enter customer account number: 1524
Enter customer initial balance: 1536.45
Enter customer name: Deer John
Enter customer account number: 1530
Enter customer initial balance: 607.11
Enter customer name: Avero
Enter customer account number: 1545
Enter customer initial balance: 1670.45
Total customer account balance is 3814.01
Please submit both the CustomerAccountArray.java file that you modify and the CustomerAccounts.java file so we can test your program.
You can use this as your outline for the main(String[] args) method in the CustomerAccountArray class:
Scanner in = new Scanner(System.in);
// Ask the user for the number of customer accounts.
// Create a CustomerAccount array to hold the specified
// number of customer account objects.
// Discard the remaining "newline" in Scanner's buffer.
in.nextLine();
// Loop to create the customer accounts:
for (...)
{
// Ask the user to enter the customer name,
// customer account number, and initial account balance.
// Construct a CustomerAccount object using the information
// provided by the user.
// Store each new CustomerAccount object into the array
// created above.
// Then, discard remaining "newline" in Scanner's buffer.
in.nextLine();
}
// Loop: Compute the sum of the account balances.
// Print the sum of the account balances.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
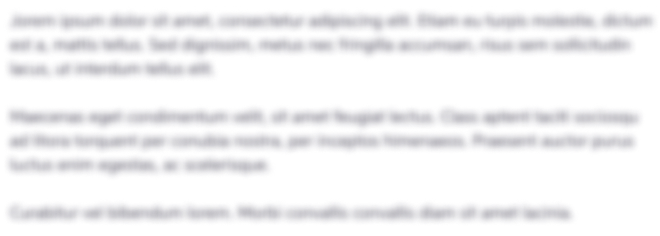
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started