Question
Write a program in C base code #include #include typedef struct mazeStruct { char arr[32][32]; /* allows for a maze of size 30x30 plus outer
Write a program in C
base code
#include
typedef struct mazeStruct { char arr[32][32]; /* allows for a maze of size 30x30 plus outer walls */ int xsize, ysize; int xstart, ystart; int xend, yend; } maze;
int main (int argc, char **argv) { maze m1;
int xpos, ypos; int i,j;
FILE *src;
/* verify the proper number of command line arguments were given */ if(argc != 2) { printf("Usage: %s ", argv[0]); exit(-1); } /* Try to open the input file. */ if ( ( src = fopen( argv[1], "r" )) == NULL ) { printf ( "Can't open input file: %s", argv[1] ); exit(-1); }
/* read in the size, starting and ending positions in the maze */ fscanf (src, "%d %d", &m1.xsize, &m1.ysize); fscanf (src, "%d %d", &m1.xstart, &m1.ystart); fscanf (src, "%d %d", &m1.xend, &m1.yend); /* print them out to verify the input */ printf ("size: %d, %d ", m1.xsize, m1.ysize); printf ("start: %d, %d ", m1.xstart, m1.ystart); printf ("end: %d, %d ", m1.xend, m1.yend);
/* initialize the maze to empty */ for (i = 0; i
/* mark the borders of the maze with *'s */ for (i=0; i
/* mark the starting and ending positions in the maze */ m1.arr[m1.xstart][m1.ystart] = 's'; m1.arr[m1.xend][m1.yend] = 'e'; /* mark the blocked positions in the maze with *'s */ while (fscanf (src, "%d %d", &xpos, &ypos) != EOF) { m1.arr[xpos][ypos] = '*'; }
/* print out the initial maze */ for (i = 0; i
}
You MUST write functions for the following operations (these are the "same" functions as Project 2) initializing the stack, checking if the stack is empty, pushing an element onto the stack, popping an element off of the stack, accessing the top element on the stack, and . . . . resetting the stack so that it is empty and ready to be used again. These functions must NOT contain memory leaks! Expect points deducted if your code fails in this regard. (Valgrind will be used when grading.) Command Line Argument: Debug Mode Your program must be able to take one optional command line argument, the -d flag. When this flag is given, your program is to run in "debug" mode. When in this mode, your program is to display the coordinates of the maze positions as they are pushed onto the stack and popped off the stack if the Top of Stack coordinate does not have an unvisited (and unblocked) neighbor. When the flag is not given, this debugging information should not be displayed. Since the input file for the maze also comes from the command line arguments, you may not assume which order in which the command line arguments are given. Thus the command line arguments may be given as . ./a.out mazeInput.txt . ./a.out mazeInput.txt -d . ./a.out -d mazeInput.txt One simple way to set up a "debugging" mode is to use a boolean variable which is set to true when debugging mode is turned on but false otherwise. Then using a simple if statement controls whether information should be output or not. if ( debugMode TRUE) printf(" Debugging Information In"); Optional ways for writing this code can be seen on the course website You MUST write functions for the following operations (these are the "same" functions as Project 2) initializing the stack, checking if the stack is empty, pushing an element onto the stack, popping an element off of the stack, accessing the top element on the stack, and . . . . resetting the stack so that it is empty and ready to be used again. These functions must NOT contain memory leaks! Expect points deducted if your code fails in this regard. (Valgrind will be used when grading.) Command Line Argument: Debug Mode Your program must be able to take one optional command line argument, the -d flag. When this flag is given, your program is to run in "debug" mode. When in this mode, your program is to display the coordinates of the maze positions as they are pushed onto the stack and popped off the stack if the Top of Stack coordinate does not have an unvisited (and unblocked) neighbor. When the flag is not given, this debugging information should not be displayed. Since the input file for the maze also comes from the command line arguments, you may not assume which order in which the command line arguments are given. Thus the command line arguments may be given as . ./a.out mazeInput.txt . ./a.out mazeInput.txt -d . ./a.out -d mazeInput.txt One simple way to set up a "debugging" mode is to use a boolean variable which is set to true when debugging mode is turned on but false otherwise. Then using a simple if statement controls whether information should be output or not. if ( debugMode TRUE) printf(" Debugging Information In"); Optional ways for writing this code can be seen on the course website
Step by Step Solution
There are 3 Steps involved in it
Step: 1
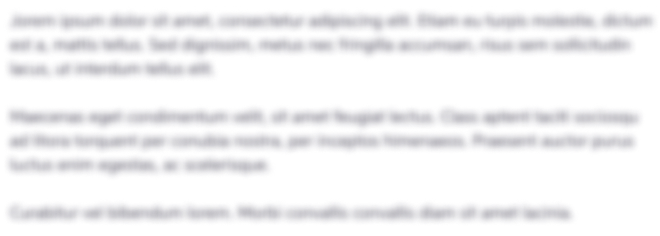
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started