Question
Write a program for a phone directory by reusing the cStrTools module of part one and adding a Phone module and a directory main module.
Write a program for a phone directory by reusing the cStrTools module of part one and adding a Phone module and a directory main module.
The cStrTools Module is here:
cStrTools.cpp:
#include namespace sdds { // returns the lower case value of a character char toLower(char ch) { if (ch >= 'A' && ch <= 'Z') ch += ('a' - 'A'); return ch; } // compares s1 and s2 cStrings and returns: // > 0 if s1 > s2 // < 0 if s1 < s3 // == 0 if s1 == s2 int strCmp(const char* s1, const char* s2) { int i; for (i = 0; s1[i] && s2[i] && s1[i] == s2[i]; i++); return s1[i] - s2[i]; } // compares s1 and s2 cStrings upto len characters and returns: // > 0 if s1 > s2 // < 0 if s1 < s3 // == 0 if s1 == s2 int strnCmp(const char* s1, const char* s2, int len) { int i = 0; while (i < len - 1 && s1[i] && s2[i] && s1[i] == s2[i]) { i++; } return s1[i] - s2[i]; } // copies src to des void strCpy(char* des, const char* src) { int i; for (i = 0; src[i]; i++) des[i] = src[i]; des[i] = 0; } // returns the length of str int strLen(const char* str) { int len; for (len = 0; str[len]; len++); return len; } // if "find" is found in "str" it will return the addres of the match // if not found it will returns nullptr (zero) const char* strStr(const char* str, const char* find) { const char* faddress = nullptr; int i, flen = strLen(find), slen = strLen(str); for (i = 0; i <= slen - flen && strnCmp(&str[i], find, flen); i++); if (i <= slen - flen) faddress = &str[i]; return faddress; } // returns true if ch is alphabetical int isAlpha(char ch) { return (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z'); } // returns true if ch is a whitespace character int isSpace(char ch) { return ch == ' ' || ch == '\t' || ch == ' ' || ch == '\v' || ch == '\f' || ch == ' '; } // removes the non-alphabetic characters from the begining and end of a word void trim(char word[]) { int i; while (word[0] && !isAlpha(word[0])) { strCpy(word, word + 1); } i = strLen(word); while (i && !isAlpha(word[i - 1])) { word[i-- - 1] = 0; } } // copies the lower case version of the source into des. void toLowerCaseAndCopy(char des[], const char source[]) { int i = 0, j = 0; for (; source[i] != 0; i++) { des[j++] = toLower(source[i]); } des[j] = 0; } }
and cStrTools.h:
#ifndef NAMESPACE_cStrTools_H // replace with relevant names #define NAMESPACE_cStrTools_H namespace sdds { char toLower(char ch); int strCmp(const char* s1, const char* s2);
int strnCmp(const char* s1, const char* s2, int len); void strCpy(char* des, const char* src); int strLen(const char* str); const char* strStr(const char* str, const char* find); int isAlpha(char ch); int isSpace(char ch); void trim(char word[]); void toLowerCaseAndCopy(char des[], const char source[]); } #endif
This program should be set up using a title for the phone directory and a file name for a data file. (see the directory module)
the data file
The data file holds records of a phone number in a tab separated format as follows:
[Name]\t[areaCode]\t[prefix]\t[number]
For example the following information:
Fred Soley (416)555-0123
is kept in the file as follows:
Fred Soley\t416\t491\t0123
Hint: to read the above record use the follwing fscanf format fscanf(fptr, "%[^\t]\t%s\t%s\t%s ",name,area,prefix,number)
view The data file
the maximum length for a name is 50 characters.
Execution
- When running, after showing the title, the program should prompt for a partial name entry.
- After receiving the partial name the program should search through the names in the file and if a name is found containing the partial entry, the matching phone recored is displayed.
- Nothing is displayed if no match is found.
- If the user enters '!' the program exits.
- If the data file could not be opened the program exits displaying an error message
- A thank you message is displayed at the end of the program.
For output formatting and messages, see the execution samples
Phone module
Only one mandatory function is required for the Phone module:
// runs the phone directory appication void phoneDir(const char* programTitle, const char* fileName);
You are free to create any function you find necessary to accomplish this task.
Tester Program
#include "Phone.h" using namespace sdds; int main() { phoneDir("Star Wars", "phones.txt"); return 0; }
DIY Execution example (existing data file)
Star Wars phone direcotry search ------------------------------------------------------- Enter a partial name to search (no spaces) or enter '!' to exit > lukE Luke Skywalker: (301) 555-0630 Enter a partial name to search (no spaces) or enter '!' to exit > sky Luke Skywalker: (301) 555-0630 Enter a partial name to search (no spaces) or enter '!' to exit > fett Jango Fett: (905) 555-6016 Boba Fett: (905) 555-9382 Enter a partial name to search (no spaces) or enter '!' to exit > feT Jango Fett: (905) 555-6016 Boba Fett: (905) 555-9382 Enter a partial name to search (no spaces) or enter '!' to exit > Jack Enter a partial name to search (no spaces) or enter '!' to exit > ! Thank you for using Star Wars directory.
DIY unsuccessful execution
#include "Phone.h" using namespace sdds; int main() { phoneDir("Star Wars", "badfile.txt"); return 0; }
DIY unsuccessful Execution example (data file not found)
Star Wars phone direcotry search ------------------------------------------------------- badfile.txt file not found! Thank you for using Star Wars directory. D:\Users\phard\Documents\Seneca\oop244\DEV\Workshops\WS01\DIY\Debug\DIY.exe (process 8424) exited with code 0. Press any key to close this window . . .
Step by Step Solution
There are 3 Steps involved in it
Step: 1
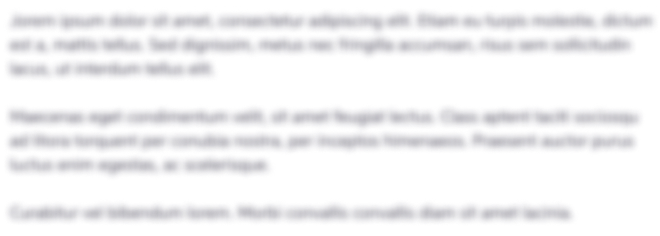
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started